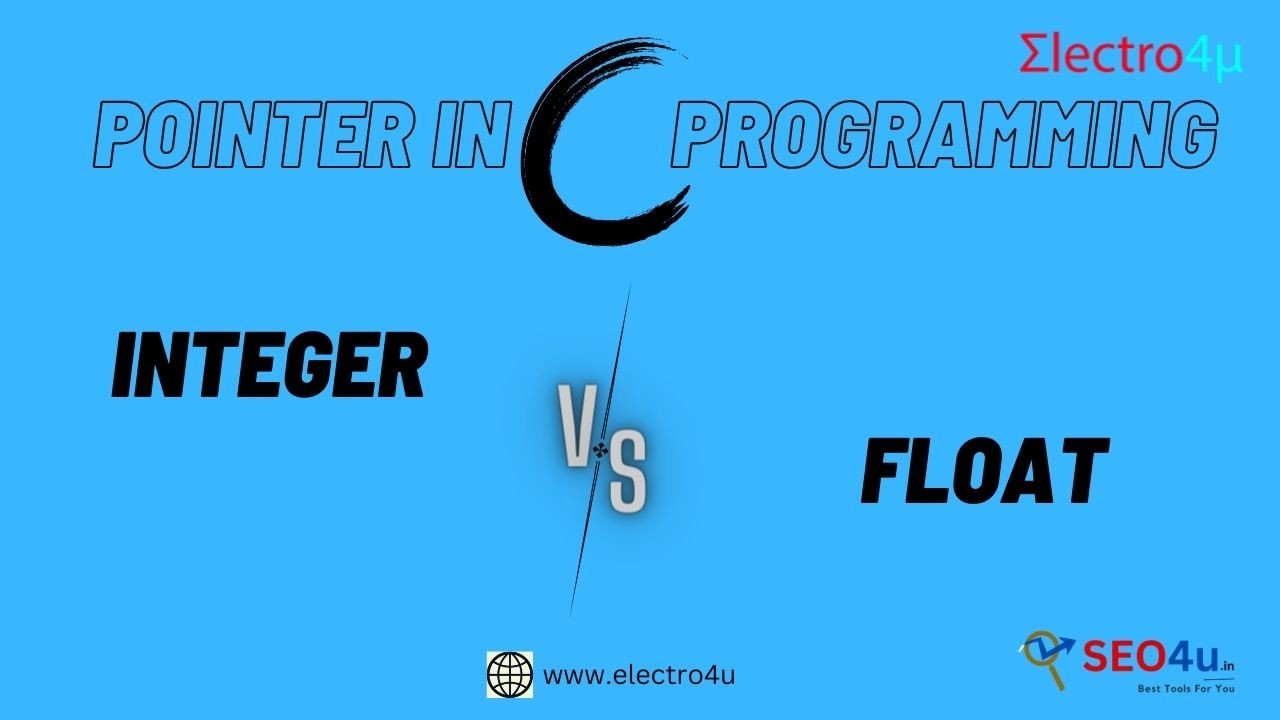
Difference Between integer pointer and float pointer in c
Integer pointer in c
In C programming, an integer pointer and a float pointer are both pointers that are used to store memory addresses of variables, but they differ in the data type of the variable they point to.
The main difference between an integer pointer and a float pointer in C is the data type of the variable they point to. An integer pointer points to a variable of integer type, while a float pointer points to a variable of float type.
Difference in data type has a number of implications, including:
- The size of the pointer: The size of a pointer in C is determined by the size of the data type it points to. Integer pointers are typically 4 bytes in size, while float pointers are typically 8 bytes in size.
- The operations that can be performed on the pointer: The operations that can be performed on a pointer are also determined by the data type it points to. For example, you can add or subtract integers from an integer pointer, but you cannot add or subtract floats from a float pointer.
- The way that the pointer is dereferenced: To access the value of the variable that a pointer points to, you need to dereference the pointer. This is done using the asterisk (*) operator. However, the way that a pointer is dereferenced depends on the data type of the variable it points to. For example, to dereference an integer pointer, you simply use the asterisk operator. However, to dereference a float pointer, you need to cast the pointer to a float type before dereferencing it.
Table summarizes the key differences between integer pointers and float pointers:
Characteristic | Integer pointer | Float pointer |
---|---|---|
Data type pointed to | Integer | Float |
Size | 4 bytes | 8 bytes |
Operations allowed | Addition, subtraction, comparison, casting | Casting, dereferencing |
Dereferencing | Use the asterisk (*) operator | Cast the pointer to a float type before dereferencing |
Example of how to use integer and float pointers:
// Declare an integer pointer.
int* int_pointer;
// Declare a float pointer.
float* float_pointer;
// Allocate memory for an integer variable.
int* int_variable = malloc(sizeof(int));
// Allocate memory for a float variable.
float* float_variable = malloc(sizeof(float));
// Assign the address of the integer variable to the integer pointer.
int_pointer = int_variable;
// Assign the address of the float variable to the float pointer.
float_pointer = float_variable;
// Store a value in the integer variable.
*int_pointer = 10;
// Store a value in the float variable.
*float_pointer = 10.5;
// Print the value of the integer variable.
printf("%d\n", *int_pointer);
// Print the value of the float variable.
printf("%f\n", *float_pointer);
Output:
10
10.5
As you can see, the integer pointer and the float pointer are used in the same way to access the values of the variables they point to. However, it is important to be aware of the differences between integer pointers and float pointers, such as the size of the pointer and the way that it is dereferenced.
The size of a pointer is not fixed, it depends on Word size of the processor. In general a 32-bit computer machine the size of a pointer would be 4 bytes while for a 64-bit computer machine, it would be 8 bytes.