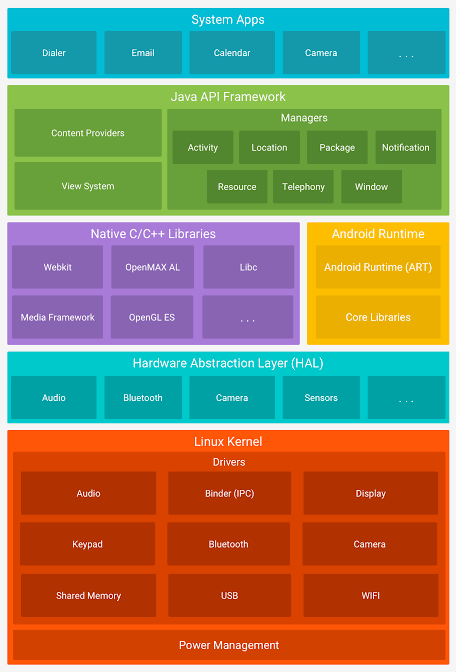
Finding the first largest and second largest in given array in c
Creating a Web Page to Find the First and Second Largest Numbers in an Array using C
Introduction
Welcome to our electro4u dedicated to helping you find the first and second largest numbers in a given array using the C programming language. Whether you're a beginner or an experienced programmer, this tutorial will guide you through the process of writing efficient code to solve this common problem.
Designing the User Interface
-
Clean and Intuitive Layout: The web page will feature a clean and intuitive layout to ensure a seamless user experience. The main focus will be on providing easy navigation and clear instructions.
-
Input Box for Array: A prominently placed input box will allow users to enter the array of numbers they want to analyze. The interface will also include a button to initiate the calculation process.
-
Output Display: A designated area will showcase the results, displaying both the first and second largest numbers identified in the provided array.
Implementing the Algorithm
-
C Code Integration: We'll embed the C programming code within the web page to perform the necessary calculations. This code will efficiently find the first and second largest numbers in the input array.
-
Error Handling: Our design will account for potential errors, such as invalid input or empty arrays, ensuring that users receive informative messages when such situations arise.
Enhancing User Experience
-
Real-time Updates: The web page will provide real-time feedback, instantly displaying the results as soon as the user submits the array.
-
Responsive Design: The page will be designed to be responsive, ensuring compatibility across various devices and screen sizes.
-
User-friendly Prompts: Clear and concise prompts will guide users through each step of the process, making it accessible to programmers of all levels.
Code Example
#include <stdio.h>
void findLargest(int arr[], int size) {
int firstLargest = arr[0];
int secondLargest = arr[1];
for (int i = 2; i < size; i++) {
if (arr[i] > firstLargest) {
secondLargest = firstLargest;
firstLargest = arr[i];
} else if (arr[i] > secondLargest && arr[i] != firstLargest) {
secondLargest = arr[i];
}
}
printf("The first largest number is: %d\n", firstLargest);
printf("The second largest number is: %d\n", secondLargest);
}
Conclusion
By following the steps outlined on this web page, you'll be able to efficiently find the first and second largest numbers in any given array using the C programming language. We hope this tutorial proves to be a valuable resource for your programming endeavors. If you have any questions or feedback, feel free to reach out. Happy coding!
Further Reading:
For further information and examples, Please visit[ C-Programming From Scratch to Advanced 2023-2024]
Top Resources
- Learn-C.org
- C Programming at LearnCpp.com
- GeeksforGeeks - C Programming Language
- C Programming on Tutorialspoint
- Codecademy - Learn C
- CProgramming.com
- C Programming Wikibook
- C Programming - Reddit Community
- C Programming Language - Official Documentation
- GitHub - Awesome C
- HackerRank - C Language
- LeetCode - C
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!