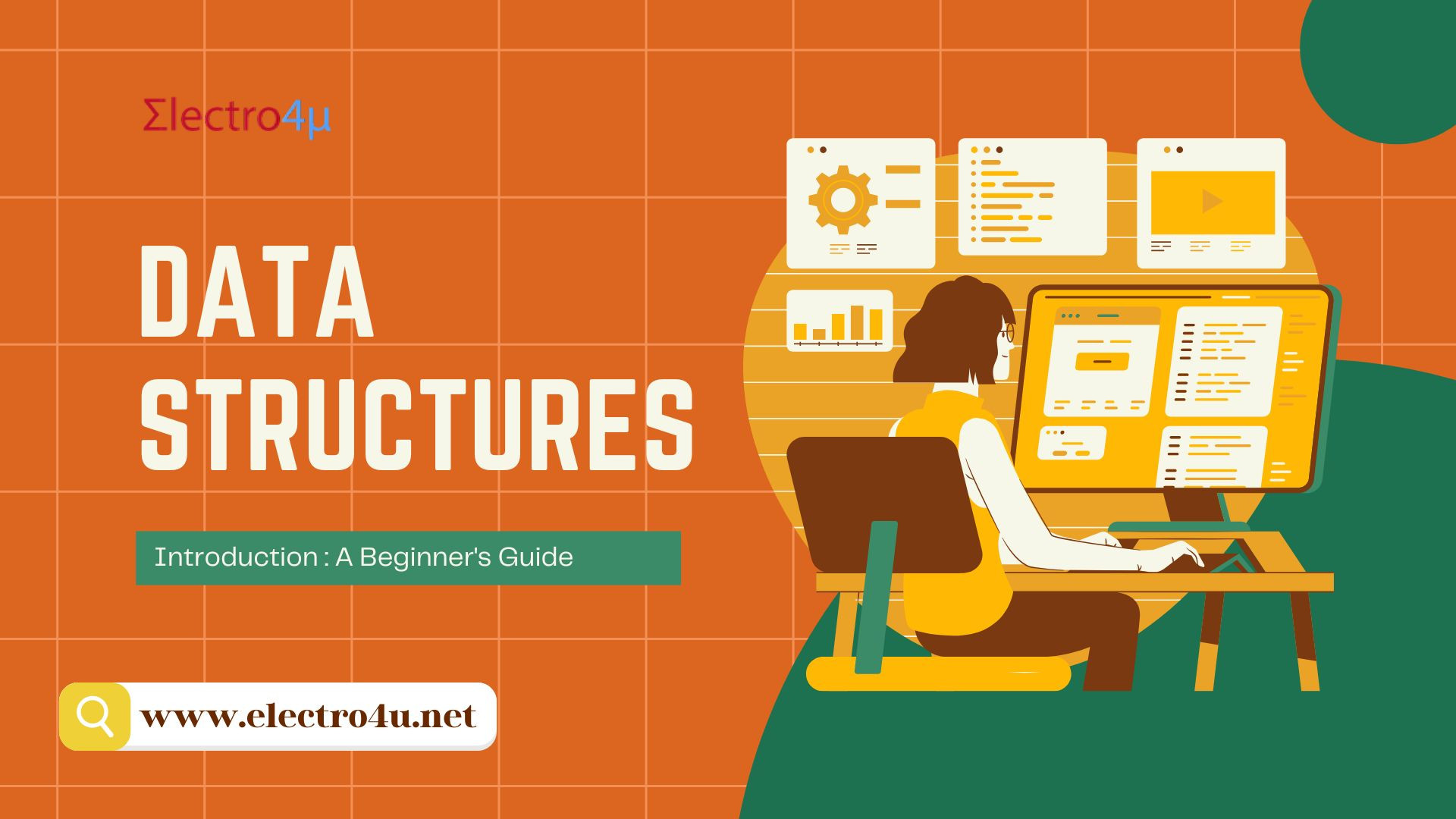
Introduction to Data Structures in C: A Beginner's Guide
Introduction to Data Structures in C: A Beginner's Guide
If you're new to programming, you might have heard the term "data structures" floating around. It sounds like something complicated, but it's actually quite simple once you break it down. In this blog, we’ll take a closer look at what data structures are, why they’re important, and how they work in C programming.
What are Data Structures?
In simple terms, data structures are just ways of organizing and storing data so that we can easily find, modify, or manage it. Imagine a library instead of throwing all the books in one big pile, you organize them by genre, author, or title. Similarly, data structures help programmers organize data in a way that makes it easier to work with.
Why Should You Care About Data Structures?
Good data structures can make your code:
>>Faster: Perform tasks like searching, sorting, or adding data more quickly.
>>Smarter: Handle large amounts of data with ease.
>>Neater: Keep your code organized and less prone to errors.
Types of Data Structures in C
In C, data structures are broadly divided into two categories:
1. Primitive Data Structures: These are the basic building blocks, like numbers or characters.
- int: Integer values.
- float: Decimal numbers.
- char: Single characters.
- double: Larger decimal numbers.
2. Non-Primitive Data Structures: These are more complex and are built using the basic data types. They help you store and manipulate data more efficiently.
- Linear Data Structures: Data is stored in a sequential order.
- Non-Linear Data Structures: Data is stored in a hierarchical or network-based structure.
Let’s dive deeper into the Linear Data Structures first!
Linear Data Structures: A Closer Look
1. Arrays: An array is like a row of boxes where each box can hold a piece of data. They’re simple, fast, and easy to use.
int arr[5] = {1, 2, 3, 4, 5};
- Pros: Easy to access and use.
- Cons: Fixed size, meaning once you define the size, you can’t change it.
2. Linked Lists: A linked list is like a chain of nodes. Each node holds data and points to the next node. It’s flexible because it can grow or shrink in size as needed.
struct Node
{
int data;
struct Node* next;
};
- Pros: You can add or remove elements easily.
- Cons: Slower access to data compared to arrays.
3. Stacks: A stack works on a Last In, First Out (LIFO) principle. Imagine a stack of plates, you can only add or remove the top plate.
#define MAX 10
int stack[MAX];
int top = -1;
- Operations: push() to add a plate, pop() to remove one.
- Real-life Example: Undo function in software.
4. Queues: A queue is like a line at a ticket counter, following a First In, First Out (FIFO) system.
#define MAX 10
int queue[MAX];
int front = -1, rear = -1;
- Operations: enqueue() to add a person, dequeue() to serve the first person in line.
- Real-life Example: People waiting in line.
Non-Linear Data Structures: Moving Beyond the Basics
1. Trees: A tree structure looks like a family tree. Each node has a value, and it can have many children, but only one parent. A binary tree is a type of tree where each node has at most two children.
struct Node
{
int data;
struct Node* left;
struct Node* right;
};
Applications: Used for hierarchical data like file systems.
2. Graphs: A graph consists of vertices (nodes) and edges (connections between nodes). It’s a great way to represent networks, like social media connections or transportation routes.
struct Graph
{
int V;
int** adjMatrix;
};
Applications: Social networks, routing algorithms.
Choosing the Right Data Structure
- When working with data structures, choosing the right one for the job is crucial:
- Use Arrays when you need fast access to elements and have a fixed size.
- Use Linked Lists when you need dynamic memory management and frequent insertions/deletions.
- Use Stacks/Queues for tasks that follow LIFO or FIFO principles.
- Use Trees/Graphs for hierarchical or network-based data.
Conclusion
Data structures are the backbone of computer programming. Once you understand how to use them, you’ll be able to write more efficient, organized, and powerful code. Whether you're managing simple lists or complex networks, understanding data structures in C will help you tackle a wide range of problems.
So, next time you find yourself dealing with data, remember: just like organizing a messy room, choosing the right data structure will make your job much easier!
Stay Tuned for More!
This blog is just an introduction to the world of data structures in C. I’m currently working on detailed blogs for each of the topics mentioned here, where I will dive deeper into their concepts, implementation, and real-world applications. So, stay tuned for more blogs that will guide you step by step in mastering data structures in C!
Happy Coding!
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!