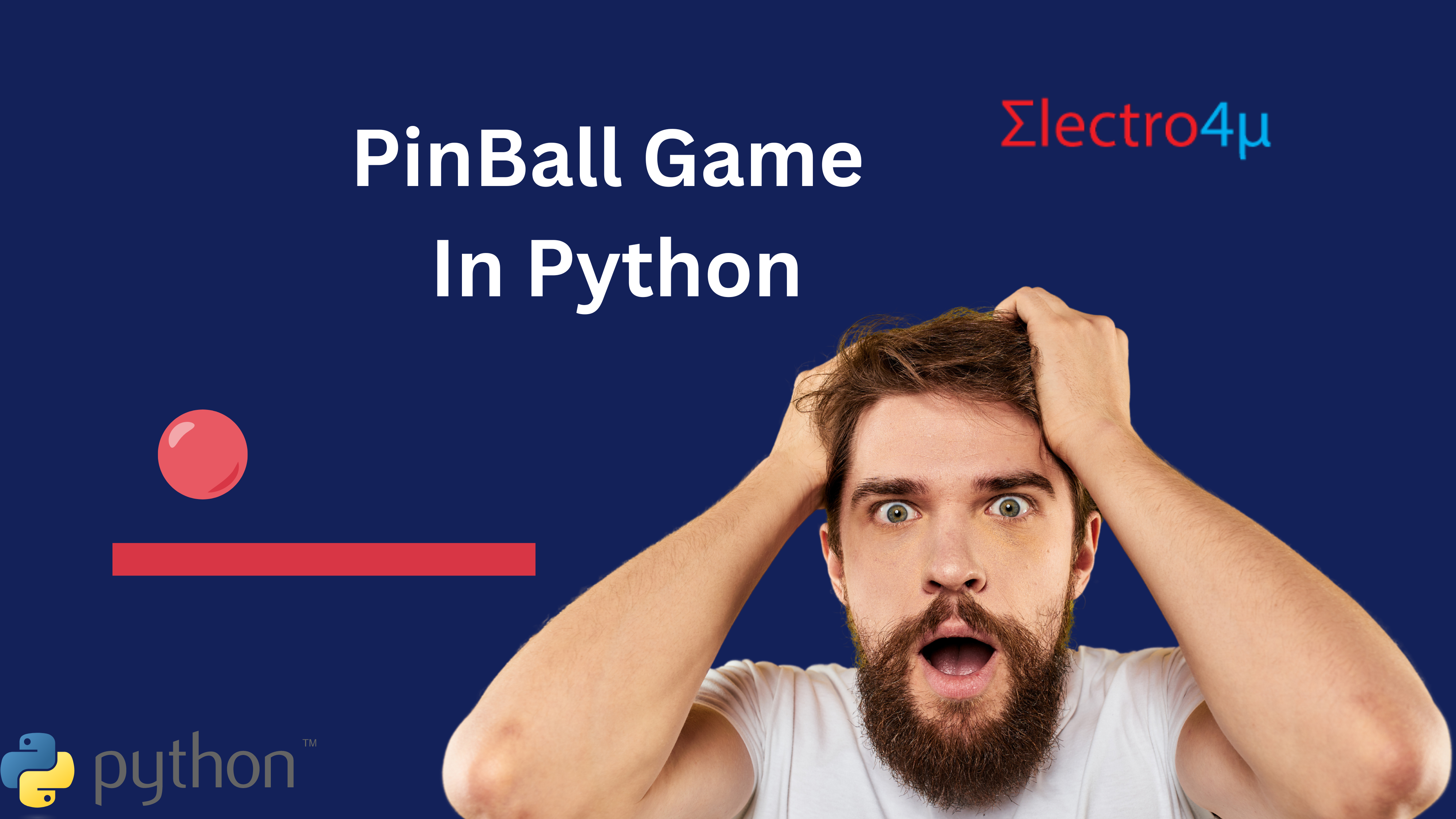
Create Your Own Pinball Game in Python with Source Code
Create a Fun and Exciting Pinball Game with Python
Are you looking to add a little bit of fun and excitement to your next programming project? Look no further than creating a pinball game with Python! With only a few lines of code, you can create an incredibly immersive and highly-interactive version of this classic arcade game. Plus, it’s totally open source, so you can share it with the world! To get started, check out our tutorial on how to build a Python pinball game.
What You Will Need
To create a pinball game with Python, you will need the following tools:
- Python 3
- import turtle
- import os
Step by Step Instructions
Let’s dive right in! Here’s a step-by-step walkthrough of what you will need to do to create a Python pinball game.
- Download and install Python 3
- Download and install the Pygame library
- Create the main game loop. This is the code that will be executed each time the game runs.
- Create the pinball game window. This is the code that will create the window that the game is played on.
- Create the pinball game objects. This code will define the flippers, bumpers, targets, rails, walls, etc.
- Create the collision detection. This code will detect when the ball hits an object and determine the resulting action.
- Create the game score. This code will keep track of the player’s points throughout the game.
- Create the game sound effects. This code will generate the sounds associated with various game events.
- Test the game. Once all of the code is written, you should thoroughly test it to make sure it works as expected.
- Share your game. Once the game is complete, you can share it with the world!
Source Code
Below is the complete source code for creating a Python pinball game. Simply copy and paste this code into a text editor and save it as "pinball.py" and then run it using Python 3. If you have any questions about this code, feel free to contact us.
# Simple Pong in Python 3 for Beginners
# By @Electrto4u
import turtle
import os
wn = turtle.Screen()
wn.title("Pong")
wn.bgcolor("black")
wn.setup(width=800, height=600)
wn.tracer(0)
# Score
score_a = 0
score_b = 0
# Paddle A
paddle_a = turtle.Turtle()
paddle_a.speed(10)
paddle_a.shape("square")
paddle_a.color("white")
paddle_a.shapesize(stretch_wid=5,stretch_len=1)
paddle_a.penup()
paddle_a.goto(-350, 0)
# Paddle B
paddle_b = turtle.Turtle()
paddle_b.speed(0)
paddle_b.shape("square")
paddle_b.color("white")
paddle_b.shapesize(stretch_wid=5,stretch_len=1)
paddle_b.penup()
paddle_b.goto(350, 0)
# Ball
ball = turtle.Turtle()
ball.speed(0)
ball.shape("square")
ball.color("white")
ball.penup()
ball.goto(0, 0)
ball.dx = 0.10
ball.dy = 0.10
# Pen
pen = turtle.Turtle()
pen.speed(0)
pen.shape("square")
pen.color("white")
pen.penup()
pen.hideturtle()
pen.goto(0, 260)
pen.write("Balmiki A: 0 sejal B: 0", align="center", font=("Courier", 24, "normal"))
# Functions
def paddle_a_up():
y = paddle_a.ycor()
y += 20
paddle_a.sety(y)
def paddle_a_down():
y = paddle_a.ycor()
y -= 20
paddle_a.sety(y)
def paddle_b_up():
y = paddle_b.ycor()
y += 20
paddle_b.sety(y)
def paddle_b_down():
y = paddle_b.ycor()
y -= 20
paddle_b.sety(y)
# Keyboard bindings
wn.listen()
wn.onkeypress(paddle_a_up, "w")
wn.onkeypress(paddle_a_down, "s")
wn.onkeypress(paddle_b_up, "Up")
wn.onkeypress(paddle_b_down, "Down")
# Main game loop
while True:
wn.update()
# Move the ball
ball.setx(ball.xcor() + ball.dx)
ball.sety(ball.ycor() + ball.dy)
# Border checking
# Top and bottom
if ball.ycor() > 290:
ball.sety(290)
ball.dy *= -1
os.system("afplay bounce.wav&")
elif ball.ycor() < -290:
ball.sety(-290)
ball.dy *= -1
os.system("afplay bounce.wav&")
# Left and right
if ball.xcor() > 350:
score_a += 1
pen.clear()
pen.write("Player A: {} Player B: {}".format(score_a, score_b), align="center", font=("Courier", 24, "normal"))
ball.goto(0, 0)
ball.dx *= -1
elif ball.xcor() < -350:
score_b += 1
pen.clear()
pen.write("Player A: {} Player B: {}".format(score_a, score_b), align="center", font=("Courier", 24, "normal"))
ball.goto(0, 0)
ball.dx *= -1
# Paddle and ball collisions
if ball.xcor() < -340 and ball.ycor() < paddle_a.ycor() + 50 and ball.ycor() > paddle_a.ycor() - 50:
ball.dx *= -1
os.system("afplay bounce.wav&")
elif ball.xcor() > 340 and ball.ycor() < paddle_b.ycor() + 50 and ball.ycor() > paddle_b.ycor() - 50:
ball.dx *= -1
os.system("afplay bounce.wav&")
Conclusion:
This code is for a simple Pong game in Python. It uses the Turtle graphics library to create the game.
The code first imports the turtle and os modules. The turtle module is used to create the game graphics, and the os module is used to play a sound effect when the ball hits the paddles.
The next few lines of code set up the game screen and the score. The screen is 800 pixels wide and 600 pixels high, and the background is black. The score is initialized to 0 for both players.
The next few lines of code create the paddles and the ball. The paddles are 50 pixels wide and 10 pixels high, and they are positioned at the left and right edges of the screen. The ball is 20 pixels wide and 20 pixels high, and it is positioned in the center of the screen.
The next few lines of code create the pen that will be used to display the score. The pen is 20 pixels wide and 20 pixels high, and it is positioned at the top of the screen.
The next few lines of code define the functions that will be used to move the paddles. The paddle_a_up() and paddle_a_down() functions are used to move the left paddle up and down, respectively. The paddle_b_up() and paddle_b_down() functions are used to move the right paddle up and down, respectively.
The next few lines of code set up the keyboard bindings. The wn.listen() function tells the turtle library to listen for keyboard events. The wn.onkeypress() function tells the turtle library what to do when a certain key is pressed. In this case, the w key is bound to the paddle_a_up() function, the s key is bound to the paddle_a_down() function, the Up key is bound to the paddle_b_up() function, and the Down key is bound to the paddle_b_down() function.
The last few lines of code are the main game loop. This loop will run forever, or until the user quits the game. In each iteration of the loop, the following steps are taken:
- The screen is updated.
- The ball is moved.
- The border checking is performed.
- The paddle and ball collisions are checked.
- The score is updated.
If the ball hits the left or right edge of the screen, the score for the opposite player is increased. If the ball hits one of the paddles, the direction of the ball is reversed.