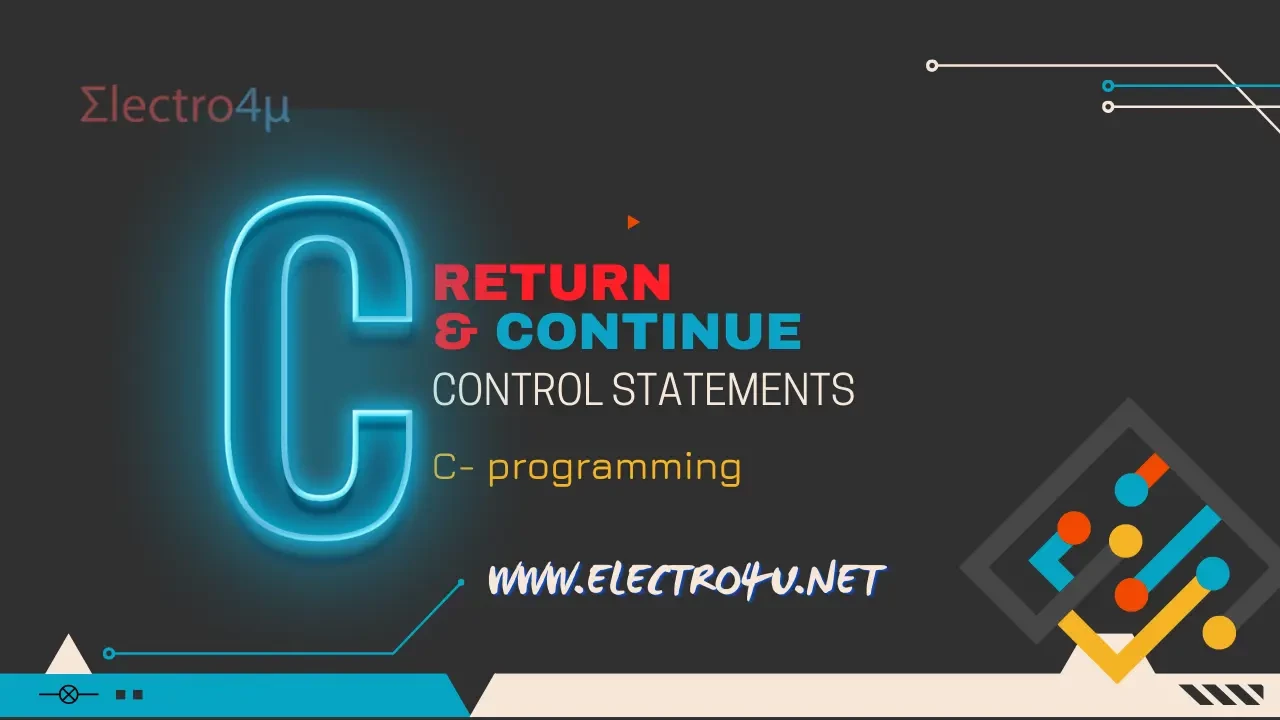
Return and Continue in c programming
Understanding Return and Continue Statements in C Programming
In C programming, "return" and "continue" are keywords that serve different purposes:
1. Return: The "return" keyword is used within a function to exit the function and return a value back to the caller. It allows you to provide a result or outcome of a function's execution. When a "return" statement is encountered in a function, it immediately terminates the function's execution and returns control to the calling code.
>Return belongs to unconditional non-iterative control statements, return is used to come out from the function
There are two types of return
- control return
Example: return (n) return control
- value return
return sum (value )
Here's an example of a function that calculates the square of a number and uses the "return" statement to provide the result:
#include
int square(int num) {
int result = num * num;
return result; // Return the square of the number
}
int main() {
int number = 5;
int squaredNumber = square(number);
printf("The square of %d is %d\n", number, squaredNumber);
return 0;
}
In this example, the "return" statement is used in the "square" function to return the square of the given number back to the caller.
2. Continue: The "continue" keyword is used within loop structures (such as "for", "while", or "do-while") to skip the remaining code within the loop for the current iteration and move on to the next iteration. It is typically used to bypass certain parts of a loop's logic based on certain conditions.
Here's an example that demonstrates the usage of the "continue" statement in a "for" loop to skip even numbers:
#include
int main() {
for (int i = 1; i <= 10; i++) {
if (i % 2 == 0) {
continue; // Skip even numbers
}
printf("%d ", i);
}
return 0;
}
In this example, the "continue" statement is used to skip the even numbers in the loop, so only the odd numbers are printed.
Both "return" and "continue" are important control flow constructs in C programming, providing the means to exit functions or skip specific iterations in loops, respectively.