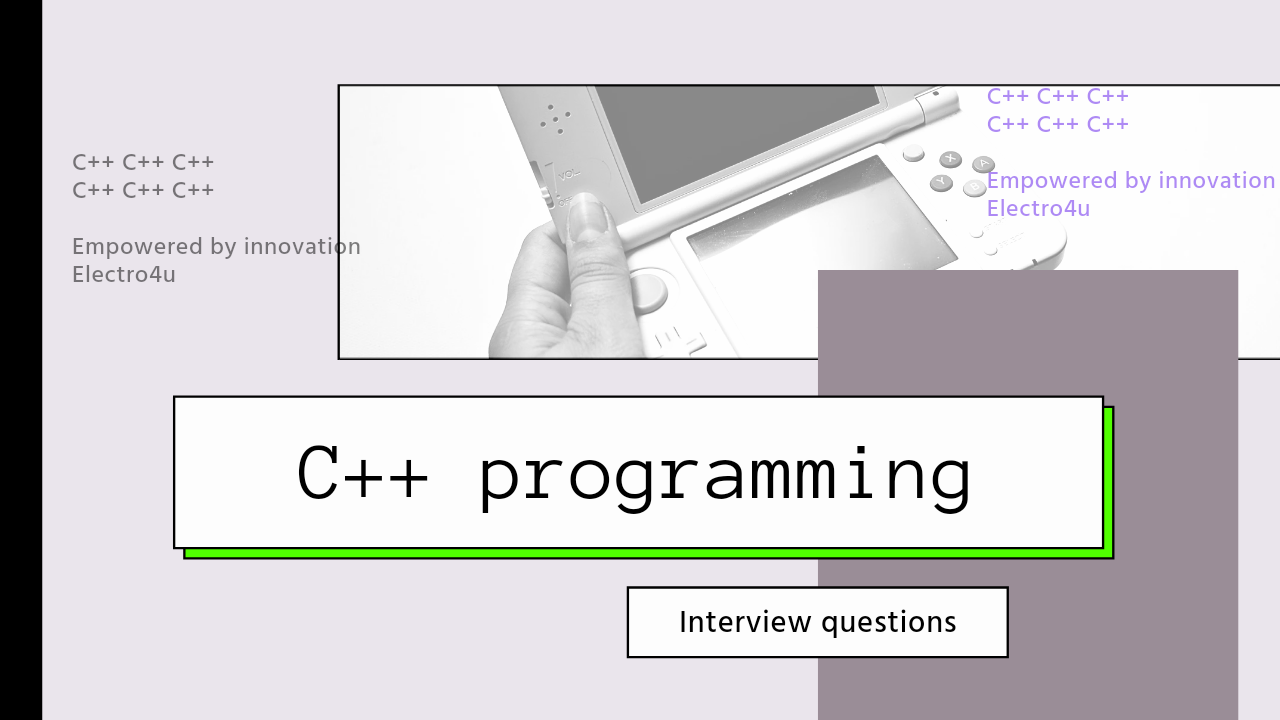
Understanding the Usage of Scope Resolution Operator in C++
Usage of Scope Resolution Operator in C++
The scope resolution operator (::) in C++ is used to access variables and functions that are defined outside of the current scope. This is useful in a number of situations, such as:
- To access a global variable when there is a local variable with the same name.
- To define a function outside of a class.
- To access the static variables and functions of a class.
- To refer to a class inside another class.
- To override a function in multiple inheritance.
Examples of how to use the scope resolution operator in C++:
C++
// Accessing a global variable when there is a local variable with the same name.
int global_var = 10;
int main() {
int local_var = 20;
std::cout << "global_var = " << ::global_var << std::endl;
std::cout << "local_var = " << local_var << std::endl;
return 0;
}
Output:
global_var = 10
local_var = 20
Defining a function outside of a class.
C++
// Defining a function outside of a class.
void my_function() {
std::cout << "Hello, world!" << std::endl;
}
int main() {
my_function();
return 0;
}
Output:
Hello, world!
Accessing the static variables and functions of a class.
C++
// Accessing the static variables and functions of a class.
class Myclass {
public:
static int static_var;
static void static_function() {
std::cout << "Static function!" << std::endl;
}
};
int Myclass::static_var = 10;
int main() {
std::cout << Myclass::static_var << std::endl;
Myclass::static_function();
return 0;
}
Output:
10
Static function!
Referring to a class inside another class.
C++
// Referring to a class inside another class.
class MyClass1 {
public:
class MyClass2 {
public:
void my_function() {
std::cout << "Hello, world!" << std::endl;
}
};
};
int main() {
MyClass1::MyClass2 my_object;
my_object.my_function();
return 0;
}
Output:
Hello, world!
Overriding a function in multiple inheritance.
C++
// Overriding a function in multiple inheritance.
class BaseClass {
public:
virtual void my_function() {
std::cout << "Base class function." << std::endl;
}
};
class DerivedClass1 : public BaseClass {
public:
void my_function() override {
std::cout << "Derived class 1 function." << std::endl;
}
};
class DerivedClass2 : public BaseClass {
public:
void my_function() override {
std::cout << "Derived class 2 function." << std::endl;
}
};
class MultiDerivedClass : public DerivedClass1, public DerivedClass2 {
public:
void my_function() override {
std::cout << "Multi derived class function." << std::endl;
}
};
int main() {
MultiDerivedClass my_object;
my_object.my_function();
return 0;
}
Output:
Multi derived class function.
The scope resolution operator is a powerful tool that can be used to write more efficient and maintainable C++ code.
MCQ. Scope Resolution operator is used ________
- To resolve the scope of global variable only
- To resolve the scope of function of the class only
- To resolve scope of the global variable as well as function of the class
- None of these
ANS: To resolve scope of the global variable as well as function of the class