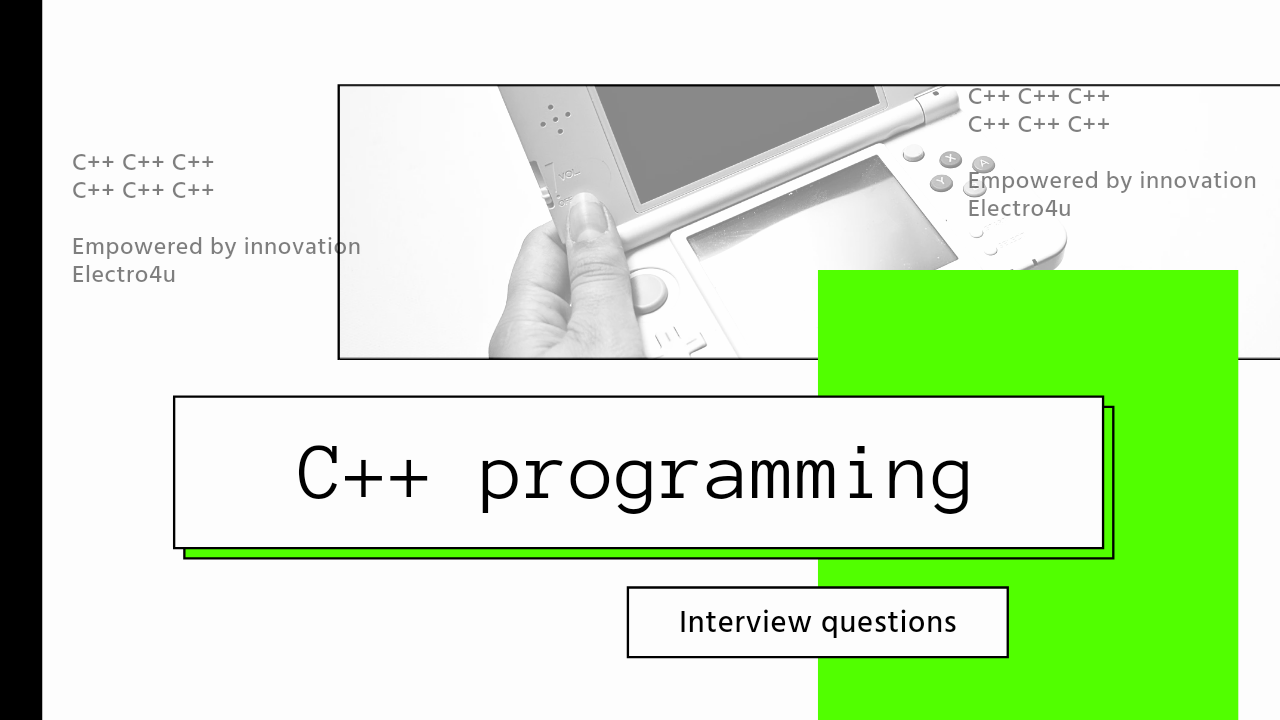
Code Reusability in C++: Best Practices and Techniques | reuse the written code in cpp?
Which concept allows you to reuse the written code in C++?
Inheritance
Inheritance is the concept of creating a new class that is based on an existing class. The new class inherits all of the properties and behaviors of the existing class, and it can also add its own new properties and behaviors.
This allows you to reuse code that you have already written, and it can help you to organize your code in a more logical way.
For example, you might have a base class called Animal that has properties such as name and species. You could then create derived classes called Dog, Cat, and Bird that inherit from the Animal class. These derived classes would have all of the properties and behaviors of the Animal class, plus their own unique properties and behaviors such as bark() for Dog, meow() for Cat, and fly() for Bird.
Example:
class Animal {
public:
std::string name;
std::string species;
};
class Dog : public Animal {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
class Cat : public Animal {
public:
void meow() {
std::cout << "Meow!" << std::endl;
}
};
int main() {
Dog dog;
dog.name = "Fido";
dog.species = "Dog";
dog.bark();
Cat cat;
cat.name = "Garfield";
cat.species = "Cat";
cat.meow();
return 0;
}
Output:Woof!
Meow!
In this example, we are reusing the code for the Animal class in the Dog and Cat classes. This saves us from having to write the same code twice, and it makes our code more organized and maintainable.
Inheritance is a powerful concept that can help you to write better and more efficient C++ code.
MCQ. Which concept allows you to reuse the written code?
- Polymorphism
- Abstraction
- Encapsulation
- Inheritance
Further Reading:
For further information and examples, Please visit[ course in production]
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!