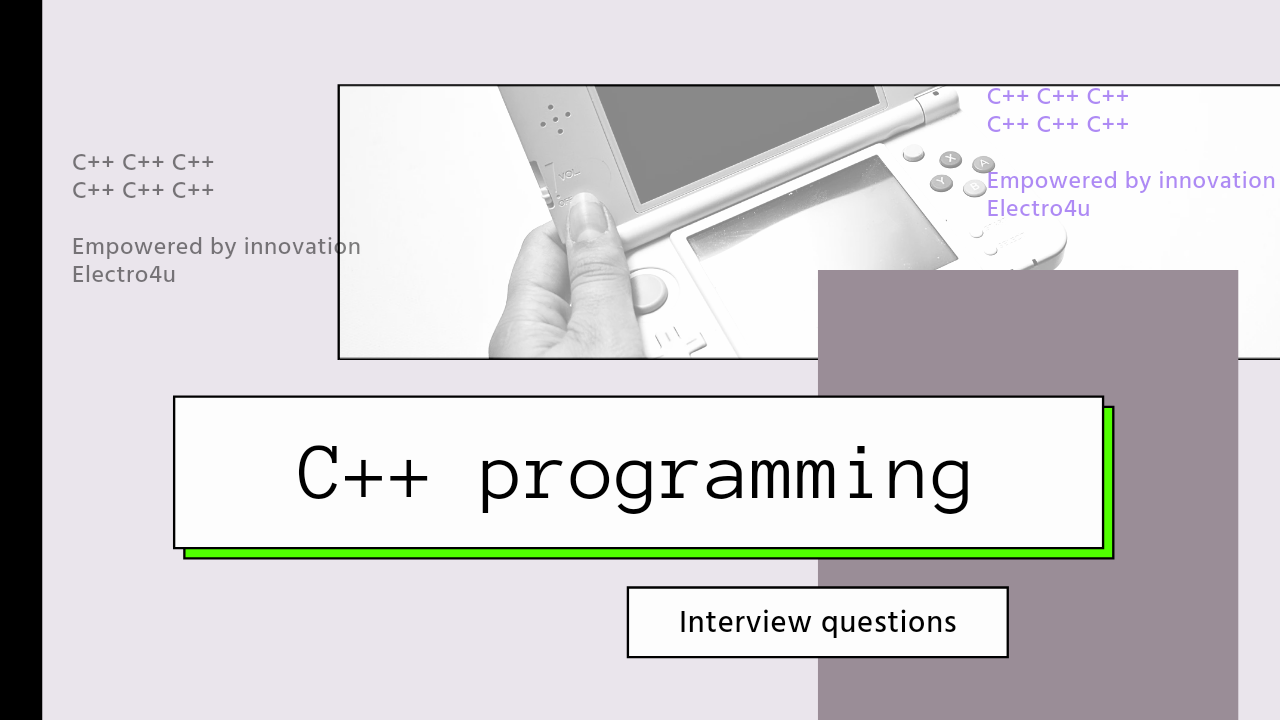
Wrapping Data in C++
Wrapping data and its related functionality into a single entity is known as
Wrapping data and its related functionality into a single entity in C++ is called encapsulation. It is one of the fundamental principles of object-oriented programming (OOP) and refers to the bundling of data and methods that operate on that data into a single unit.
Encapsulation has a number of benefits, including:
- Data protection: Encapsulation allows you to hide the internal implementation of a class from the outside world. This can help to protect your data from accidental or malicious modification.
- Code readability and maintainability: Encapsulation makes your code more readable and maintainable by grouping related data and functionality together.
- Reusability: Encapsulation allows you to create reusable classes that can be used in different parts of your program.
To implement encapsulation in C++, you can use a class. A class is a blueprint for creating objects, which are self-contained entities that contain both data and functionality.
Class that encapsulates the data and functionality for a point in two-dimensional space:
class Point {
public:
// Constructor
Point(int x, int y) : x_(x), y_(y) {}
// Getters
int GetX() const { return x_; }
int GetY() const { return y_; }
// Setters
void SetX(int x) { x_ = x; }
void SetY(int y) { y_ = y; }
// Other methods
double GetDistanceToOrigin() const { return sqrt(pow(x_, 2) + pow(y_, 2)); }
private:
int x_;
int y_;
};
This class has three public methods: GetX(), GetY(), and GetDistanceToOrigin(). These methods allow the user to access and modify the point's data, but they do not expose the internal implementation of the class.
The class also has two private data members: x_ and y_. These data members are hidden from the outside world, which helps to protect the point's data from accidental or malicious modification.
To use the Point class, you can create an object like this:
Point point(1, 2);
int x = point.GetX();
int y = point.GetY();
point.SetX(3);
point.SetY(4);
You can also call the GetDistanceToOrigin() method to get the distance between the point and the origin:
double distance = point.GetDistanceToOrigin();
Encapsulation is a powerful tool that can help you to write more secure, readable, maintainable, and reusable code.
Here are some additional points about wrapping data and its related functionality into a single entity in C++:
- Encapsulation can be used to implement other OOP concepts, such as inheritance and polymorphism.
- Encapsulation can also be used to create more abstract APIs, which can make your code more flexible and easier to use.
- Encapsulation is a key principle of good software design, and it is widely used in large and complex C++ codebases.
Wrapping data and its related functionality into a single entity is known as
- Polymorphism
- Abstraction
- Encapsulation
- Modularity
Further Reading:
For further information and examples, Please visit[ course in production]
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!