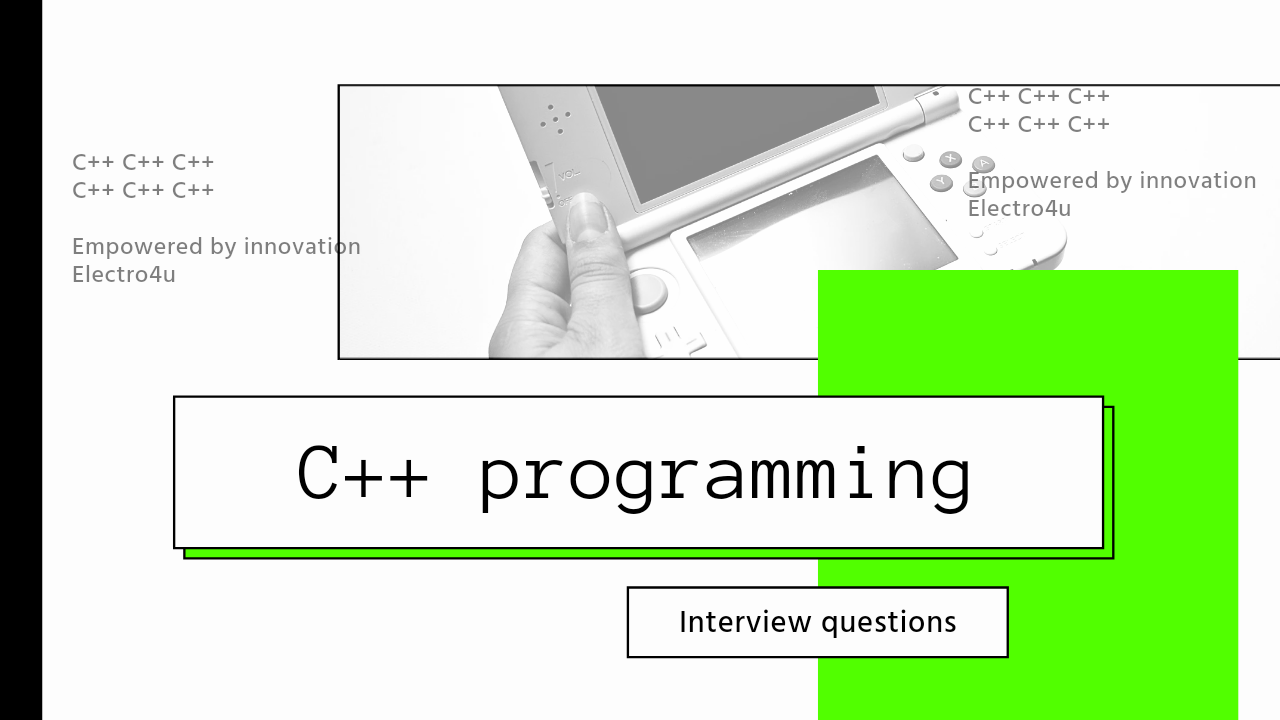
Extraction/get from Operator in cpp?
Extraction/Get From Operator in C++
The extraction operator ( >> ) is used to read data from an input stream into a variable. It is a binary operator, meaning that it takes two operands: an input stream object on the left and a variable on the right.
The extraction operator is predefined for all standard C++ data types, such as int, float, and char. It can also be overloaded to read custom data types.
To use the extraction operator, simply place it between the input stream object and the variable where you want to store the data. For example, the following code reads an integer from the standard input stream and stores it in the variable number:
int number;
cin >> number;
The extraction operator will continue to read characters from the input stream until it encounters a whitespace character, such as a space or a newline character. The whitespace character will then be discarded.
If the input stream does not contain any valid data for the type of variable on the right-hand side of the extraction operator, the operation will fail. This can happen if the input stream is empty or if it contains invalid characters.
Example:
how to use the extraction operator:
#include <iostream>
using namespace std;
int main() {
int number;
string name;
cout << "Enter a number: ";
cin >> number;
cout << "Enter your name: ";
cin >> name;
cout << "Hello, " << name << "!" << endl;
cout << "The number you entered is " << number << endl;
return 0;
}
Output:
Enter a number: 10
Enter your name: Alice
Hello, Alice!
The number you entered is 10
Overloading the Extraction Operator
The extraction operator can be overloaded to read custom data types. To do this, you must provide a friend function for the data type that takes an input stream object and a reference to the data type as parameters. The friend function should then read the data from the input stream and store it in the data type.
how to overload the extraction operator for a custom data type called Point:
class Point {
public:
int x;
int y;
friend istream& operator>>(istream& in, Point& point) {
in >> point.x >> point.y;
return in;
}
};
Point point;
cin >> point;
- <<
- >
- >>
- <
Further Reading:
For further information and examples, Please visit[ course in production]
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!