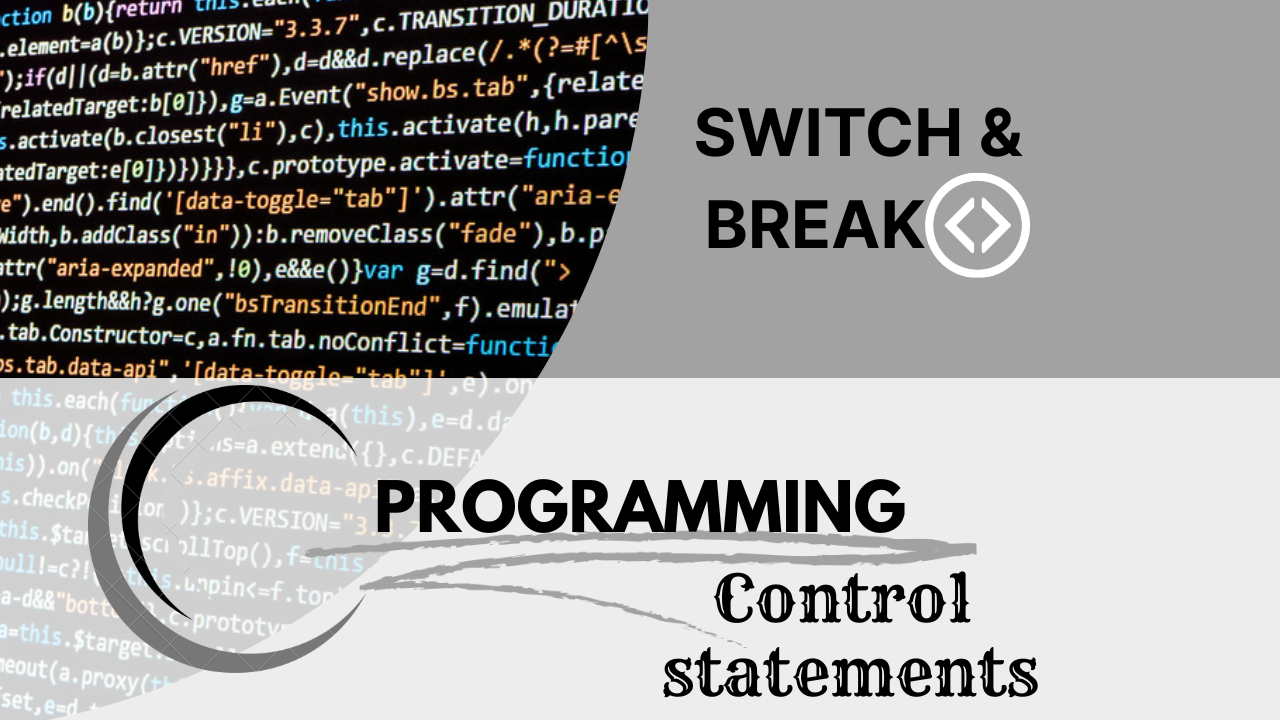
Switch and Break control statements in c programming
Mastering Switch and Break Statements in C Programming
In C programming, the switch and break control statements are used for decision-making and control flow.
Switch statement: The switch statement is a selection statement that allows you to choose one of several code blocks to be executed based on the value of an expression. Its syntax is as follows:
The switch is a conditional noniterative control statement. it is used for menu-based program
Syntax:
switch (expression) {
case constant1:
// code block executed if expression matches constant1
break;
case constant2:
// code block executed if expression matches constant2
break;
// more cases...
default:
// code block executed if expression does not match any constant
}
Here's how it works:
- The expression is evaluated, and its value is compared with the constants specified in the case labels.
- If a match is found, the code block following that case label is executed until a break statement is encountered or the end of the switch statement is reached.
- The break statement is used to exit the switch statement. Without a break statement, control will fall through to the next case, executing the code block of that case as well. This is known as "fall-through."
- If no match is found, and a default label is specified, the code block following the default label is executed.
- It's important to include a break statement at the end of each case (except the last one) to prevent fall-through if it's not intended.
Break statement: The break statement is primarily used to exit from a loop or switch statement. Its usage within a loop causes an immediate termination of the loop, and in a switch statement, it terminates the switch block. Here's the syntax:
break;
- When a break statement is encountered, the control exits the innermost loop or switch statement, and program execution continues with the next statement after the loop or switch.
- If multiple nested loops or switch statements are present, the break statement will only exit the innermost one.
- In the case of a switch statement, the break statement prevents fall-through to the next case.
Both switch and break statements provide control flow and decision-making capabilities in C programming, making them useful for handling different cases and controlling program execution based on conditions.
Note:
Label: The label should be an integers family constant
Default: the default case is not mandatory it is optional or t is preferable and suggestible
Break: The Break is an exit control loop, once the break control encounter loop is terminated
Further Reading:
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!