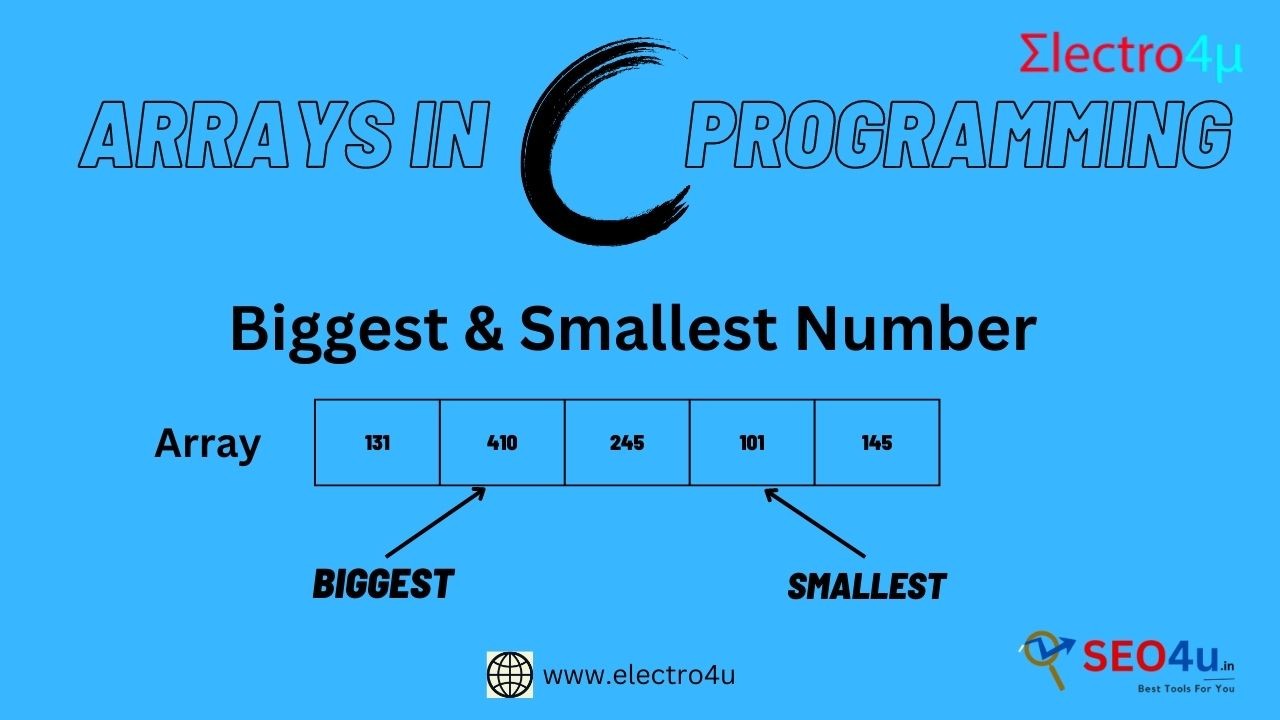
write a c program take 10 numbers through the keyword into an array and find the biggest and smallest number in an unsorted array without using any shorting Technique.
Finding the Largest and Smallest Numbers in an Unsorted Array
10 | 20 | 30 | 40 | 50 | 60 | 70 | 80 | 10 | 100 |
biggest:100
smallest:10
Introduction
- Welcome to this C programming tutorial where we'll learn how to find the largest and smallest numbers in an unsorted array without using any sorting techniques.
Program Description
- The program will take 10 numbers as input from the user through the keyboard.
- These numbers will be stored in an array.
- The program will then determine the largest and smallest numbers within this unsorted array.
Program Logic
- We'll use a loop to read the numbers and store them in an array.
- Then, we'll iterate through the array to find the largest and smallest numbers.
- We'll compare each element with the current known largest and smallest numbers to update them if necessary.
Code Implementation
- Below is the C code that accomplishes this task:
#include <stdio.h>
int main() {
int numbers[10];
int i;
// Reading numbers from the user
printf("Enter 10 numbers:\n");
for(i = 0; i < 10; i++) {
scanf("%d", &numbers[i]);
}
// Assuming the first number as both the largest and smallest
int largest = numbers[0];
int smallest = numbers[0];
// Finding the largest and smallest numbers
for(i = 1; i < 10; i++) {
if(numbers[i] > largest) {
largest = numbers[i];
}
if(numbers[i] < smallest) {
smallest = numbers[i];
}
}
// Printing the results
printf("The largest number is: %d\n", largest);
printf("The smallest number is: %d\n", smallest);
return 0;
}
Usage
- You can copy the code above into your preferred C programming environment.
- Run the program, and it will prompt you to enter 10 numbers.
- After you've entered the numbers, the program will display the largest and smallest numbers.
For further information and examples, Please visit C-Programming From Scratch to Advanced 2023-2024
Conclusion
- Congratulations! You've successfully created a C program that finds the largest and smallest numbers in an unsorted array without using any sorting techniques.