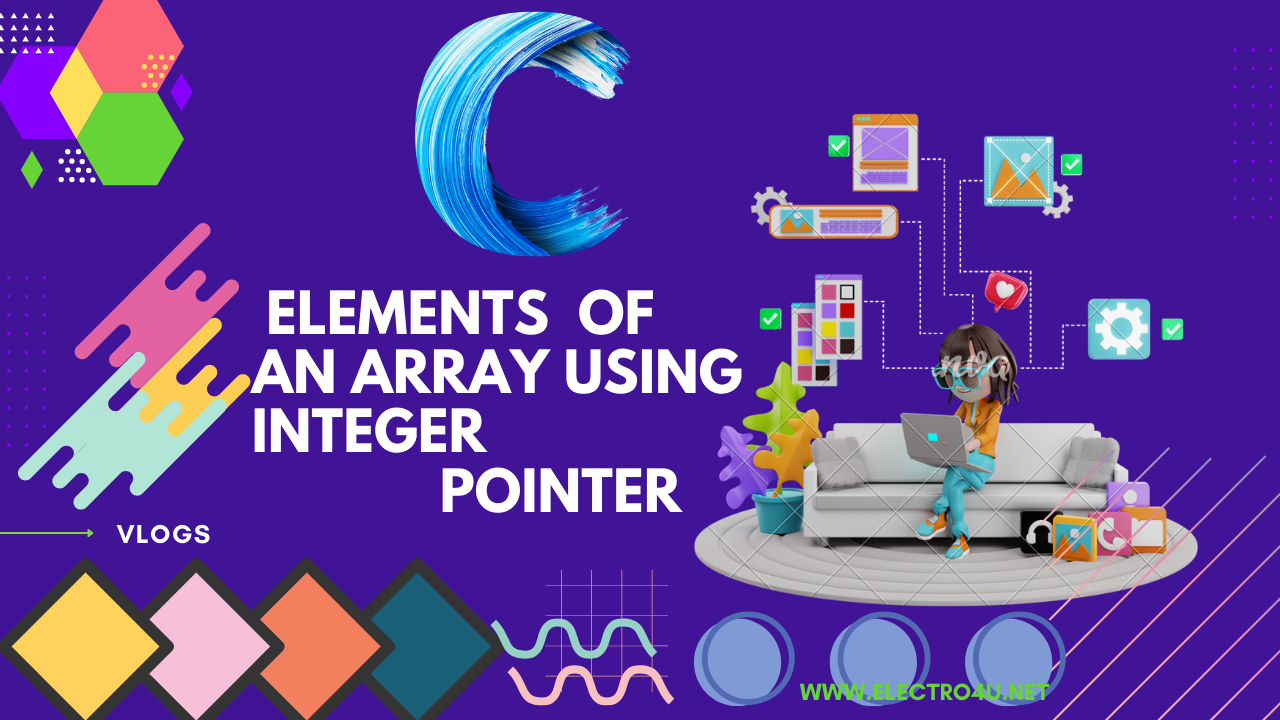
Print Array Elements Using Integer Pointers in C with electro4u
Printing Array Elements Using Integer Pointer in C
Introduction
Welcome to our web page where we'll guide you through the process of writing a C program to print the elements of an array using integer pointers. Whether you're a beginner or looking to refresh your knowledge, this page will provide you with a clear and concise explanation.
Point 1: Understanding the Objective
- Before we dive into the code, let's understand the objective. We want to print the elements of an array in C using integer pointers. This means we'll access the array elements through pointers to integers.
Point 2: Declaring an Array
- First, declare an array in C. For example, int arr[] = {1, 2, 3, 4, 5}; creates an array of integers with five elements.
Point 3: Creating an Integer Pointer
- Next, create an integer pointer. You can do this with int *ptr;, where ptr is the pointer variable.
Point 4: Pointing to the Array
- Use the pointer to point to the first element of the array by assigning ptr = arr;. Now, ptr points to the first element of arr.
Point 5: Printing Array Elements
- To print the array elements, use a loop. A for loop is commonly used for this purpose.
-
In this example, we iterate through the array using the pointer, printing each element. We also increment the pointer to move to the next element.for (int i = 0; i < 5; i++) { printf("%d ", *ptr); ptr++; // Move the pointer to the next element }
-
Point 6: Full Code Example
- Provide a complete code example to demonstrate the process from start to finish.
#include <stdio.h> int main() { int arr[] = {1, 2, 3, 4, 5}; int *ptr = arr; for (int i = 0; i < 5; i++) { printf("%d ", *ptr); ptr++; } return 0; }
Conclusion:
- Designing a web page to explain how to print array elements using integer pointers in C involves breaking down the process into clear and concise points. This approach helps users understand the concept and enables them to write the code successfully. If you have any questions or need further assistance, feel free to reach out. Happy coding!
-
Further Reading:
For further information and examples, Please visit[ C-Programming From Scratch to Advanced 2023-2024]
Top Resources
- Learn-C.org
- C Programming at LearnCpp.com
- GeeksforGeeks - C Programming Language
- C Programming on Tutorialspoint
- Codecademy - Learn C
- CProgramming.com
- C Programming Wikibook
- C Programming - Reddit Community
- C Programming Language - Official Documentation
- GitHub - Awesome C
- HackerRank - C Language
- LeetCode - C