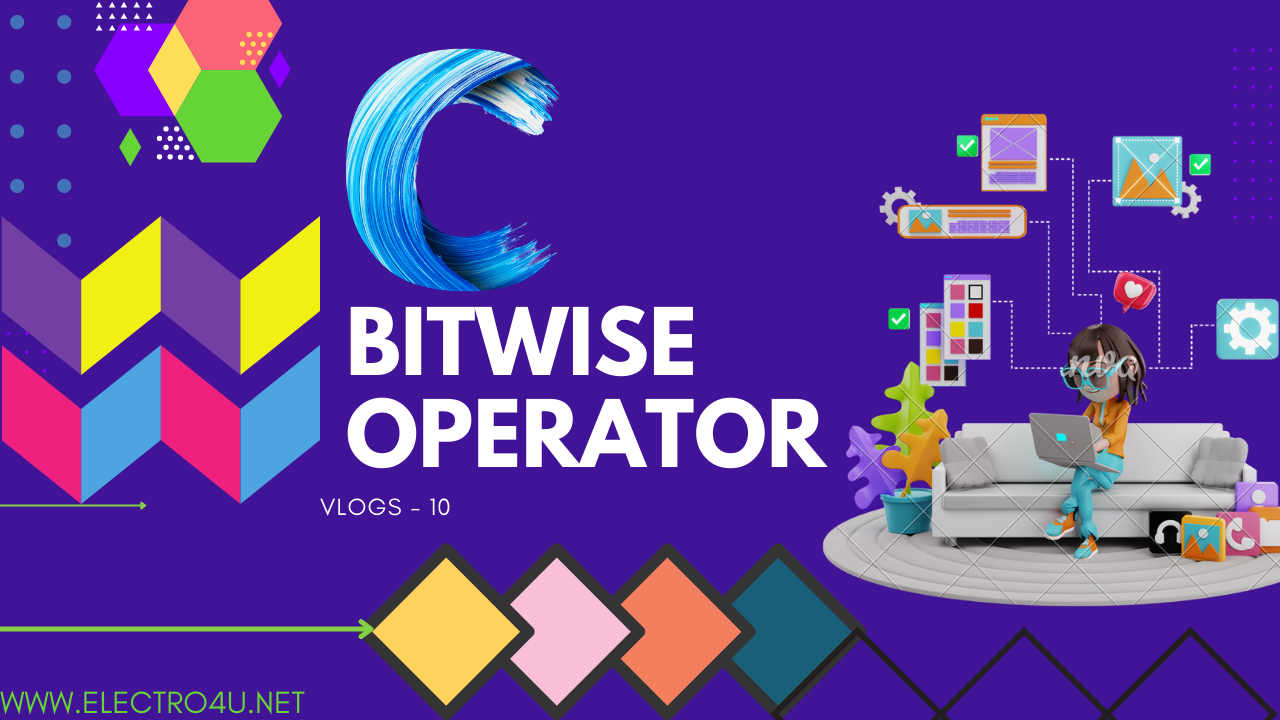
Bitwise operator in c programming
Bitwise Operators in C Programming
Bitwise Operators: Bitwise operators are used for operations on individual bits. Bitwise operators operate on integers only, C has the ability to support the manipulation of data at the bit level
In C programming, bitwise operators are used to perform bitwise operations on the binary representation of integers. There are six bitwise operators in C
Bitwise Operators are
Symbol | Bitwise operator |
& | Bitwise AND |
| | Bitwise OR |
^ | Bitwise XOR |
~ | One's complement |
<< | Left shift |
>> | Right shift |
Note:
- Except for One's complement (~) all bitwise operators are binary Operators, which means ~ is a unary operator.
- all These Operators will not modify the operand
- we can not use real numbers on a bitwise operator
Bitwise AND (&)
Bitwise AND operator required two operands. Each bit in the result is 1 only if both corresponding bits in the two input operands are 1.
A | B | A&B |
0 | 0 | 0 |
0 | 1 | 0 |
1 | 0 | 0 |
1 | 1 | 1 |
Bitwise OR( | )
The (vertical bar) operator performs a bitwise OR on two integers. Each bit in the result is 1 if either of the corresponding bits in the two input operands is 1.
A | B | A&B |
0 | 0 | 0 |
0 | 1 | 0 |
1 | 0 | 0 |
1 | 1 | 1 |
Bitwise XOR (^)
The (caret) operator performs a bitwise exclusive-OR on two integers. Each bit in the result is 1 if one, but not both, of the corresponding bits in the two input operands is 1.
A | B | A&B |
0 | 0 | 0 |
0 | 1 | 0 |
1 | 0 | 0 |
1 | 1 | 1 |
Program: Swapping of two numbers using bitwise XOR operator
#include
int main()
{
int n1,n2;
printf("Enter two number n1 & n2:");
scanf("%d%d",&n1,&n2);
printf("Before swapping n1:%d and n2:%d\n",n1,n2);
n2=n1^n2;
n1=n1^n2;
n2=n1^n2;
printf("After swapping n1:%d and n2:%d\n",n1,n2);
}
Output:
Enter two number n1 & n2:10 20
Before swapping n1:10 and n2:20
After swapping n1:20 and n2:10
Bitwise One's complement (~)
The ~(tilde) operator performs a bitwise complement on its single integer operand. 2 (The operator is therefore a unary operator, like and the unary -, &, and * operators.) Complementing a number means to change all the 0 bits to 1 and all the 1s to Os.
------------------------------------------
1 1 1 1 1 1 1 1 1 0 1 0 1 0 0 1
What is the difference between logical NOT (!) and Bitwise compliment(~)
- Both are unary operator
- ! comes to logical not but ~ comes to bitwise operator
- in bitwise compliment, for the result, we need to use 1's and 2's to get the result
Shift Operator
in the shift operator, only the first operand converts the binary no need to convert the second operand to binary. the second operand should not be a negative second operand must and should be a Positive (+ve) number.
Formula to calculate the shift result
R=num*2^shift
There are two types of shift operator
Bitwise LeftShift (<<)
Bitwise RightShift (>>)
Bitwise Leftshift oerator(<<)
Bitwise Leftshift (<<) operator shifts its first operand left by a number of bits given by its second operand, filling in new 0 bits at the right side.
Bitwise RightShift (>>)
Bitwise RightShift (>>) is the first operand right. If the first operand is unsigned, >> fills in 0 bits from the left, but if the first operand is signed, >> might fill in 1 bit if the high-order bit was already 1. (Uncertainty like this is one
the reason why it's usually a good idea to use all unsigned operands when working with the bitwise operators.)
Program: Write a Program to set a bit a given number
#include
int main()
{
int num,pos;
printf("Enter The number:\n");
scanf("%d",&num);
printf("Enter the bits position:\n");
scanf("%d",&pos);
printf("Before set number:%d\n",num);
num=num|(1<<pos);
printf("After set number: %d\n",num);
}
Output:
Enter The number: 10
Enter the bits position: 2
Before set number:10
After set number: 14
Program: Write a program to clear a bit in a given number
#include
int main()
{
int num,pos;
printf("Enter The number:\n");
scanf("%d",&num);
printf("Enter the bits position:\n");
scanf("%d",&pos);
printf("Before set number:%d\n",num);
num=num&~(1<<pos);
printf("After set number: %d\n",num);
}
Output
Enter The number: 10
Enter the bits position: 3
Before set number:10
After set number: 2
Program: write a program to compare a bit in a given number
#include
int main()
{
int num,pos;
printf("Enter The number:\n");
scanf("%d",&num);
printf("Enter the bits position:\n");
scanf("%d",&pos);
printf("Before set number:%d\n",num);
num=num^(1<<pos);
printf("After set number: %d\n",num);
}
Output:
Enter The number:10
Enter the bits position:2
Before set number:10
After set number: 14
Note: some formula
set a bit:
Num=num|1<<pos
clear a bit: Num=Num&(1<<pos)
compare a bit: Num^(1<<pos)