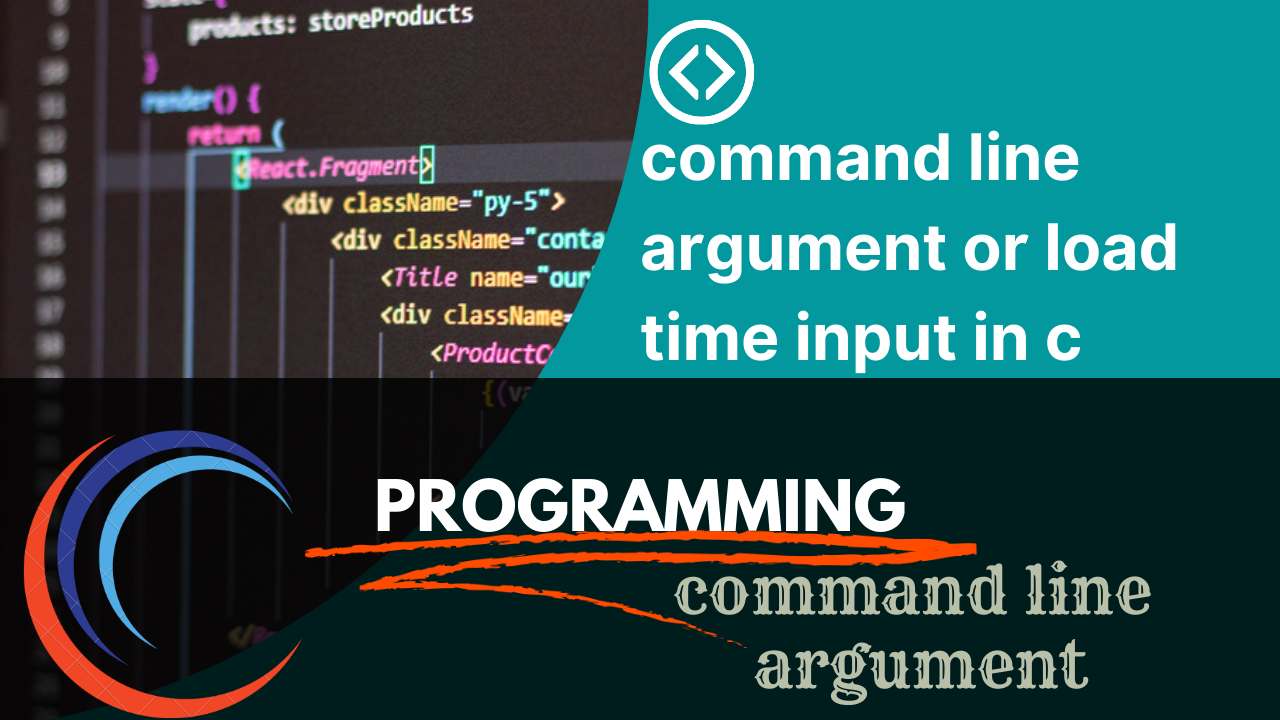
command line argument in c- load time input in c
Command line arguments in C are the arguments that are passed to the program when it is executed. These arguments can be used to control the program's behavior or to provide input to the program.
To access command line arguments in C, the main function must have at least two arguments:
- argc: This argument contains the number of command line arguments that were passed to the program.
- argv: This argument is an array of pointers to the command line arguments.
what is argc :
argc holds the number of arguments we are passing throw the command line including the name of the executable file where each argument separated with space
#include
void main()
{
printf("argc=%d\n",argc);
}
what is argv:
argv holds the base address of an array of pointers where each pointer points to one command line argument and at last null pointer present
Note; whatever you passed the command line is treated as a string
How to access command line arguments in C:
int main(int argc, char *argv[]) {
// Print the number of command line arguments.
printf("Number of command line arguments: %d\n", argc);
// Print each of the command line arguments.
for (int i = 0; i < argc; i++) {
printf("Command line argument %d: %s\n", i, argv[i]);
}
return 0;
}
To compile and run the above code, you would use the following commands:
gcc -o command_line_args command_line_args.c
./command_line_args
This would execute the program without passing any command line arguments. To pass command line arguments, you would simply add them to the end of the command when you execute the program.
For example, the following command would pass two command line arguments to the program:
./command_line_args arg1 arg2
When you run the program, it would print the following output:
Number of command line arguments: 2
Command line argument 0: command_line_args
Command line argument 1: arg1
Command line argument 2: arg2
Load time input in C
Load time input is input that is provided to the program when it is compiled. This can be done using the #define preprocessor directive.
To use the #define directive
to define a load time input variable,
syntax:
#define VARIABLE_NAME value
For example, the following code defines a load time input variable called INPUT_VALUE and sets it to the value 10:
#define INPUT_VALUE 10
Once the load time input variable has been defined, it can be used anywhere in the program as if it were a regular variable. For example, the following code prints the value of the INPUT_VALUE variable:
int main() { printf("Load time input value: %d\n", INPUT_VALUE); return 0; }
To compile and run the above code, you would use the following commands:
gcc -o load_time_input load_time_input.c ./load_time_input
This would execute the program and print the following output:
Load time input value: 10
Load time input can be used to provide initial values to variables or to configure the program's behavior. For example, you could use load time input to specify the name of a file to read or the number of threads to use.
Note: Three are types of main Declaration in c
- void main( )
- void main(int argc, char **argv)
- void main(int argc, char **argv,char **env)