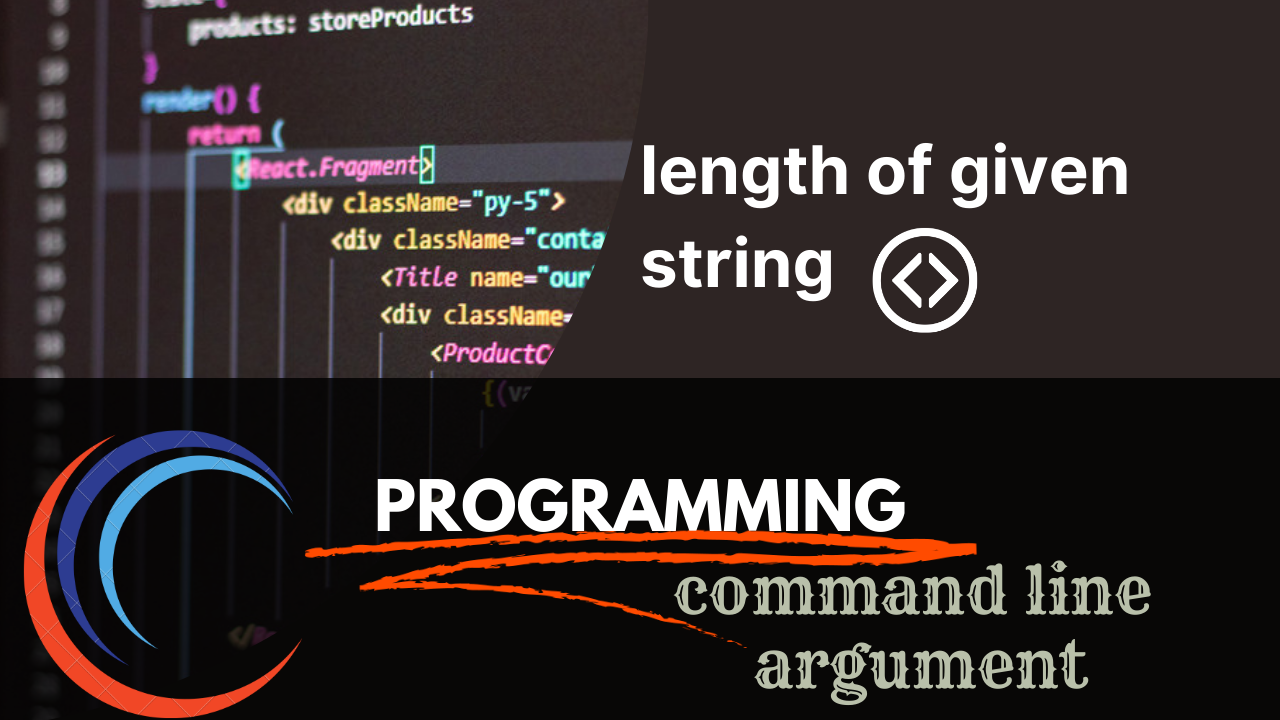
write a program for finding length of given string using command line argument in c
C Programming: Calculate String Length via Command Line
To find the length of a given string using a command-line argument in C, we can use the following steps:
- Declare a character array to store the string.
- Get the
command-line
argument using the argc and argv variables. - Copy the
command-line
argument to the character array. - Iterate over the character array until you reach the null terminator
('\0').
- Increment a counter for each character in the array.
- The counter will contain the length of the string.
C program to find the length of a given string using a command-line argument:
C Programming
#include <stdio.h>
#include <string.h>
int main(int argc, char *argv[]) {
// Declare a character array to store the string
char str[100];
// Get the command-line argument
char *arg = argv[1];
// Copy the command-line argument to the character array
strcpy(str, arg);
// Iterate over the character array until you reach the null terminator
int len = 0;
while (str[len] != '\0') {
len++;
}
// Print the length of the string
printf("The length of the string is %d\n", len);
return 0;
}
To compile and run the program, you can use the following commands:
gcc -o strlen strlen.c
./strlen <string>
For example, to find the length of the string "hello", you would use the following command:
./strlen hello
Output:
The length of the string is 5
Further Reading:
What is command line arguments? What is the use of that?
By default Command line Arguments are treated as ?
command line argument in c- load time input in c
write a program for finding length of given string using command line argument in c
write a program to convert asci to integers using command line in c- ATOI
write a program for basic calculator operation using command line in c
assignment of command line argument in c
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!