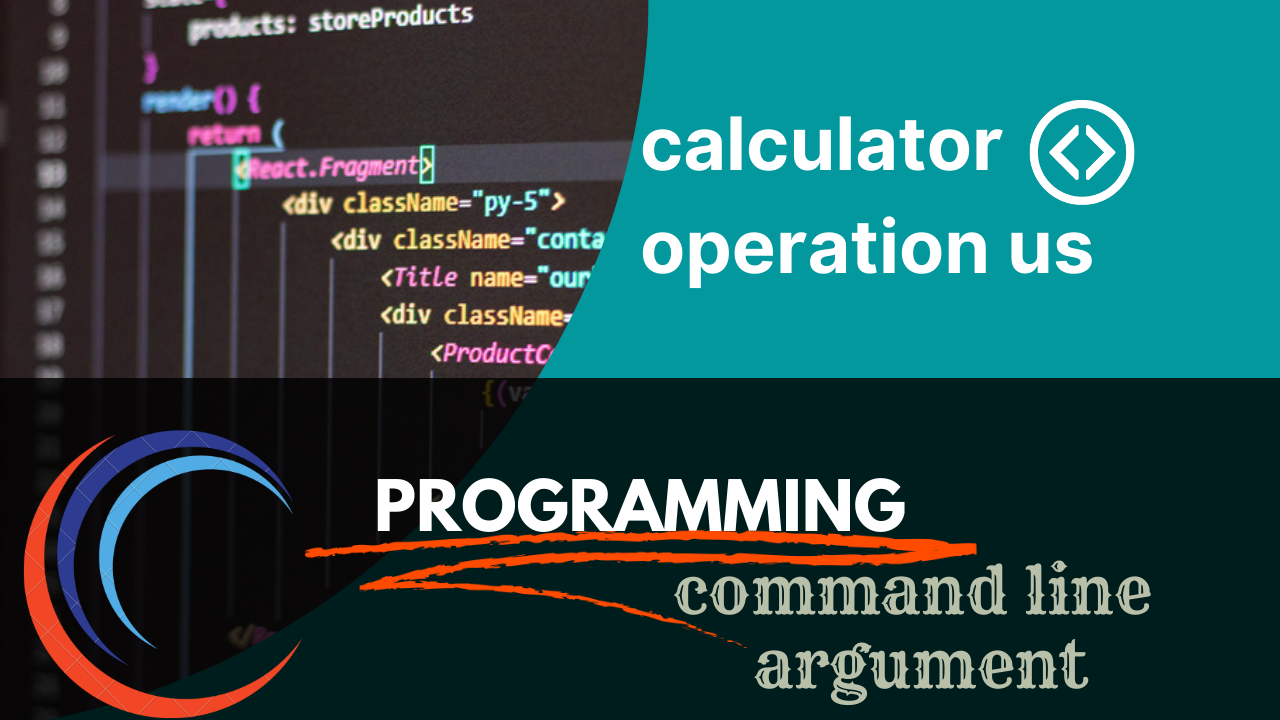
write a program for basic calculator operation using command line in c
Command Line Calculator Program in C: A Step-by-Step Guide
Program for basic calculator operations using the command line in C:
#include <stdio.h>
#include <stdlib.h>
int main(int argc, char *argv[]) {
// Check if the user has provided exactly three arguments
if (argc != 3) {
printf("Usage: calculator <operator> <number1> <number2>\n");
return 1;
}
// Convert the operator and numbers to doubles
double operator = atof(argv[1]);
double number1 = atof(argv[2]);
double number2 = atof(argv[3]);
// Perform the calculation based on the operator
double result;
switch (operator) {
case '+':
result = number1 + number2;
break;
case '-':
result = number1 - number2;
break;
case '*':
result = number1 * number2;
break;
case '/':
result = number1 / number2;
break;
default:
printf("Unsupported operator: %s\n", argv[1]);
return 1;
}
// Print the result
printf("%f\n", result);
return 0;
}
To compile and run the program, you can use the following commands:
gcc -o calculator calculator.c
./calculator <operator> <number1> <number2>
For example, to add two numbers, you would use the following command:
./calculator + 10 20
This would print the following output:
30.000000
You can use the program to perform any of the basic arithmetic operations, including addition, subtraction, multiplication, and division.
Further Reading:
Variable argument list function in c
write a program for sprint function using variable argument list function in c
write a program to access nameless argument in c - variable argument list function
assignment of variable argument list function in c
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!