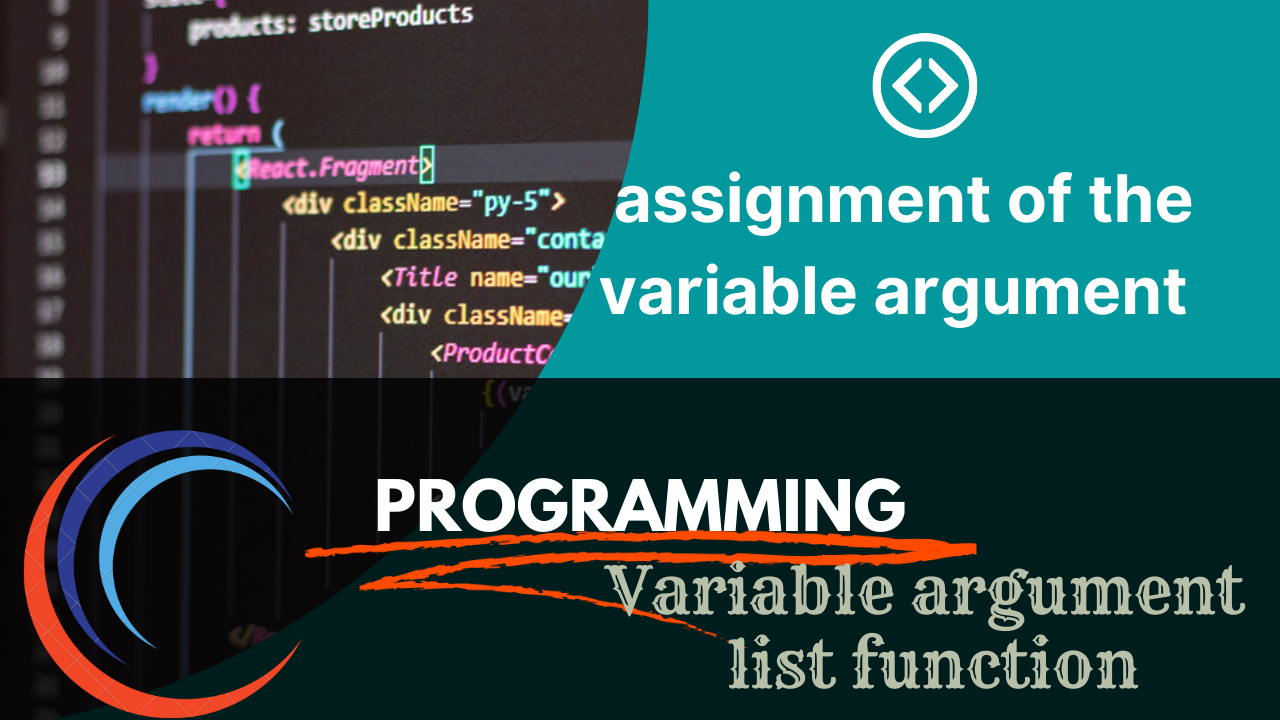
write a program to access nameless argument in c - variable argument list function
C Program: Accessing Nameless Arguments in Variable Argument List Function
To access nameless arguments in C, we can use the va_list type and the va_start()
, va_arg()
, and va_end()
macros.
The va_list
type is a special type that is used to store the information about the nameless arguments. The va_start()
macro is used to initialize a va_list
variable and to start iterating over the nameless arguments. The va_arg()
macro is used to get the next nameless argument. The va_end()
macro is used to clean up the va_list
variable.
Example of a program to access nameless arguments in C:
#include
#include
void print_args(int num_args, ...) {
va_list args;
va_start(args, num_args);
for (int i = 0; i < num_args; i++) {
int arg = va_arg(args, int);
printf("%d ", arg);
}
va_end(args);
printf("\n");
}
int main() {
print_args(3, 1, 2, 3);
print_args(5, 4, 5, 6, 7, 8);
return 0;
}
Output:
1 2 3
4 5 6 7 8
The print_args()
function takes two arguments
: the number of nameless arguments and the nameless arguments themselves
. The function first initializes a va_list variable using the va_start() macro.
Then, it iterates over the nameless arguments using the va_arg()
macro and prints each argument to the console. Finally, the function cleans up the va_list
variable using the va_end()
macro.
In the main function, we call the print_args()
function twice, once with three nameless arguments
and once with five nameless arguments
. The output of the program is as shown above.
Further Reading:
Variable argument list function in c
write a program for sprint function using variable argument list function in c
write a program to access nameless argument in c - variable argument list function
assignment of variable argument list function in c
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!