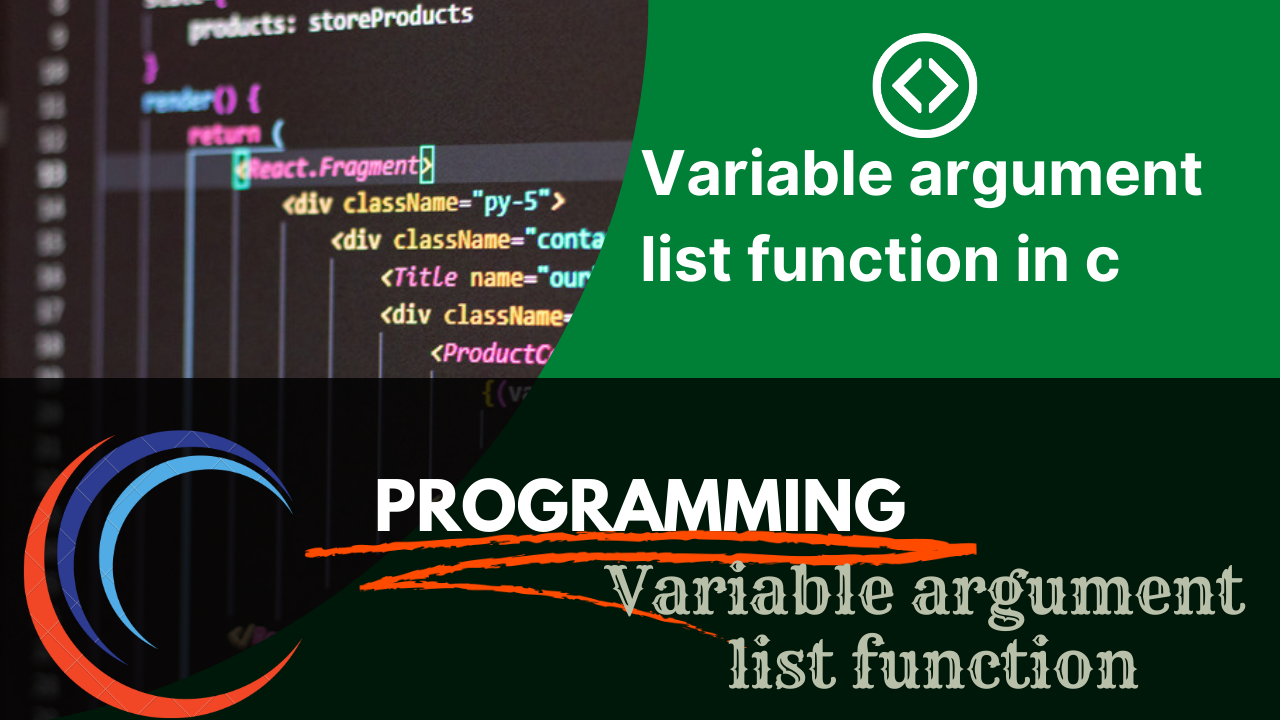
Variable argument list function in c
What is the variable argument list function in c
A variable argument list function in C is a function that can take a variable number of arguments. This is in contrast to a fixed argument list function, which can only take a fixed number of arguments.
Variable argument list functions are declared using the ellipsis (...)
operator. The ellipsis operator indicates that the function can take a variable number of arguments. The following is an example of a variable argument list function:
void my_function(int n, ...) {
// ...
}
The my_function()
function can take any number of arguments, starting with the first argument, n
. The variable argument list is accessed using the va_list type
. The va_list type
is a special type that allows you to iterate over the variable argument list.
The following is an example of how to use the va_list type
to iterate over the variable argument list in the my_function()
function:
void my_function(int n, ...) {
va_list ap;
// Initialize the va_list object
va_start(ap, n);
// Iterate over the variable argument list
while (1) {
// Get the next argument from the variable argument list
int arg = va_arg(ap, int);
// If the end of the variable argument list has been reached, break from the loop
if (arg == EOF) {
break;
}
// Do something with the argument
printf("%d ", arg);
}
// Clean up the va_list object
va_end(ap);
}
The va_start()
macro initializes the va_list
object to the first argument in the variable argument list. The va_arg()
macro gets the next argument from the variable argument list. The va_end()
macro cleans up the va_list
object.
Variable argument list functions can be used for a variety of purposes, such as:
- Printing a variable number of arguments to the console
- Calculating the sum of a variable number of arguments
- Finding the maximum or minimum value of a variable number of arguments
- Passing a variable number of arguments to another function
Example
Example of a variable argument list function that prints a variable number of arguments to the console:
void my_printf(const char *format, ...) { va_list ap; va_start(ap, format); while (*format != '\0') { if (*format == '%') { switch (*(++format)) { case 'd': printf("%d", va_arg(ap, int)); break; case 'f': printf("%f", va_arg(ap, double)); break; case 's': printf("%s", va_arg(ap, char *)); break; default: printf("%%"); break; } } else { printf("%c", *format); } ++format; } va_end(ap); }
The my_printf()
function can be used to print a variable number of arguments to the console, just like the standard printf()
function.
Conclusion
Variable argument list functions are a powerful feature of the C language. They allow you to write functions that can take a variable number of arguments. This can be useful for a variety of purposes.
Further Reading:
Variable argument list function in c
write a program for sprint function using variable argument list function in c
write a program to access nameless argument in c - variable argument list function
assignment of variable argument list function in c
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [[email protected]].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!