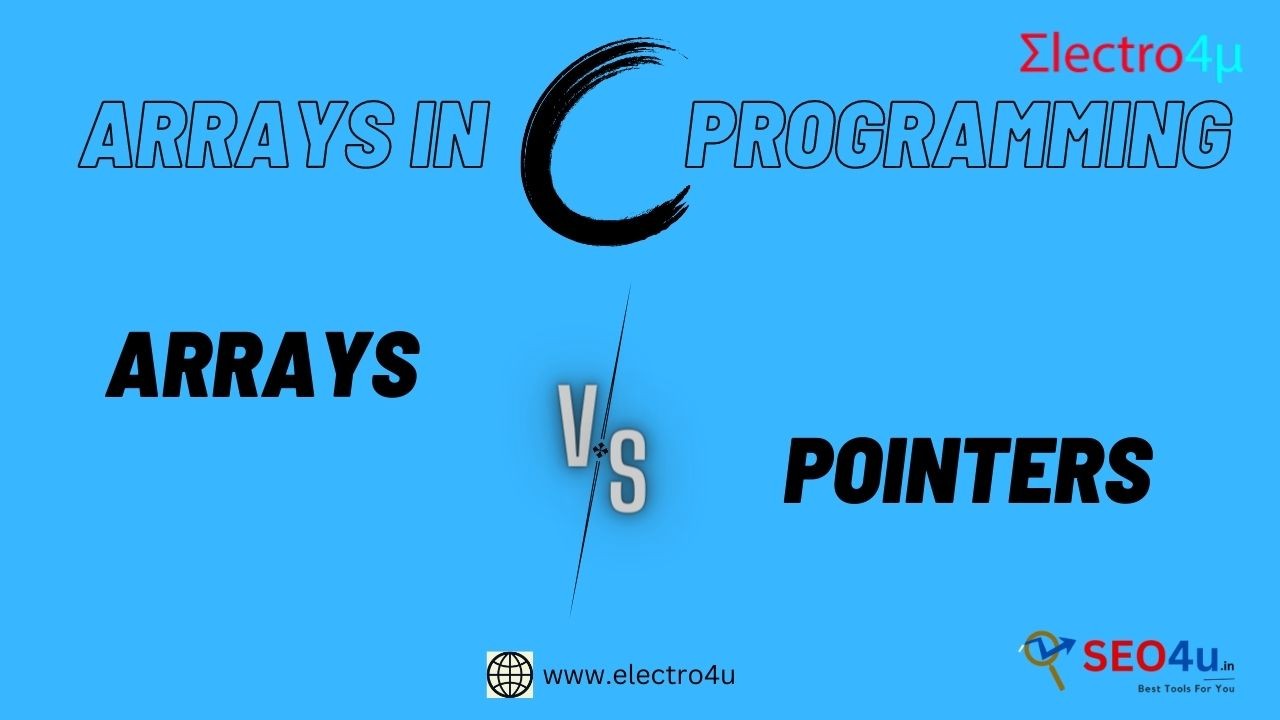
Difference between arrays and pointers?
Arrays and pointers are two different concepts in computer programming, but they are closely related and can be used interchangeably in certain contexts.
Arrays vs. Pointers: Understanding the Differences and Similarities
Arrays and pointers are two different, but related, concepts in programming.
An array is a data structure that stores a collection of elements of the same type. The elements of an array are stored in contiguous memory locations, and the array can be accessed using an index.
A pointer is a variable that stores the address of another variable. Pointers can be used to access any type of data, including arrays.
Table that summarizes the key differences between arrays and pointers:
Characteristic | Array | Pointer |
---|---|---|
Type | Data structure | Variable |
Stores | A collection of elements of the same type | The address of another variable |
Accessed using | An index | The dereference operator (*) |
Can be resized? | No | Yes |
Can be used to access any type of data? | No | Yes |
Examples of how to use arrays and pointers in C programming:
// Array example
int my_array[10]; // Declare an array of 10 integers
// Initialize the array
for (int i = 0; i < 10; i++) {
my_array[i] = i;
}
// Access the elements of the array using an index
printf("%d\n", my_array[5]); // Prints 5
// Pointer example
int* my_pointer; // Declare a pointer to an integer
// Assign the pointer the address of the first element in the array
my_pointer = &my_array[0];
// Dereference the pointer to access the element of the array
printf("%d\n", *my_pointer); // Prints 0
// Increment the pointer to access the next element of the array
my_pointer++;
// Dereference the pointer to access the next element of the array
printf("%d\n", *my_pointer); // Prints 1
Arrays and pointers are both powerful tools, but they can be difficult to learn and use correctly. If you are new to programming, it is important to take the time to learn about arrays and pointers before using them in your programs.