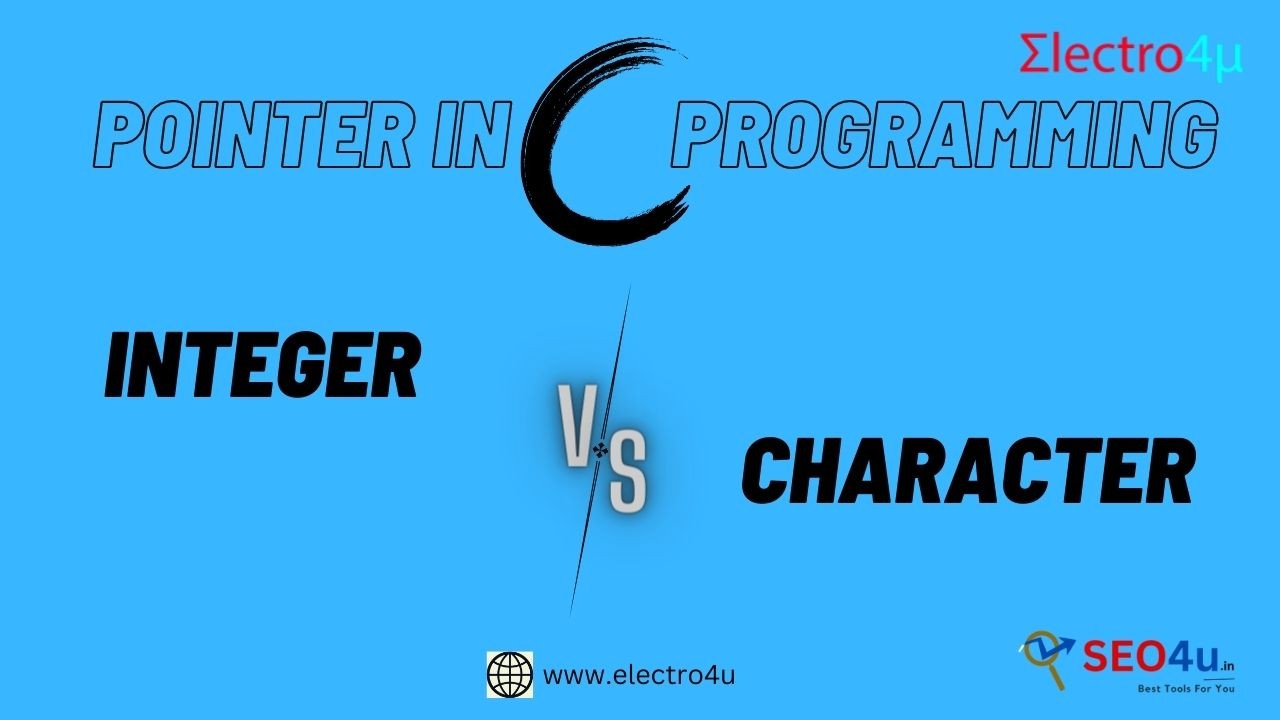
Difference between integer pointer and character pointer in c
Difference between Integer Pointer and Character Pointer in C
Integer pointer
An integer pointer is a variable that stores the address of an integer variable. Integer pointers are declared using the int* keyword. For example, the following code declares an integer pointer variable called ptr:
C programming
int* ptr;
ptr variable can store the address of any integer variable.
To assign the address of an integer variable to an integer pointer, we use the & operator. For example, the following code assigns the address of the integer variable x to the integer pointer ptr:
C programming
int x = 10;
ptr = &x;
Once the ptr pointer variable contains the address of an integer variable, we can use it to access the value of the integer variable. To do this, we use the * operator. For example, the following code prints the value of the integer variable x to the console:
C programming
printf("%d\n", *ptr);
This code will print the following output:
10
Character pointer
A character pointer is a variable that stores the address of a character variable. Character pointers are declared using the char* keyword. For example, the following code declares a character pointer variable called ptr:
C program
char* ptr;
The ptr variable can store the address of any character variable.
To assign the address of a character variable to a character pointer, we use the & operator. For example, the following code assigns the address of the character variable c to the character pointer ptr:
C program
char c = 'a';
ptr = &c;
Once the ptr pointer variable contains the address of a character variable, we can use it to access the value of the character variable. To do this, we use the * operator. For example, the following code prints the value of the character variable c to the console:
C program
printf("%c\n", *ptr);
This code will print the following output:
a
Difference between integer pointer and character pointer
The main difference between an integer pointer and a character pointer is the size of the data type that they point to. An integer pointer points to an integer, which is typically 4 bytes in size. A character pointer points to a character, which is typically 1 byte in size.
integer pointers and character pointers:
Feature | Integer Pointer | Character Pointer |
---|---|---|
Size of data pointed to | 4 bytes | 1 byte |
Dereferencing operator | * | * or [] |
This difference in size can be seen when we try to dereference the pointers. For example, the following code will print the value of the integer pointed to by ptr:
C program
int* ptr = NULL;
printf("%d\n", *ptr);
This code will cause a segmentation fault, because the ptr pointer is NULL and does not point to any valid memory location.
Code will not cause a segmentation fault, even though the ptr pointer is NULL:
C program
char* ptr = NULL;
printf("%c\n", *ptr);
This is because the ptr pointer is a character pointer, and character pointers are typically initialized to the null character (\0), which is a valid character value.
Another difference between integer pointers and character pointers is that character pointers can be used to access strings. A string is a sequence of characters terminated by a null character (\0). To access the characters of a string, we can use a character pointer to iterate over the string until we reach the null character.
Code prints the characters of the string hello to the console:
C program
char* str = "hello";
while (*str != '\0') {
printf("%c", *str);
str++;
}
This code will print the following output:
hello
Integer pointers cannot be used to access strings in this way.
I hope this helps to explain the difference between integer pointers and character pointers in C.
Further Reading:
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!