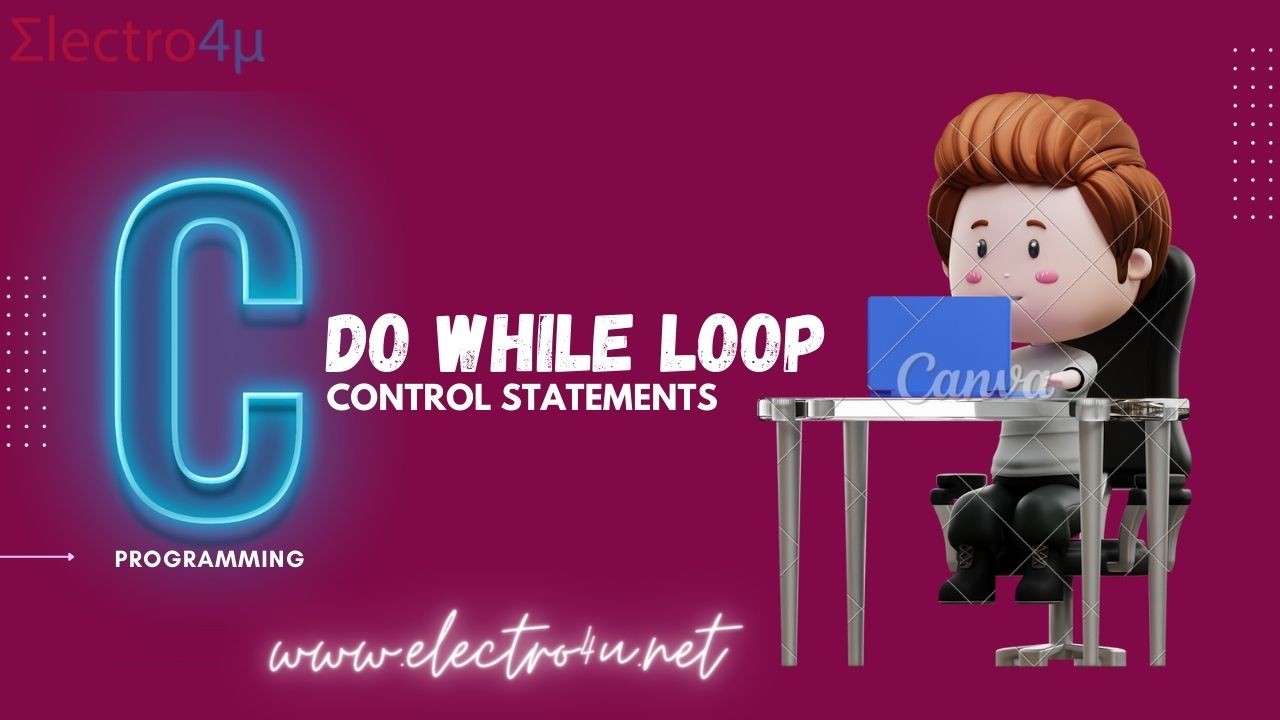
do while in c programming
Understanding the "do while" Loop in C Programming
The "do-while" loop in C programming is similar to the "while" loop, but with one key difference: the code block is executed at least once before checking the loop condition. Here's the syntax of the "do-while" loop:
syntax:
"do-while" loop works as follows:
- The code block inside the "do" statement is executed.
- After executing the code block, the condition is checked.
- If the condition is true, the loop continues, and the process repeats from step 1.
- If the condition is false, the loop is terminated, and control is transferred to the next statement after the loop.
Example that prints the numbers from 1 to 5 using a "do-while" loop:
#include <stdio.h>
int main()
{
int i = 1;
do
{
printf("%d\n", i);
i++;
}
while (i <= 5);
return 0;
}
Output:
1 2 3 4 5
In this example, the loop starts by executing the code block inside the "do" statement, which prints the value of i. After each iteration, i is incremented by 1 (i++). The condition i <= 5 is checked at the end of each iteration. If it is true, the loop continues; otherwise, the loop is terminated.
The "do-while" loop is useful when you want to ensure that the code block executes at least once, regardless of the condition. It is often used when you need to perform some operations before checking the condition, or when you want to prompt the user for input at least once.
key characteristics of the "do-while" loop:
- The loop condition is checked at the end of each iteration.
- The code block is executed at least once, irrespective of the condition.
- The loop continues as long as the condition remains true.
When deciding between a "do-while" loop, a "while" loop, or a "for" loop, consider the specific requirements of your program and choose the loop structure that best fits those requirements.
Further Reading:
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!