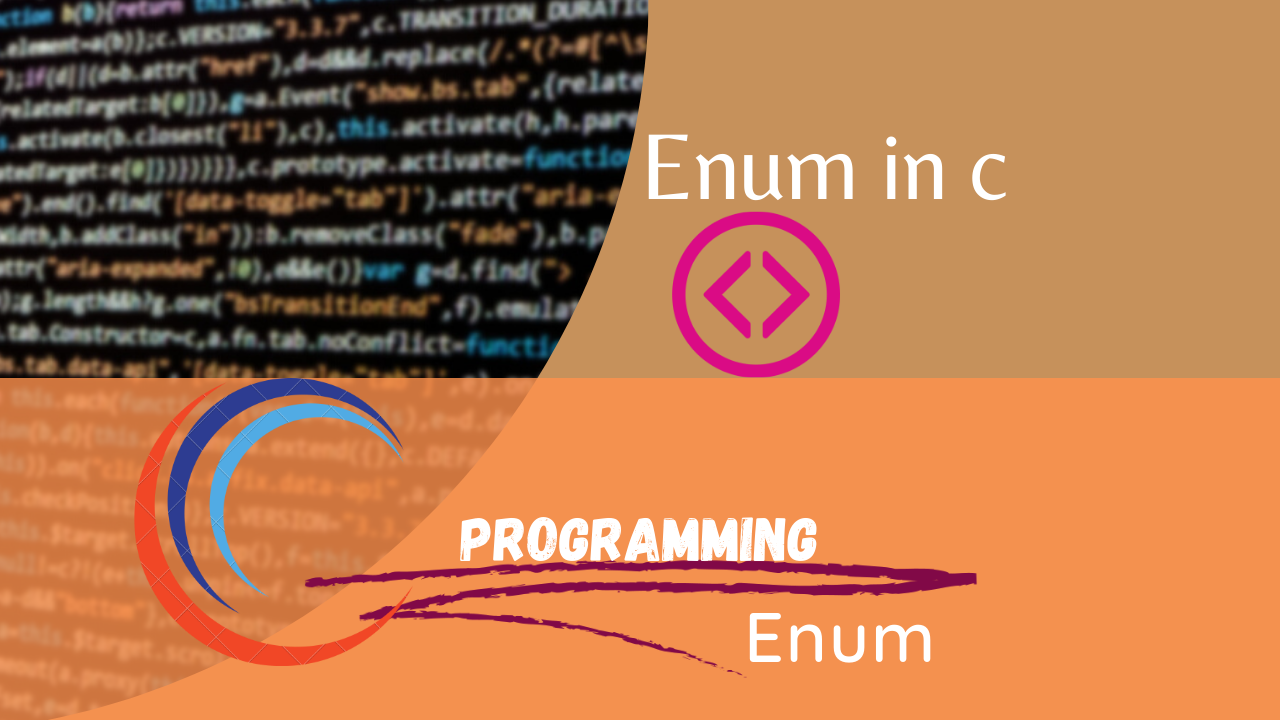
Enum in c programming with electro4u.net
Enums in C
Introduction
Enums, short for enumerations, are a user-defined data type in C that allow you to assign names to integral constants. They make the code more readable and maintainable.
Syntax
enum enum_name {
value1,
value2,
// ...
};
Example
enum Color {
RED,
GREEN,
BLUE
};
Usage
-
Defining Enums:
Enums are defined using the enum keyword followed by the enum name and a list of possible values. These values are automatically assigned integers starting from 0.
enum Days {SUNDAY, MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY};
-
Accessing Enum Values:
Enum values can be accessed using dot notation.
enum Days today = WEDNESDAY;
-
Explicitly Assigning Values:
You can explicitly assign values to enum members.
enum Status {SUCCESS=0, FAILURE=1, ERROR=2};
-
Size of Enums:
sizeof(enum) returns the size of the enum in bytes.
printf("Size of enum Status: %d bytes", sizeof(enum Status));
Benefits of Enums
-
Readability: Enums make the code more human-readable as the constants have meaningful names.
-
Maintainability: If the underlying values need to change, you only need to modify the enum definition.
-
Avoiding Magic Numbers: Enums help avoid the use of 'magic numbers' in the code, which can be hard to understand.
Conclusion
Enums are a powerful feature in C that allow you to create named constants, improving code readability and maintainability.
Further Reading:
For further information and examples, Please visit[ C-Programming From Scratch to Advanced 2023-2024]
Top Resources
- Learn-C.org
- C Programming at LearnCpp.com
- GeeksforGeeks - C Programming Language
- C Programming on Tutorialspoint
- Codecademy - Learn C
- CProgramming.com
- C Programming Wikibook
- C Programming - Reddit Community
- C Programming Language - Official Documentation
- GitHub - Awesome C
- HackerRank - C Language
- LeetCode - C
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!