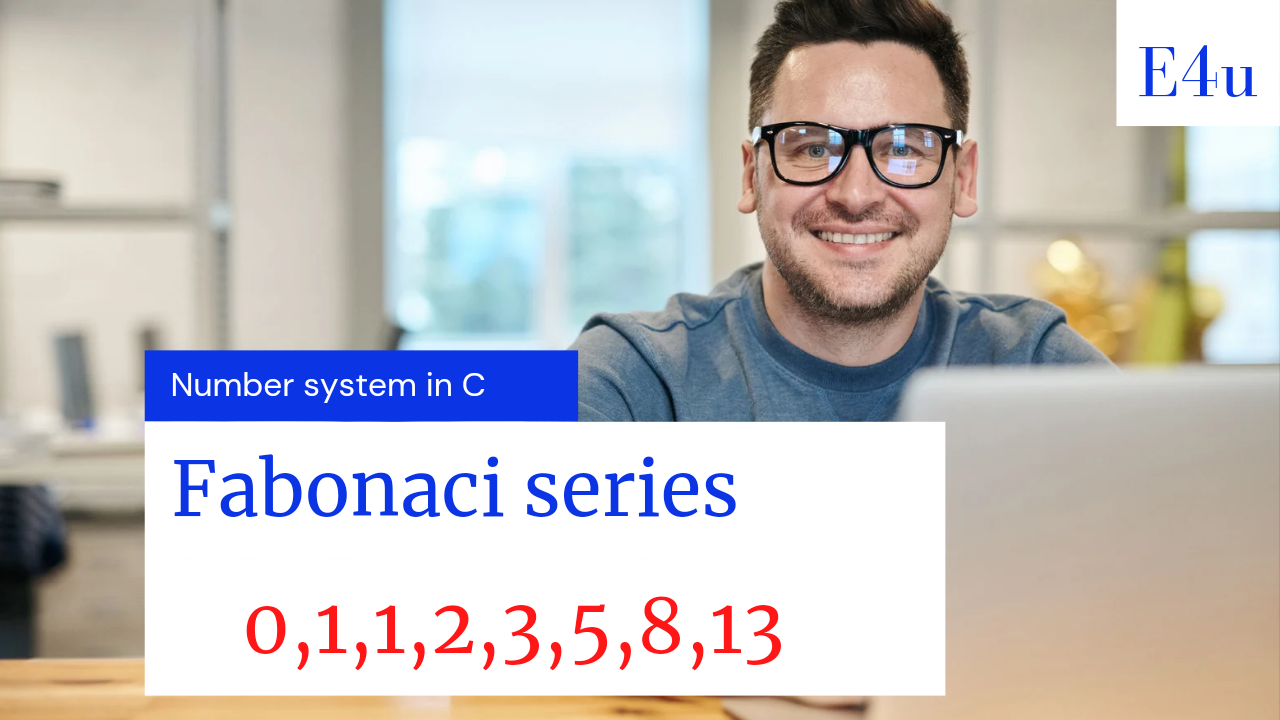
Fibonacci series in c programming
Understanding Fibonacci Series in C Programming
Introduction
- The Fibonacci series is a mathematical sequence where each number is the sum of the two preceding ones.
- It starts with 0 and 1, and the subsequent numbers are generated by adding the previous two.
Why Learn Fibonacci Series in C?
- It's a fundamental concept in programming and mathematics.
- It's a great exercise for understanding loops and recursion.
- It finds applications in various fields like mathematics, computer science, and even nature.
Fibonacci Series Algorithm in C
Using a Loop
- Initialize three variables: a (first Fibonacci number), b (second Fibonacci number), and c (next Fibonacci number).
- Use a loop to generate the Fibonacci series.
Using Recursion
- Define a recursive function to calculate the Fibonacci series.
- The base cases are typically the first two Fibonacci numbers (0 and 1).
- The function calls itself with modified parameters to calculate subsequent Fibonacci numbers.
Code Examples
Fibonacci Series using a Loop
#include <stdio.h>
int main() {
int n, a = 0, b = 1, c, i;
printf("Enter the number of terms: ");
scanf("%d", &n);
printf("Fibonacci Series: ");
for(i = 0; i < n; i++) {
if(i <= 1)
c = i;
else {
c = a + b;
a = b;
b = c;
}
printf("%d ", c);
}
return 0;
}
Fibonacci Series using Recursion
#include <stdio.h>
int fibonacci(int n) {
if(n <= 1)
return n;
return fibonacci(n-1) + fibonacci(n-2);
}
int main() {
int n, i;
printf("Enter the number of terms: ");
scanf("%d", &n);
printf("Fibonacci Series: ");
for(i = 0; i < n; i++)
printf("%d ", fibonacci(i));
return 0;
}
Tips and Best Practices
- Use the loop-based approach for efficiency in most cases.
- Be cautious with recursion as it can lead to stack overflow for large inputs.
- Implement proper error handling for user inputs.
Conclusion
- Understanding and implementing the Fibonacci series is a crucial skill for any programmer.
- Practice and experiment with different methods to solidify your understanding.