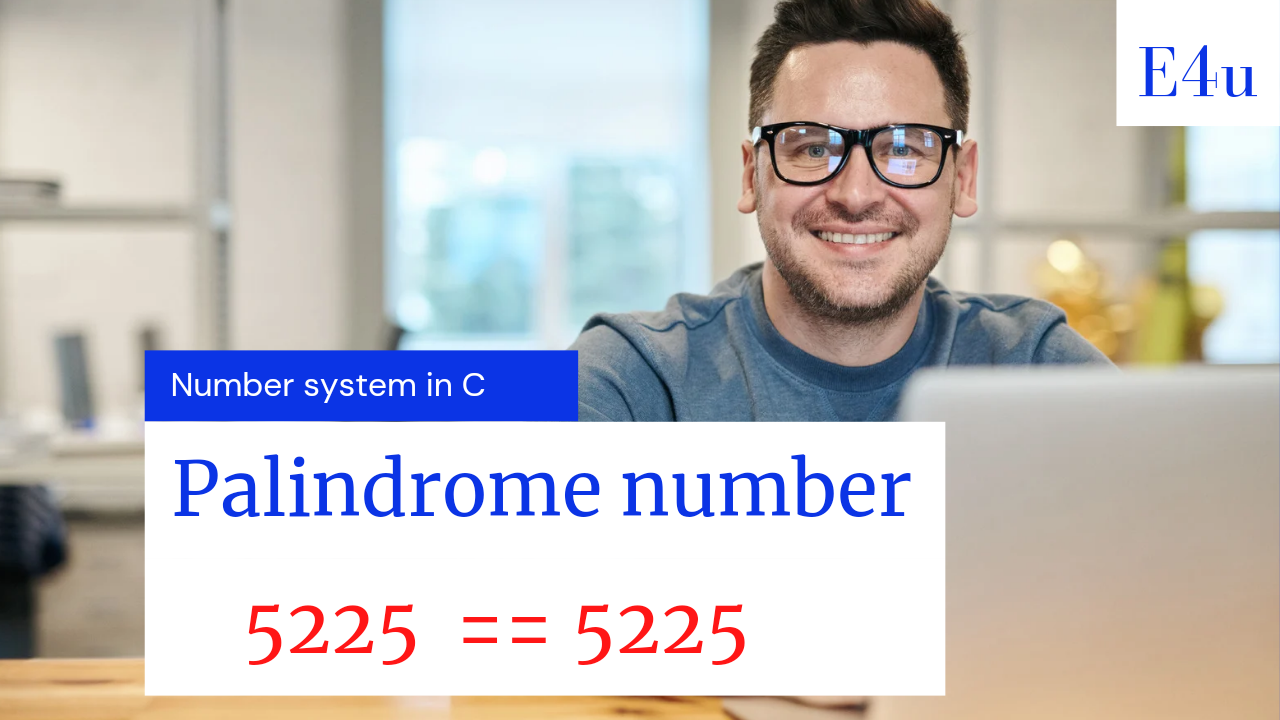
Palindrome number in c programming
Palindrome Numbers in C
I am discovering and Identifying Palindrome Numbers using C Programming Language.
What is a Palindrome Number?
A palindrome number is a number that remains the same when its digits are reversed.
For example, 121, 1331,
and 4444
are all palindrome numbers.
How to Check for Palindrome Numbers in C:
Using a Loop:
- Iterate through the digits of the number.
- Compare the original number with its reverse.
- If they match, the number is a palindrome.
Using Recursion:
- Define a recursive function to reverse the number.
- Compare the original number with its reverse.
- If they match, the number is a palindrome.
Palindrome Check using a Loop
// C program to check if a number is palindrome
#include
int main() {
int num, reversedNum = 0, remainder, originalNum;
// Input from user
printf("Enter an integer: ");
scanf("%d", &num);
originalNum = num;
// Reversing the number
while (num != 0) {
remainder = num % 10;
reversedNum = reversedNum * 10 + remainder;
num /= 10;
}
// Checking if the number is palindrome
if (originalNum == reversedNum)
printf("%d is a palindrome.\n", originalNum);
else
printf("%d is not a palindrome.\n", originalNum);
return 0;
}
Here is an example of how to use the program:
Enter an integer: 121
121 is a palindrome.
Here is an example of how to use the program to check if a number is not a palindrome:
Enter an integer: 123
123 is not a palindrome.
Palindrome Check using Recursion
// C program to check if a number is palindrome using recursion
#include
int reverse(int num) {
static int reversedNum = 0;
if (num == 0)
return reversedNum;
reversedNum = reversedNum * 10 + num % 10;
return reverse(num / 10);
}
int main() {
int num, originalNum;
// Input from user
printf("Enter an integer: ");
scanf("%d", &num);
originalNum = num;
int reversedNum = reverse(num);
// Checking if the number is palindrome
if (originalNum == reversedNum)
printf("%d is a palindrome.\n", originalNum);
else
printf("%d is not a palindrome.\n", originalNum);
return 0;
}
Output
Enter an integer: 121
121 is a palindrome.
Conclusion:
- Palindrome numbers are interesting mathematical concepts that have applications in various fields including number theory and computer science.
- By using C programming language, we can easily identify palindrome numbers through loops or recursion.
Further Reading:
Program to check whether the given string is a palindrome or not in c
write a program to print palindrome numbers in 10 array elements.
Palindrome number in c programming
Write a c program for 10 elements palindrome number
Design a function to check the given number is Palindrome or not if Palindrome return 1 else 0
Writing a C Program to Print Available Palindrome Numbers between 50 to 100 using a While Loop
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!