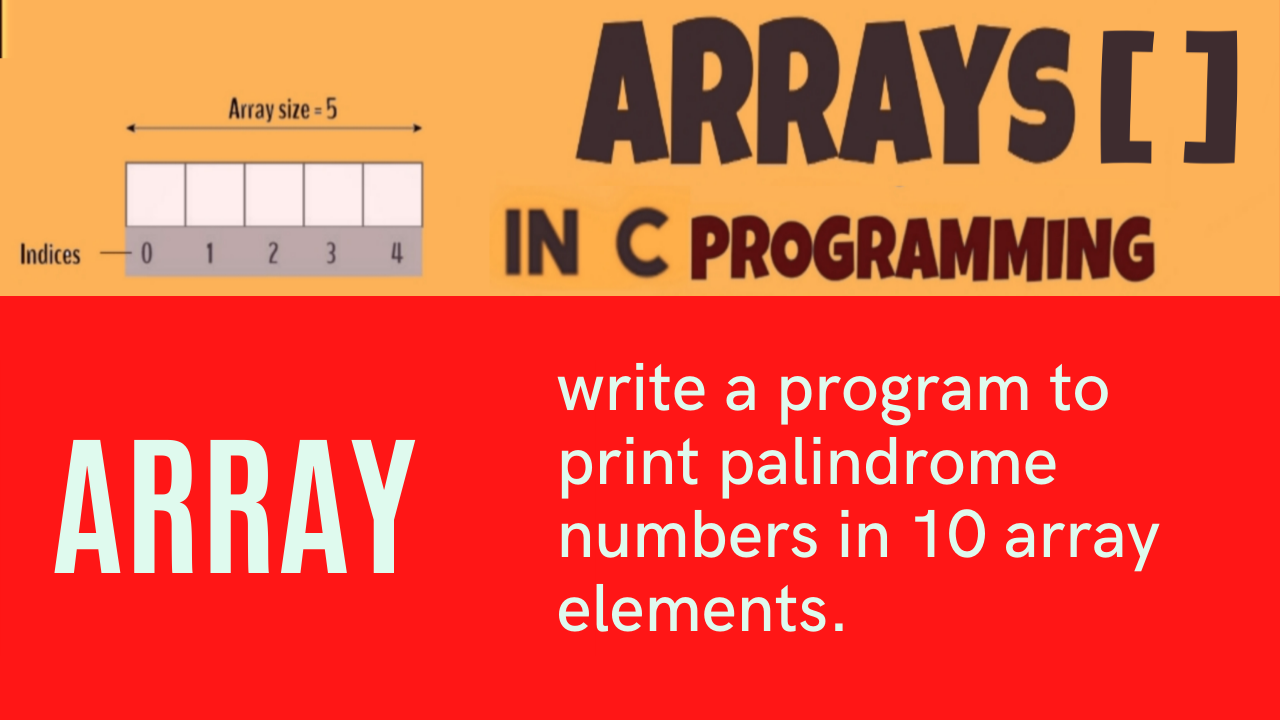
write a C Program to print palindrome numbers in 10 array elements.
C Program to Print Palindrome Numbers in an Array of 10 Elements
"Write a C program to print palindrome numbers in 10 array elements"
means that you need to write a computer program using the C programming language that checks 10 numbers stored in an array to see if they are palindromes.
A palindrome number is a number that remains the same when its digits are reversed. For example, 121, 1221
, and 12321
are palindrome numbers.
In this task, you are required to take input of 10 numbers from the user, store them in an array, and then check each number to see if it's a palindrome. If a number is a palindrome, it should be printed out.
The output of the program should only include palindrome numbers that are found among the 10 array elements.
C program that prints palindrome numbers in 10 array elements:
#include
int main() {
int arr[10];
int i, j, num, rem, rev;
printf("Enter 10 numbers: ");
for (i = 0; i < 10; i++) {
scanf("%d", &arr[i]);
}
printf("Palindrome numbers in the array are: ");
for (i = 0; i < 10; i++) {
num = arr[i];
rev = 0;
// Reverse the number
while (num != 0) {
rem = num % 10;
rev = rev * 10 + rem;
num /= 10;
}
// Check if the number is palindrome
if (rev == arr[i]) {
printf("%d ", arr[i]);
}
}
return 0;
}
Attention: Here's how the program works:
- The user is asked to enter 10 numbers.
- The program checks each number to see if it's a palindrome.
- To check if a number is a palindrome, the program first reverses the number using a while loop.
- Then, it checks if the reversed number is equal to the original number. If they're equal, the number is a palindrome.
- If a palindrome is found, the program prints it out.
Note that this program assumes that the input consists of 10 integers. If the input has a different number of integers, the behavior of the program is undefined.
Top Resources
write a program to print palindrome numbers in 10 array elements.
Writing a C Program to Print Available Palindrome Numbers between 50 to 100 using a While Loop
write a program to print perfect numbers in 10 array elements.
Write a program to find out how many angstrom number in 10 elements of an array and count it.
Write a program to find out how many prime number are present in a given 10 elements of an array
Print Prime Numbers Between 50 and 100 Using C programming language
Further Reading:
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!