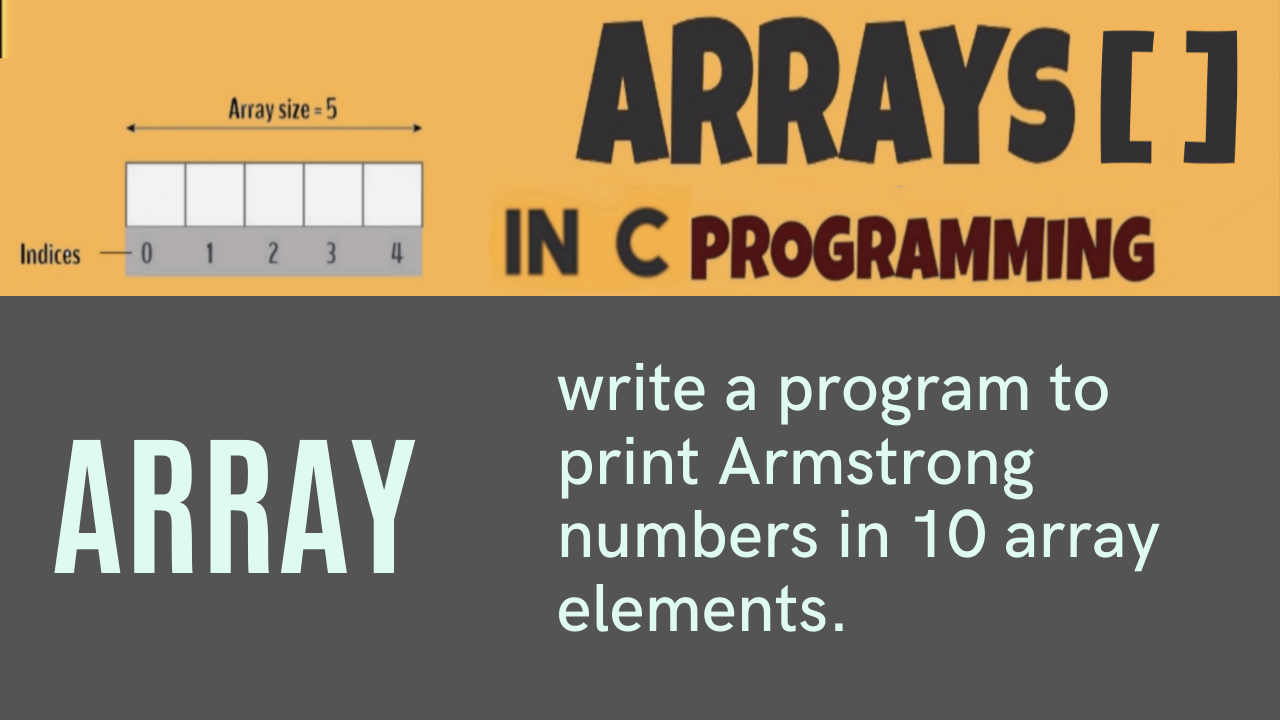
write a program to print Armstrong numbers in 10 array elements.
what is Armstrong number
An Armstrong number (also known as a narcissistic number, plenary number, or a plus perfect number) is a number that is equal to the sum of its own digits, each raised to the power of the number of digits.
In other words, let's say we have an n-digit number 'abcd...z'. If it is an Armstrong number, it would satisfy the condition:
an+bn+cn+…+zn=abcd...z
Here, 'n' represents the number of digits in the number.
For example, let's consider the 3-digit number 153:
- 13+53+33=1+125+27=153
As you can see, 153 is an Armstrong number because it is equal to the sum of the cubes of its digits.
Armstrong numbers are interesting mathematical curiosities and are often used as exercises in programming to demonstrate loops, conditionals, and arithmetic operations. They are named after Michael F. Armstrong, who is credited with discovering them.
C program that generates and prints Armstrong numbers within an array of 10 elements:
#include <stdio.h>
#include <math.h>
int isArmstrong(int num) {
int originalNum, remainder, n = 0;
originalNum = num;
// Count the number of digits
while (originalNum != 0) {
originalNum /= 10;
++n;
}
originalNum = num;
int result = 0;
// Check if it's an Armstrong number
while (originalNum != 0) {
remainder = originalNum % 10;
result += pow(remainder, n);
originalNum /= 10;
}
return result == num;
}
int main() {
int armstrongNumbers[10];
int count = 0;
int num = 0;
while (count < 10) {
if (isArmstrong(num)) {
armstrongNumbers[count] = num;
++count;
}
++num;
}
printf("The first 10 Armstrong numbers are:\n");
for (int i = 0; i < 10; i++) {
printf("%d ", armstrongNumbers[i]);
}
return 0;
}
This program defines a function isArmstrong to check if a number is an Armstrong number. It then generates and stores the first 10 Armstrong numbers in the armstrongNumbers array. Finally, it prints those numbers. Keep in mind that Armstrong numbers can get quite large, so be cautious with larger ranges.