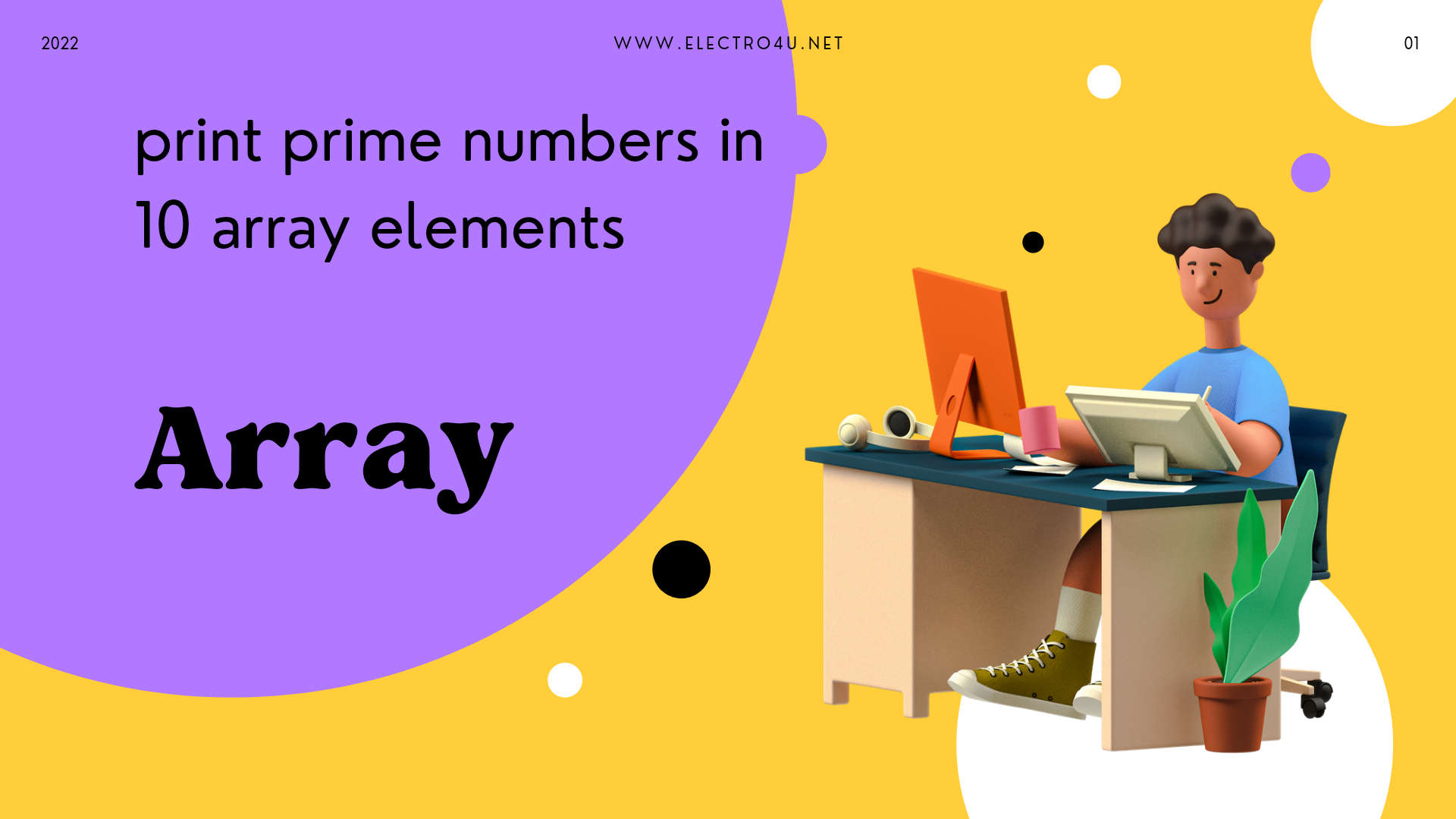
write a program to print prime numbers in 10 array elements in c++
Program in C to Print Prime Numbers in an Array of 10 Elements
"Write a program to print prime numbers in 10 array elements in cpp"
means creating a computer program using the C programming language that will take 10
integers as input from the user and store them in an array. The program should then identify and print out any prime numbers that are present in the array.
A prime number is a positive integer greater than 1 that has no positive integer divisors other than 1
and itself. For example, 2, 3, 5, 7, 11,
and 13
are prime numbers, but 4, 6, 8, 9,
and 10
are not.
The program should use a function to check if each number in the array is prime or not, and then print out the prime numbers found in the array to the console. If no prime numbers are found, the program should output a message indicating this.
C++ program to print prime numbers in 10 array elements:
#include <iostream>
using namespace std;
bool is_prime(int num) {
if (num <= 1) {
return false;
}
for (int i = 2; i <= num / 2; i++) {
if (num % i == 0) {
return false;
}
}
return true;
}
int main() {
int arr[10];
cout << "Enter 10 numbers: ";
for (int i = 0; i < 10; i++) {
cin >> arr[i];
}
cout << "Prime numbers in the array: ";
for (int i = 0; i < 10; i++) {
if (is_prime(arr[i])) {
cout << arr[i] << " ";
}
}
cout << endl;
return 0;
}
This program works by first prompting the user to enter 10 numbers into an array. Then, it iterates through the array and checks if each number is prime using the is_prime() function. If a number is prime, the program prints it to the console. Finally, the program prints a message indicating that there are no prime numbers in the array if none are found.
Output
Enter 10 numbers: 1 2 3 4 5 6 7 8 9 10
Prime numbers in the array: 2 3 5 7
Top Resources
Prime Numbers: C Program Example and Explanation
Design a function to check the given number is prime or not if prime return 1 else 0
Write a C Program to Check if a Number is Prime or Not Using For Loop
Writing a C Program to Print Prime Numbers Between 50 and 100
write a program to print prime numbers in 10 array elements.
Write a C program to input 10 numbers through the keyword and find the number of prime counts it stores the into a separate array and display it.
Further Reading:
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!