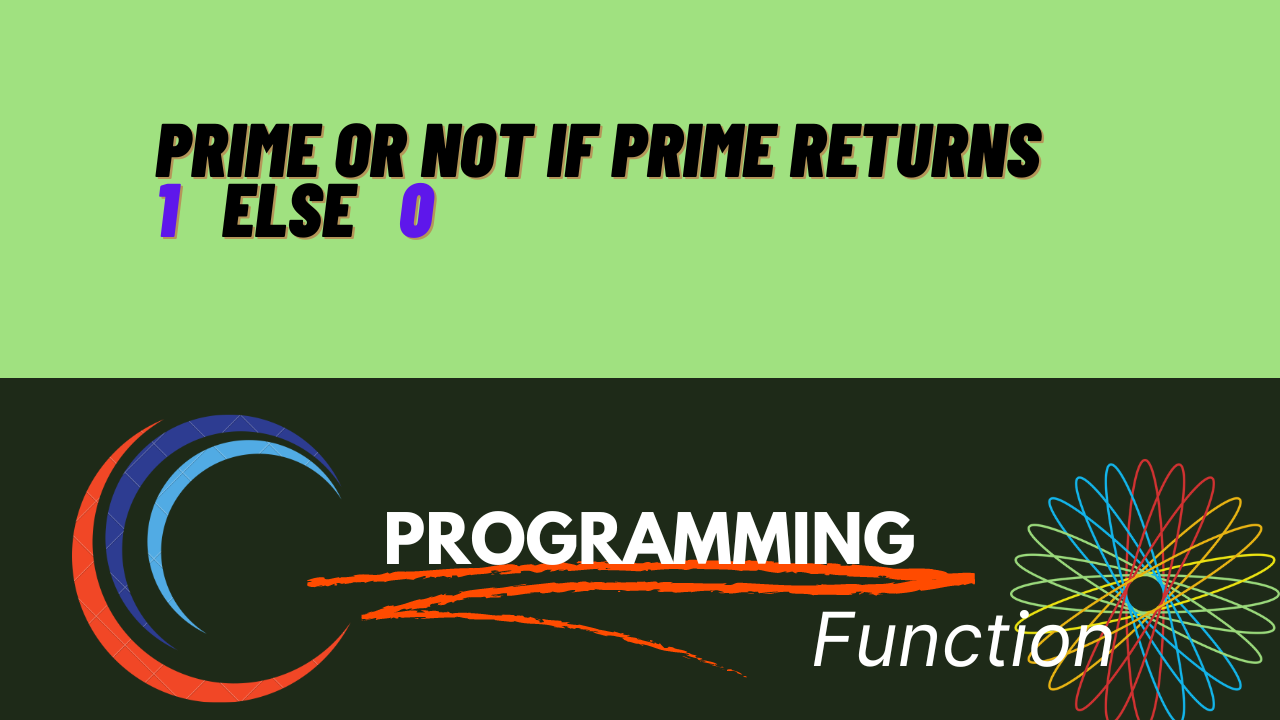
Design a function to check the given number is prime or not if prime return 1 else 0 in c
Design a Prime Number Checker Function in C
To design a function to check if a given number is prime or not and return 1
if prime else 0
in C, we can use the following algorithm:
- Take the number as input.
- Initialize a variable flag to
0.
- Iterate from
2
to the square root of the number. - If the number is divisible by any of the numbers in the loop, set flag to
1
and break the loop. - If the loop completes without setting flag to
1
, then the number is prime. - Return flag.
C code implements this algorithm:
C Programming
int is_prime(int n) {
int flag = 0;
for (int i = 2; i <= sqrt(n); i++) {
if (n % i == 0) {
flag = 1;
break;
}
}
return flag;
}
This function can be used as follows:
C Programming
int main() {
int n;
printf("Enter a number: ");
scanf("%d", &n);
int is_prime = is_prime(n);
if (is_prime) {
printf("%d is a prime number.\n", n);
} else {
printf("%d is not a prime number.\n", n);
}
return 0;
}
Example output:
Enter a number: 11
11 is a prime number.
Top Resources
Prime Numbers: C Program Example and Explanation
Design a function to check the given number is prime or not if prime return 1 else 0
Write a C Program to Check if a Number is Prime or Not Using For Loop
Writing a C Program to Print Prime Numbers Between 50 and 100
write a program to print prime numbers in 10 array elements.
Write a C program to input 10 numbers through the keyword and find the number of prime counts it stores the into a separate array and display it.
Further Reading:
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!