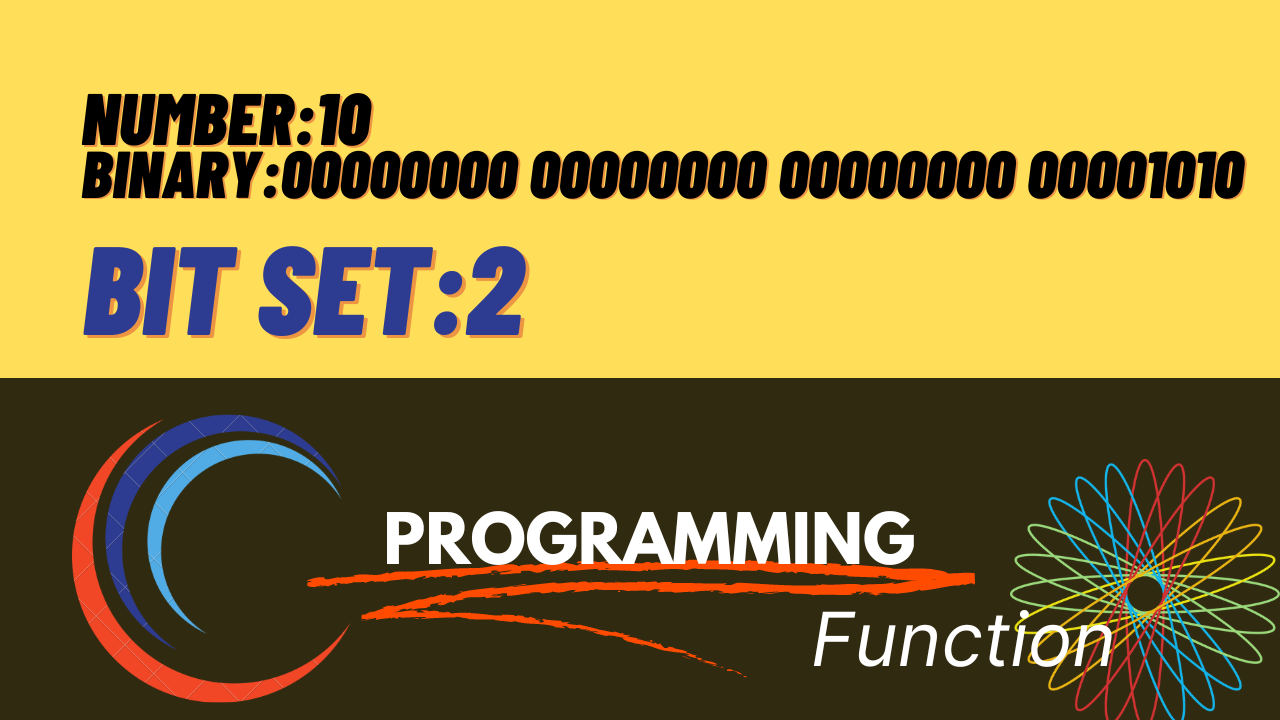
Design a function to count how many bits are set in a given integers in c
Count Set Bits in an Integer - C Programming
Counting the number of bits that are set in a given integer in C refers to the process of determining how many binary digits (bits) in the integer are equal to 1.
For example, if we have the integer value 42,
which has a binary representation of 101010,
counting the number of bits that are set means counting the number of 1's in the binary representation. In this case, there are three bits that are set.
The process of counting the number of bits that are set in an integer is a common problem in computer programming, and it has many applications in fields such as cryptography, image processing, and computer networks. One common approach to counting set bits in an integer is to use bitwise operators such as AND, OR
, and XOR,
along with bit shifting and masking operations. Another approach is to use lookup tables or pre-calculated bit patterns to count set bits in a single operation.
In C programming, counting set bits in an integer can be implemented using a function that takes an integer as an argument and returns the count of set bits. The function typically uses a loop to iterate over each bit in the integer, check if it is set, and increment a count variable accordingly. The result of the function is the total count of set bits in the integer
Design a function to count how many bits are set in a given integer in c
#include<stdio.h>
void print_binary(int n);
int count_bit_set(int);
void main()
{
int num,c;
printf("Enter the Number:");
scanf("%d",&num);
print_binary(num);
printf("\n");
c=count_bit_set(num);
printf("c=%d\n",c);
}
void print_binary(int n)
{
int pos;
for(pos=31;pos>=0;pos--)
{
printf("%d",n>>pos&1);
}
}
int count_bit_set(int n)
{
int c,pos;
for(pos=0,c=0;pos<=31;pos++)
{
if(n>>pos&1)
c++;
}
return c;
}
Output:
Enter the Number: 10
00000000000000000000000000001010
c=2
Further Reading:
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them