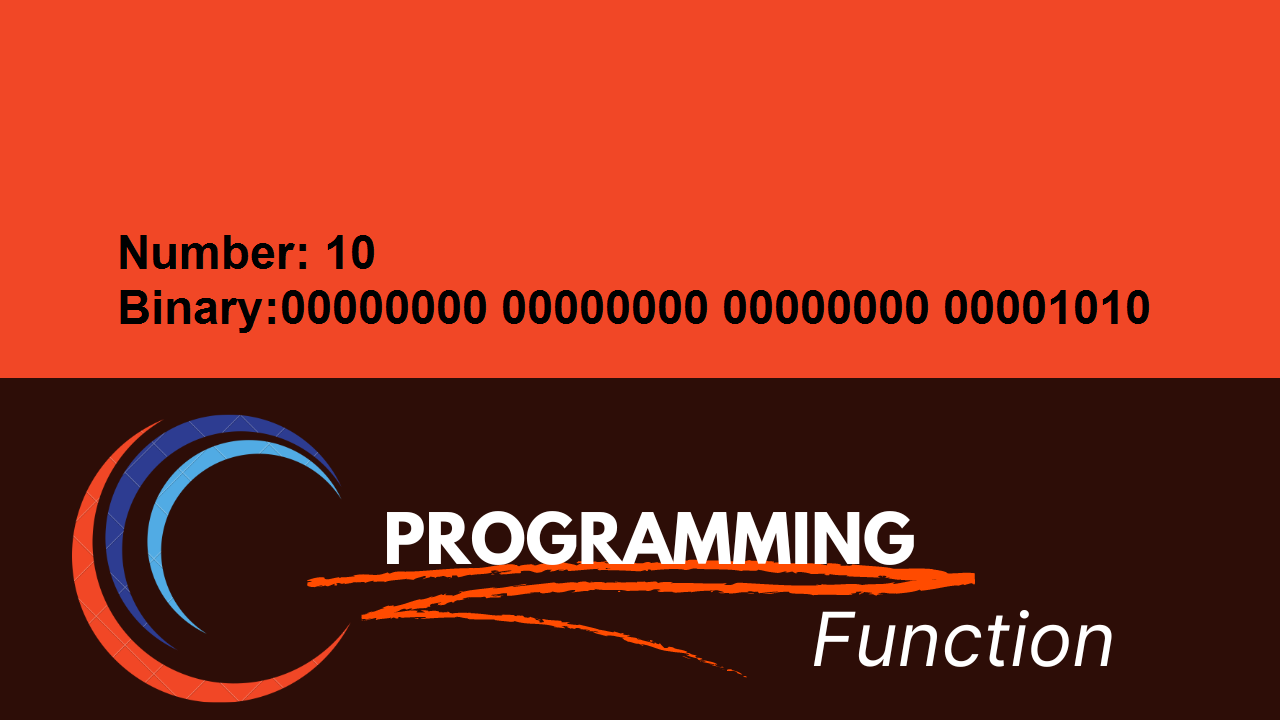
Design a function to print the number into binary formate in c | electro4u
Designing a Binary Number Printing Function in C
Objective:
Create a function in C to convert a given number into its binary representation.
Steps:
Function Declaration:
-
- Begin by declaring the function prototype.
void printBinary(int num);
Function Definition:
-
- Define the function. Use bitwise operations to extract individual bits.
void printBinary(int num) { int i; for(i = sizeof(int) * 8 - 1; i >= 0; i--) { printf("%d", (num & (1 << i)) ? 1 : 0); } }
Explanation:
-
- sizeof(int) * 8 determines the number of bits in an integer.
- The loop iterates through each bit, starting from the leftmost.
- (num & (1 << i)) checks if the i-th bit is set.
- If set, it prints 1, otherwise 0.
Example Usage:
int main() {
int num = 10; // Example number
printBinary(num);
return 0;
}
Output:
00000000000000000000001010
Design a function to print the number into binary format in c
#include<stdio.h>
void print_binary(int);
void main()
{
int num;
printf("enter the number:");
scanf("%d",&num);
print_binary(num);
printf("\n");
}
void print_binary(int n)
{
int pos;
for(pos=31;pos>=0;pos--)
{
printf("%d",n>>pos&1);
}
}
Output:
Enter the number: 10
00000000000000000000000000001010
Conclusion:
You now have a function, printBinary, that can convert an integer into its binary representation. This function can be utilized in your C programs to perform binary operations.
Further Reading:
[Design a function to print the number into binary formate in c]
[Writing a c program to print the binary format of float using character format]
[Write a program to print the binary formate of float using integer pointer in c]
[Write a program to print Binary number using for loop]
[printing the Binary Format of any Floating number using union]
[printing the binary of any integer number using union in c | electro4u]
Further Reading:
For further information and examples, Please visit[ C-Programming From Scratch to Advanced 2023-2024]
Top Resources
- Learn-C.org
- C Programming at LearnCpp.com
- GeeksforGeeks - C Programming Language
- C Programming on Tutorialspoint
- Codecademy - Learn C
- CProgramming.com
- C Programming Wikibook
- C Programming - Reddit Community
- C Programming Language - Official Documentation
- GitHub - Awesome C
- HackerRank - C Language
- LeetCode - C
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!