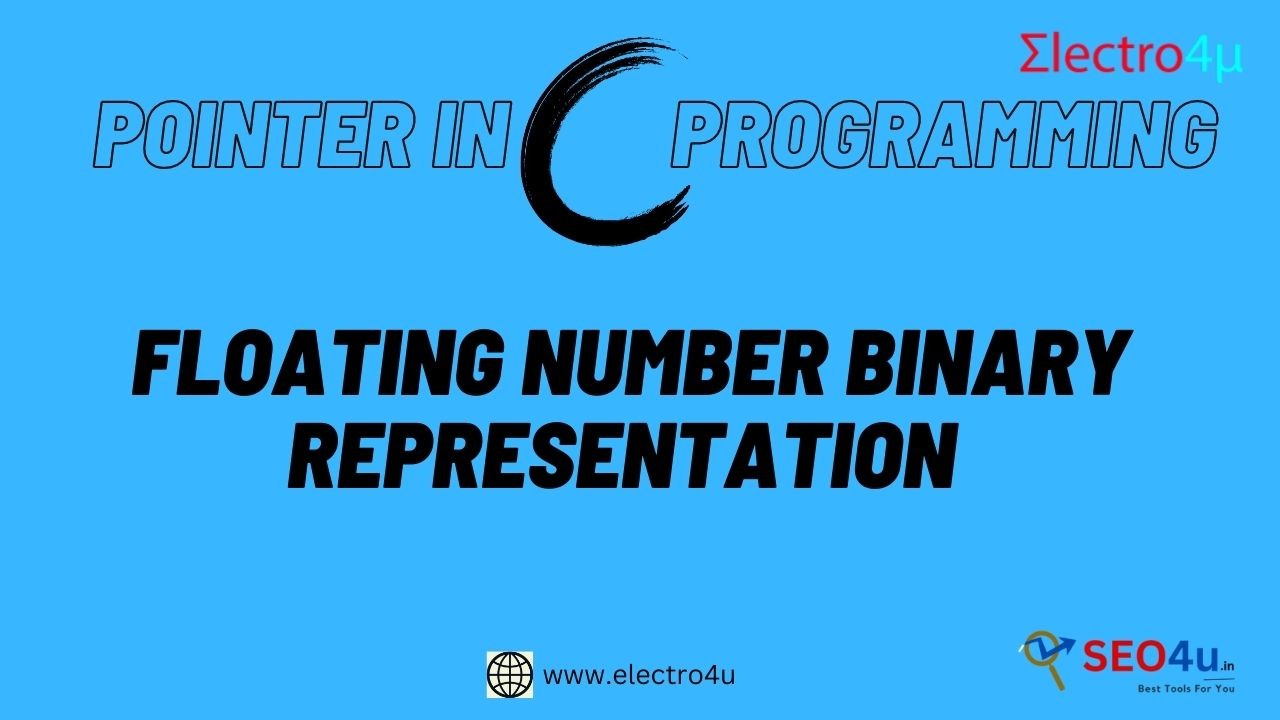
printing the Binary Format of any Floating number using union
Printing the Binary Format of any Floating Number using Union in C
Introduction:
- Learn how to convert a floating-point number into its binary representation using the Union data structure in the C programming language.
Step 1: Define a Union:
- Begin by defining a union that contains a floating-point variable and an integer variable of the same size.
union FloatUnion {
float f;
int i;
};
Step 2: Convert the Floating-Point Number:
- Assign a floating-point number to the f member of the union.
union FloatUnion data;
data.f = 3.14; // Example: Assigning a floating-point number
Step 3: Access the Binary Representation:
- Access the binary representation of the floating-point number through the integer member.
int binaryRepresentation = data.i;
Step 4: Print the Binary Format:
- Utilize bitwise operations to extract individual bits and print the binary format.
for(int i = sizeof(int)*8 - 1; i >= 0; i--) {
printf("%d", (binaryRepresentation >> i) & 1);
}
Example Output:
- If the floating-point number is 3.14, the binary representation would be printed as 01000000010010001111010111000011.
Program 01: How to Print the Binary Format of a Floating Point Number Using Union in C
#include
union u
{
int i;
float f;
};
void main()
{
union u u1;
int pos;
printf("enter any floating number:\n");
scanf("%f",&u1.f);
// u1.f=20.5;
for(pos=31;pos>=0;pos--)
printf("%d",u1.i>>pos&1);
printf("\n");
}
output:
enter any floating number: 20.30
01000001101000100110011001100110
Attention: The program defines a union called u that contains two members: an integer i and a float f. Since the integer and float members of the union occupy the same memory location, writing to one member and then reading from the other can result in unpredictable behavior.
The main() function declares an instance of the union called u1 and an integer called pos. The program then prompts the user to enter a floating-point number using the printf() and scanf() functions provided by the standard input/output library. The user's input is stored in the f member of the union using the scanf() function.
The program then enters a for loop that iterates over each bit of the integer representation of the floating-point number. The loop uses bitwise operations to extract each bit from the integer and print it to the screen. Specifically, it shifts the integer right by pos bits using the >> operator and then performs a bitwise AND with the value 1 to extract the least significant bit. The resulting bit value is printed to the screen using the printf() function.
Finally, the program prints a newline character to the screen using the printf() function to end the output.
Conclusion:
- You've successfully learned how to print the binary format of a floating-point number using a union in C. This technique is useful for various applications, including low-level manipulation of data.
Further Reading:
[Design a function to print the number into binary formate in c]
[Writing a c program to print the binary format of float using character format]
[Write a program to print the binary formate of float using integer pointer in c]
[Write a program to print Binary number using for loop]
[printing the Binary Format of any Floating number using union]
[printing the binary of any integer number using union in c | electro4u]
Top Resources
- Learn-C.org
- C Programming at LearnCpp.com
- GeeksforGeeks - C Programming Language
- C Programming on Tutorialspoint
- Codecademy - Learn C
- CProgramming.com
- C Programming Wikibook
- C Programming - Reddit Community
- C Programming Language - Official Documentation
- GitHub - Awesome C
- HackerRank - C Language
- LeetCode - C
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [[email protected]].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!