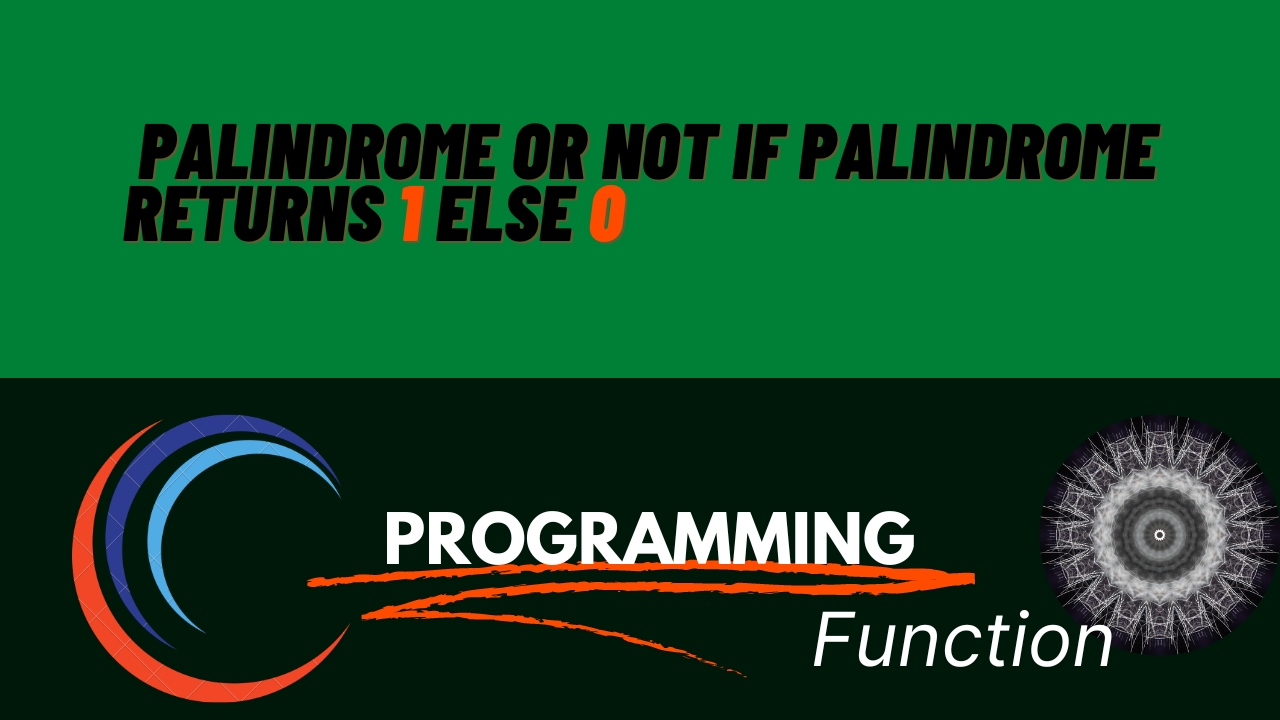
C Function to Check Number is Palindrome or not
Check if a Number is Palindrome
To design a function to check if a given number is a palindrome in C, we can use the following algorithm:
- Reverse the given number.
- Compare the reversed number with the original number.
- If the reversed number is equal to the original number, then the number is a palindrome.
- Otherwise, the number is not a palindrome.
C Function implementation of the above algorithm:
C Programming
int isPalindrome(int number) {
int reversedNumber = 0;
int temp = number;
while (temp > 0) {
int remainder = temp % 10;
reversedNumber = reversedNumber * 10 + remainder;
temp /= 10;
}
if (reversedNumber == number) {
return
1;
} else {
return
0;
}
}
This function takes an integer number as input and returns 1 if the number is a palindrome, and 0 otherwise.
How to use the isPalindrome() function:
C Programming
int main()
{
int number;
printf("Enter a number: ");
scanf("%d", &number);
int isPalindromeResult = isPalindrome(number);
if (isPalindromeResult == 1) {
printf("%d is a palindrome.\n", number);
} else {
printf("%d is not a palindrome.\n", number);
}
return
0;
}
Output:
Enter a number: 121
121 is a palindrome.
Further Reading:
Program to check whether the given string is a palindrome or not in c
write a program to print palindrome numbers in 10 array elements.
Palindrome number in c programming
Write a c program for 10 elements palindrome number
Design a function to check the given number is Palindrome or not if Palindrome return 1 else 0
Writing a C Program to Print Available Palindrome Numbers between 50 to 100 using a While Loop
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!