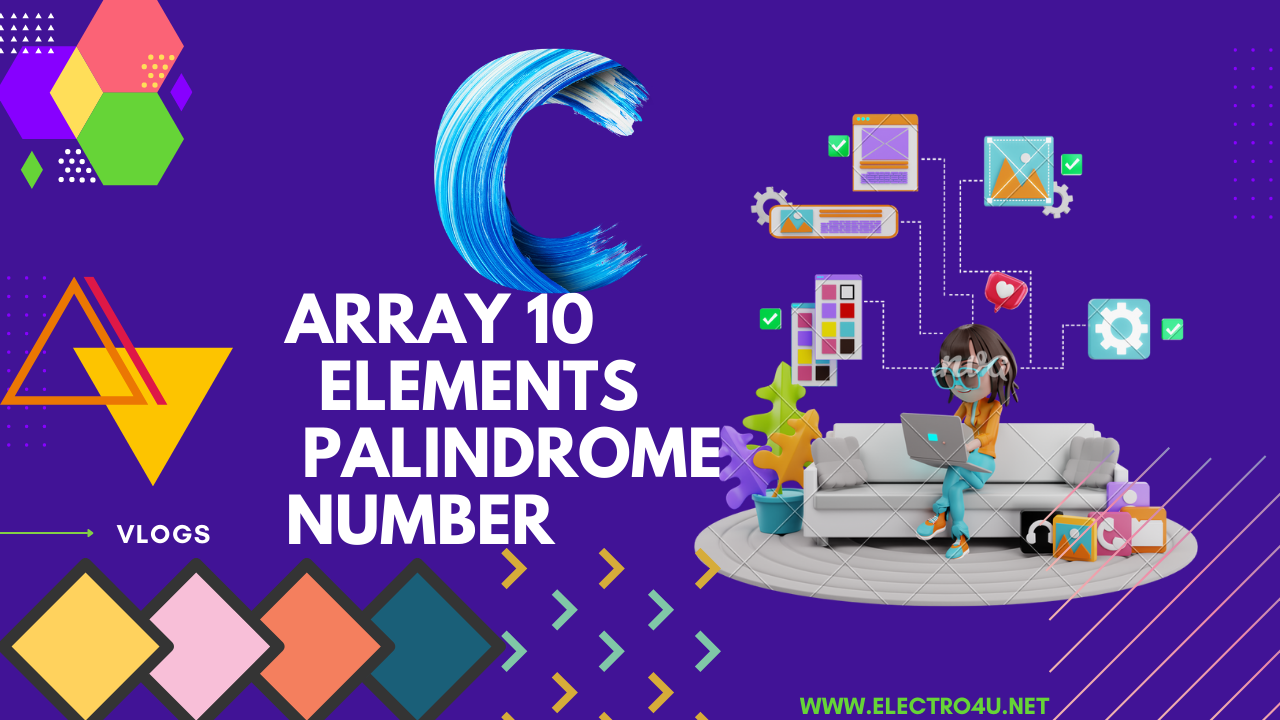
Write a c program for 10 elements palindrome number
C Program to Find 10 Palindrome Numbers - Source Code and Explanation
A palindrome number is a number that remains the same when its digits are reversed. In other words, a number is a palindrome number if it reads the same from left to right and from right to left.
For example, 121
is a palindrome number because it remains the same when its digits are reversed: 121.
To write a C program for 10 elements palindrome number, we can use the following steps:
- Declare an array to store the 10 elements.
- Prompt the user to enter the 10 elements.
- Check if each element of the array is equal to the corresponding element in the reverse order.
- If all the elements of the array are equal to the corresponding elements in the reverse order, then the array is a palindrome. Otherwise, the array is not a palindrome.
C program for 10 elements palindrome number:
C program
#include <stdio.h>
int main() {
int arr[10];
int i, is_palindrome = 1;
// Prompt the user to enter the 10 elements.
printf("Enter 10 elements: ");
for (i = 0; i < 10; i++) {
scanf("%d", &arr[i]);
}
// Check if each element of the array is equal to the corresponding element in the reverse order.
for (i = 0; i < 5; i++) {
if (arr[i] != arr[9 - i]) {
is_palindrome = 0;
break;
}
}
// Print the result.
if (is_palindrome) {
printf("The array is a palindrome.\n");
} else {
printf("The array is not a palindrome.\n");
}
return 0;
}
output:
Enter 10 elements: 1 2 3 4 5 5 4 3 2 1
The array is a palindrome
Top Resources
write a program to print palindrome numbers in 10 array elements.
Writing a C Program to Print Available Palindrome Numbers between 50 to 100 using a While Loop
write a program to print perfect numbers in 10 array elements.
Write a program to find out how many angstrom number in 10 elements of an array and count it.
Write a program to find out how many prime number are present in a given 10 elements of an array
Print Prime Numbers Between 50 and 100 Using C programming language
Further Reading:
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!