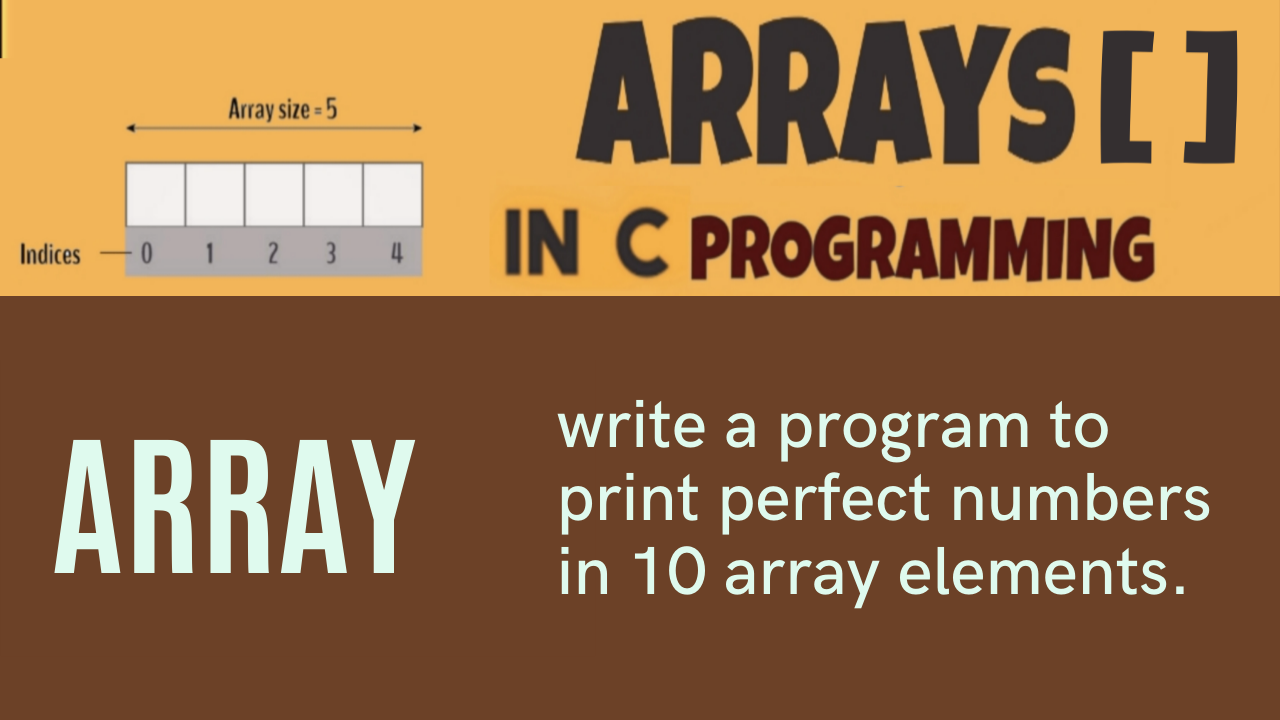
write a program to print perfect numbers in 10 array elements.
A perfect number is a positive integer that is equal to the sum of its proper divisors (that is, all divisors of the number except for the number itself).
For example, 6 is a perfect number because its proper divisors are 1, 2, and 3, and:
1 + 2 + 3 = 6 Click here to more
print perfect numbers in 10 array elements in c.
Here's an example program in C to find perfect numbers in an array of 10 elements:
#include <stdio.h>
int is_perfect(int num) {
int sum = 0;
for (int i = 1; i < num; i++) {
if (num % i == 0) {
sum += i;
}
}
return (sum == num);
}
int main() {
int arr[] = {6, 28, 496, 8128, 33550336, 10, 30, 100, 200, 500};
int n = 10;
for (int i = 0; i < n; i++) {
if (is_perfect(arr[i])) {
printf("%d is a perfect number\n", arr[i]);
}
}
return 0;
}
In this program, we define a helper function is_perfect that checks if a number is a perfect number by summing its proper divisors (that is, all divisors of the number except for the number itself) and comparing the sum to the original number. We then define the main function that initializes an array of 10 elements, iterates over each element, and prints a message if the element is a perfect number.