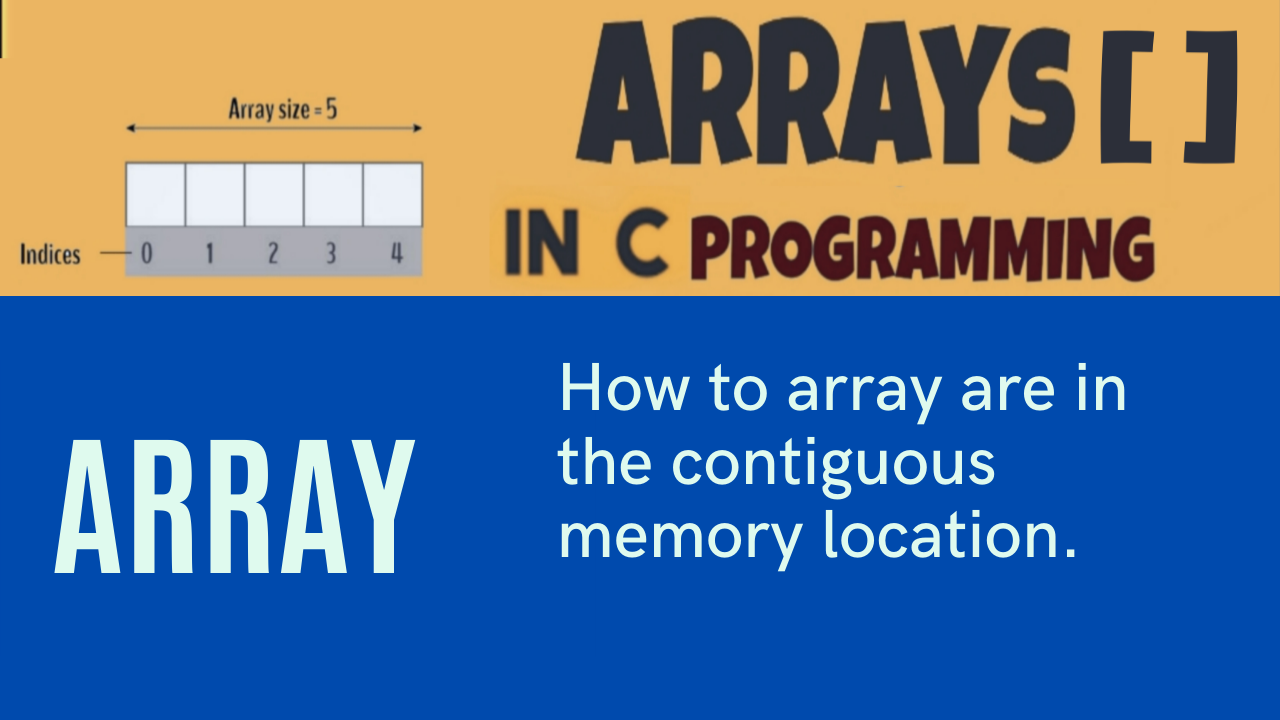
How to array are in the contiguous memory location in c.
How Arrays Are Stored in Contiguous Memory Location in C
Arrays are stored in contiguous memory locations in C. This means that the elements of an array are stored next to each other in memory. This is because arrays are implemented as a block of memory that is allocated when the array is declared.
The following diagram shows how an array is stored in memory:
Address | Value
------- | --------
00000000 | 10
00000004 | 20
00000008 | 30
0000000C | 40
In this example, the array is declared as follows:
int arr[4] = {10, 20, 30, 40};
The array is stored at the address 00000000.
The first element of the array is stored at address 00000000
, the second element is stored at address 00000004,
and so on.
The contiguous storage of arrays makes them very efficient for accessing and manipulating data. For example, to access the third element of the array, we simply need to calculate the address of the third element, which is 00000000 + 8 = 00000008
. We can then use this address to access the element using a pointer.
The contiguous storage of arrays also makes them very efficient for caching data. When the CPU accesses an element of an array, it will typically cache the entire array in memory. This means that subsequent accesses to the array will be very fast.
Program that demonstrates array are in contiguous memory location
#include<stdio.h>
int main() {
int arr[5] = {1, 2, 3, 4, 5};
printf("Address of arr[0]: %p\n", &arr[0]);
printf("Address of arr[1]: %p\n", &arr[1]);
printf("Address of arr[2]: %p\n", &arr[2]);
printf("Address of arr[3]: %p\n", &arr[3]);
printf("Address of arr[4]: %p\n", &arr[4]);
return 0;
}
Here's how the program works:
- The program declares an integer array arr of size 5 and initializes it with some values.
- The program then prints out the memory addresses of each element in the array using the & operator.
- The memory addresses printed out should be contiguous since arrays in C are stored in contiguous memory locations.
Note that the output of this program may vary on different systems, but the addresses should be contiguous regardless.
Top Resources
Difference between arrays and pointers?
What is the difference between strings and character arrays?
Further Reading:
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!