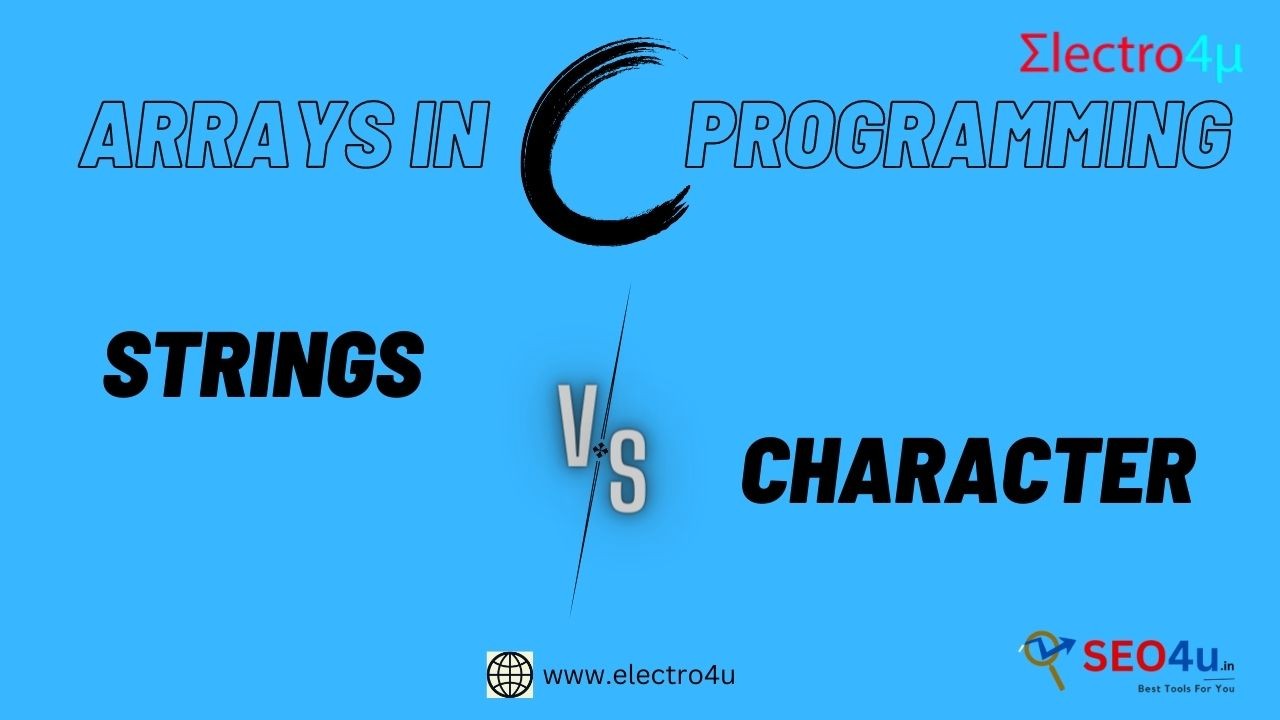
What is the difference between strings and character arrays?
Strings vs. Character Arrays in C: Key Differences
Strings and character arrays are both data types that store sequences of characters in C. However, there are some key differences between the two.
Strings are immutable sequences of characters. This means that they cannot be changed once they are created. Strings are created using single quotes or double quotes. For example, the following code creates a string:
char* my_string = "This is a string.";
Character arrays are mutable sequences of characters. This means that they can be changed once they are created. Character arrays are created using square brackets. For example, the following code creates a character array:
char my_character_array[] = {'T', 'h', 'i', 's', ' ', 'i', 's', ' ', 'a', ' ', 'c', 'h', 'a', 'r', 'a', 'c', 't', 'e', 'r', '.'};
Another key difference between strings and character arrays is that strings are terminated by a null character (\0). This means that the compiler knows where the string ends, even if the array that stores the string is larger than the number of characters in the string. Character arrays, on the other hand, are not terminated by a null character. This means that it is important to keep track of the length of the character array to avoid accessing characters outside of the array.
Key differences between strings and character arrays:
Feature | String | Character array |
---|---|---|
Mutability | Immutable | Mutable |
Termination | Terminated by a null character (\0) | Not terminated by a null character (\0) |
Creation | Created using single quotes or double quotes | Created using square brackets |
int main() {
char* my_string = "This is a string.";
char my_character_array[] = {'T', 'h', 'i', 's', ' ', 'i', 's', ' ', 'a', ' ', 'c', 'h', 'a', 'r', 'a', 'c', 't', 'e', 'r', '.'};
// Accessing a character in a string
char first_character_of_string = my_string[0]; // Outputs 'T'
// Accessing a character in a character array
char first_character_of_array = my_character_array[0]; // Outputs 'T'
// Trying to change a character in a string
my_string[0] = 'H'; // This will result in an error
// Changing a character in a character array
my_character_array[0] = 'H'; // This is allowed
return 0;
}
Which one to use?
Strings are generally easier to use than character arrays, because they are immutable and terminated by a null character. However, character arrays can be more efficient in some cases, because they can be allocated on the stack and they do not require the compiler to add a null character to the end of the array.
It is important to choose the correct data type for your needs. If you need to store a sequence of characters that cannot be changed, then you should use a string. If you need to store a sequence of characters that can be changed, or if you need to be more efficient with memory usage, then you should use a character array.