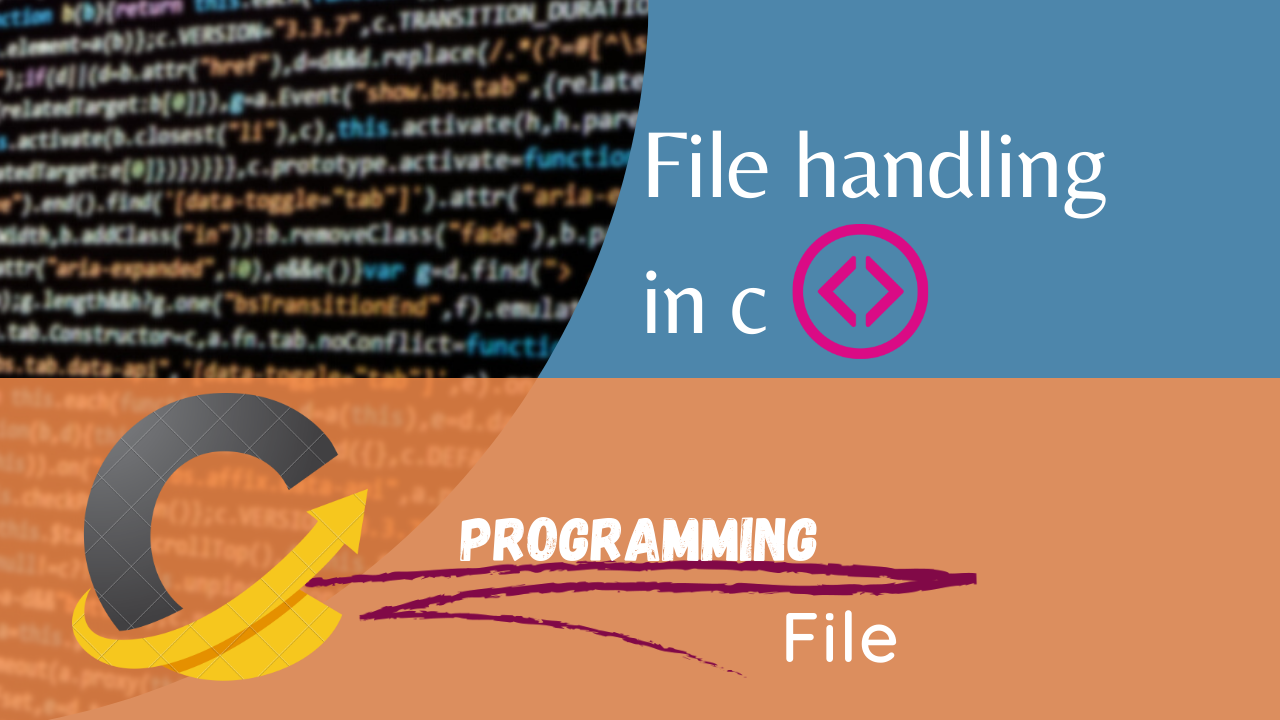
File Handling in C: A Comprehensive Guide with electro4u
File Handling in C
File handling in C allows programs to read and write data to files. This is essential for tasks like saving user preferences, processing large datasets, and more.
File Operations
Opening a File
FILE *fopen(const char *filename, const char *mode);
- fopen is used to open a file.
- filename is the name of the file.
- mode specifies how the file will be opened (read, write, etc.).
Closing a File
int fclose(FILE *stream);
- fclose is used to close a file after you're done using it.
Reading from a File
int fscanf(FILE *stream, const char *format, ...);
- fscanf reads formatted data from a file.
char *fgets(char *str, int n, FILE *stream);
- fgets reads a line from a file.
Writing to a File
int fprintf(FILE *stream, const char *format, ...);
- fprintf writes formatted data to a file.
int fputs(const char *str, FILE *stream);
- fputs writes a string to a file.
File Pointers
- FILE* is a pointer to a FILE structure which contains information about the file.
Checking for Errors
int ferror(FILE *stream);
- ferror checks if an error has occurred on a stream.
void clearerr(FILE *stream);
- clearerr clears the end-of-file and error indicators.
File Positioning
int fseek(FILE *stream, long int offset, int whence);
- fseek moves the file pointer to a specified location.
long int ftell(FILE *stream);
- ftell returns the current position of the file pointer.
Error Handling
- Always check if file operations were successful (fopen, fclose, etc.).
- Use ferror to check for errors.
Example
FILE *fp = fopen("myfile.txt", "w");
if (fp != NULL) {
fprintf(fp, "Hello, World!\n");
fclose(fp);
} else {
printf("Error opening file.\n");
}
Conclusion
- Understanding file handling is essential for working with external data sources in C.
- Proper handling ensures data integrity and program robustness.
Further Reading:
For further information and examples, Please visit[ C-Programming From Scratch to Advanced 2023-2024]
Top Resources
- Learn-C.org
- C Programming at LearnCpp.com
- GeeksforGeeks - C Programming Language
- C Programming on Tutorialspoint
- Codecademy - Learn C
- CProgramming.com
- C Programming Wikibook
- C Programming - Reddit Community
- C Programming Language - Official Documentation
- GitHub - Awesome C
- HackerRank - C Language
- LeetCode - C
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!