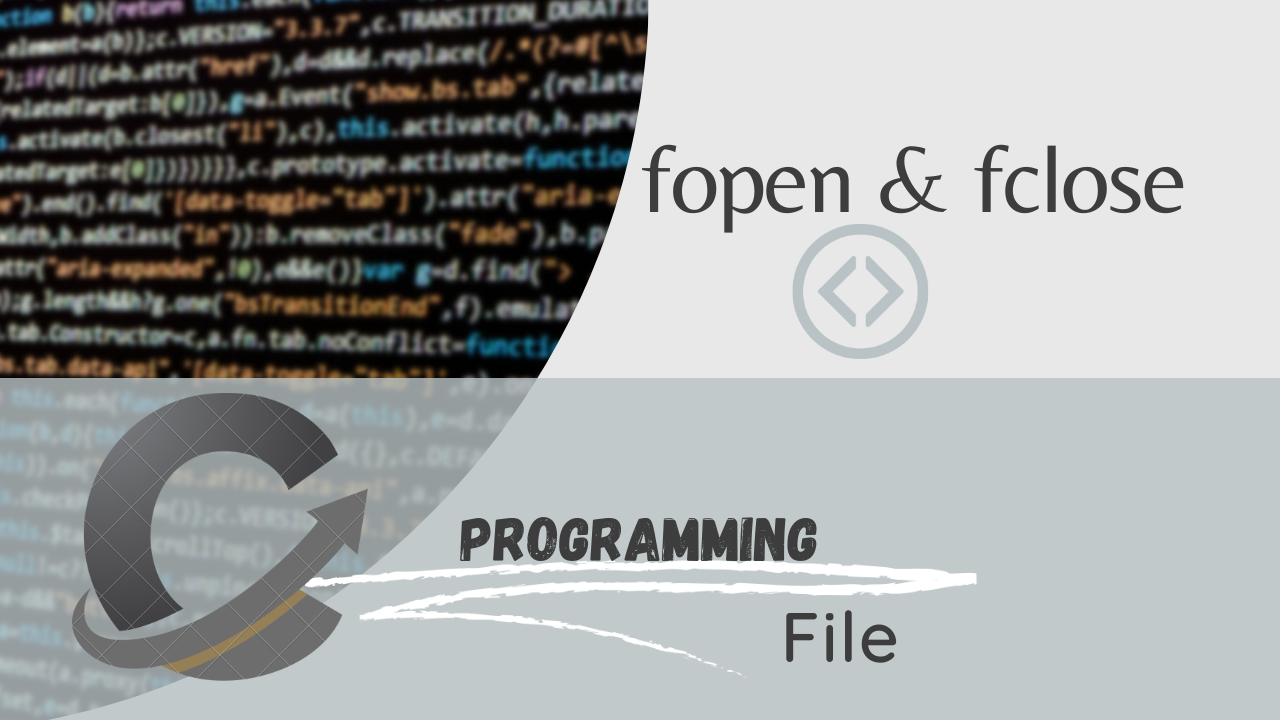
fopen and fclose function in c programming | electro4u
File Handling in C: fopen and fclose Functions
Introduction
File handling in C is an essential aspect of programming. It allows us to read from and write to files on our computer's storage. Two crucial functions in C for file handling are fopen and fclose.
fopen Function
The fopen function is used to open a file and obtain a file pointer to perform various operations such as reading, writing, etc.
fopen() function is used to open a file. It takes two arguments: the name of the file and the mode in which the file is to be opened. The mode can be "r" (read), "w" (write), "a" (append), "r+" (read and write), "w+" (read and write, overwriting the file), or "a+" (read and write, appending to the file).
Syntax:
⇒upon success fopen( ) return file pointer.
⇒upon failure, it returns zeros.
- filename: A string representing the name of the file you want to open.
- mode: A string indicating the mode in which you want to open the file (e.g., "r" for read, "w" for write, "a" for append, etc.).
Example:
FILE* file_ptr;
file_ptr = fopen("example.txt", "w");
if (file_ptr == NULL) {
printf("File could not be opened.");
exit(1);
}
In this example, we try to open a file named "example.txt" in write mode. If the file does not exist, it will be created. If the file exists, its content will be truncated.
fclose Function
The fclose function is used to close an open file.
Syntax:
int fclose(FILE* stream);
- stream: A pointer to the FILE structure representing the file.
Example:
FILE* file_ptr;
file_ptr = fopen("example.txt", "w");
if (file_ptr == NULL) {
printf("File could not be opened.");
exit(1);
}
// Perform operations on the file
fclose(file_ptr);
In this example, we open the file "example.txt" for writing. After performing operations on the file, we close it using fclose.
Program: Write a c program of file using fclose() and fopen()
#include<stdio.h>
void main(int argc,char **argv)
{
FILE *fp;
if(argc!=2)
{
printf("usage:./a.out fname\n");
return;
}
fp=fopen(argv[1],"r");//load time
//fp=fopen("data","r");//run time
/*char s[20];
printf("Enter the file name\n");
scanf("%s",s)
fp=open(s,"r");
*/
if(fp==0)
{
printf("File not present ...\n");
return;
}
printf("file present ...\n");
}
Conclusion
Understanding fopen and fclose functions is crucial for effective file handling in C. These functions provide the foundation for reading from and writing to files, which is a common task in many C programs.
Further Reading:
Top Resources
- Learn-C.org
- C Programming at LearnCpp.com
- GeeksforGeeks - C Programming Language
- C Programming on Tutorialspoint
- Codecademy - Learn C
- CProgramming.com
- C Programming Wikibook
- C Programming - Reddit Community
- C Programming Language - Official Documentation
- GitHub - Awesome C
- HackerRank - C Language
- LeetCode - C
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug thm
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on [ electro4u_offical_ ] for updates and tips.