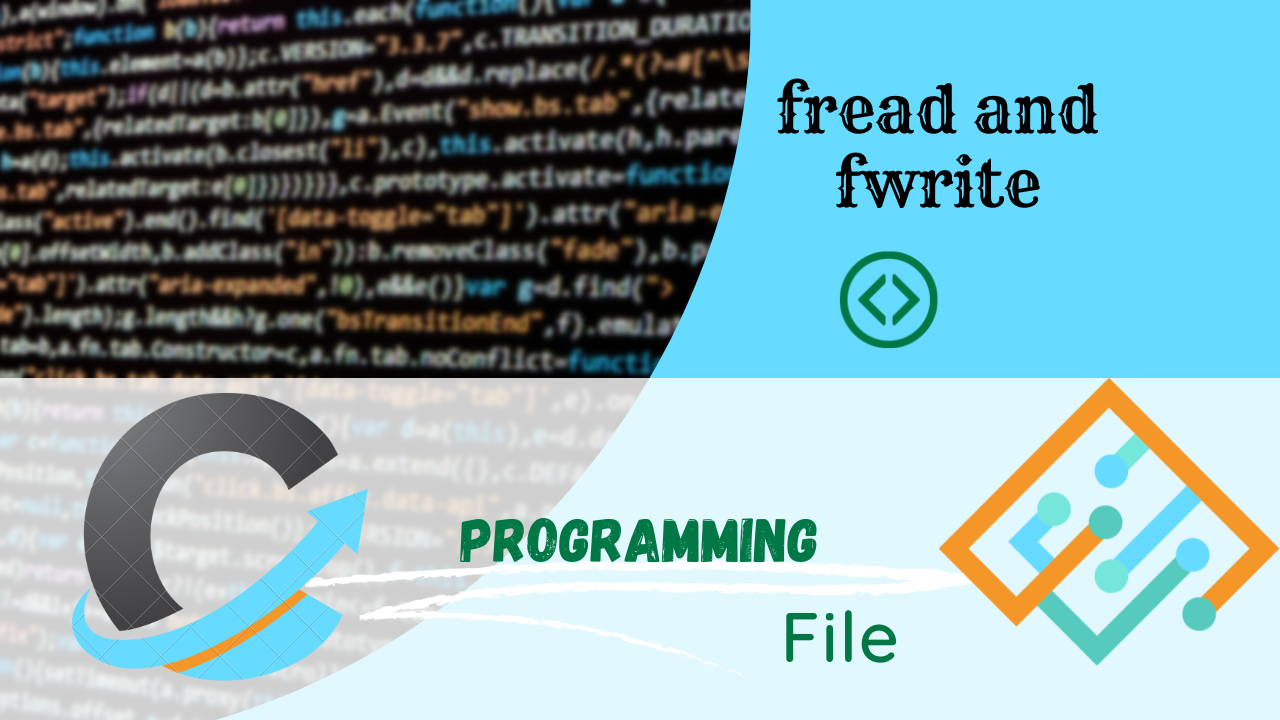
fread and fwrite in c
fread() and fwrite() in C
fread
and fwrite
: fread and fwite is binary stream functions (related text types)
. fread and fwrite are two file-handling functions in C programming that are used to read and write binary data to and from a file. Here's a brief explanation of each function:
What is Fread() function in c
The fread function is used to read binary data from a file. The function takes four arguments:
Syntax of fread
- ptr: A pointer to the memory location where the data will be stored.
- size: The size (in bytes) of each element to be read.
- count: The number of elements to be read.
- stream: A pointer to the file object to be read from.
The function returns the number of elements successfully read.
What is fwrite() function in c
The fwrite() function takes the same arguments as the fread() function, but it writes data to the file stream instead of reading it.
syntax of fwrite
- ptr: A pointer to the memory location containing the data to be written.
- size: The size (in bytes) of each element to be written.
- count: The number of elements to be written.
- stream: A pointer to the file object to be written to.
The function returns the number of elements successfully written.
Example code snippet that demonstrates how to use fread and fwrite to read and write binary data to a file:
struct student {
char name[50];
int age;
char gender;
char grade;
};
struct student s = {"John Smith", 18, 'M', 'A'};
// Write binary data to a file using fwrite
FILE *fp;
fp = fopen("student_data.bin", "wb");
fwrite(&s, sizeof(struct student), 1, fp);
fclose(fp);
// Read binary data from a file using fread
fp = fopen("student_data.bin", "rb");
struct student s2;
fread(&s2, sizeof(struct student), 1, fp);
fclose(fp);
// Print the data read from the file
printf("Name: %s\n", s2.name);
printf("Age: %d\n", s2.age);
printf("Gender: %c\n", s2.gender);
printf("Grade: %c\n", s2.grade);
In this example, we use fwrite to write the student data to a binary file named "student_data.bin".
We then use fread to read the binary data from the same file, and store it in a new struct student object named s2.
Finally, we print the values of the s2
object to verify that the data was read correctly from the file.
Note: There are two types of Text file
- ASCI test file
- Binary test file
Conclusion
The fread()
and fwrite()
functions are powerful tools for reading and writing data from and to binary files in C. They are more efficient than the fgets()
and fputs()
functions, and they can be used to read and write any type of data, including integers, floats, strings, and structures.
Further Reading:
fseek and ftell in c
fread() and fwrite() in C
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!