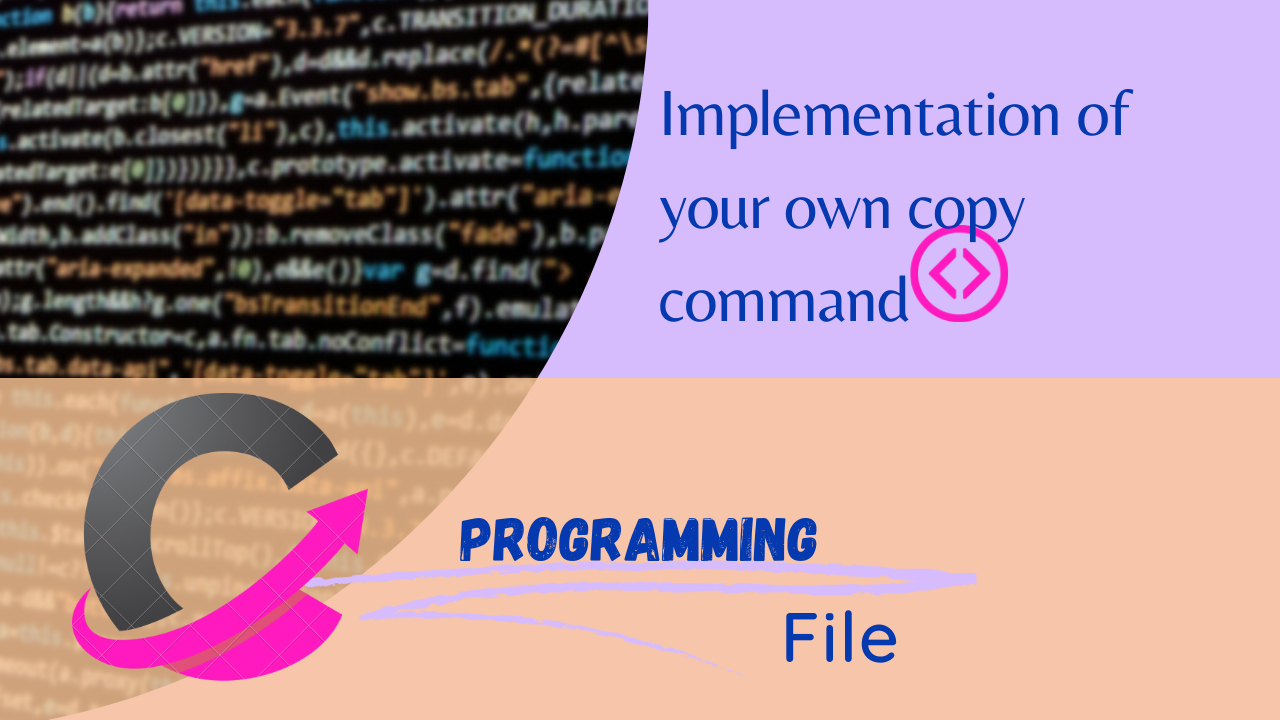
Implementation of your own copy command in c
C Programming: Building Your Own Copy Command
To implement your own copy command in C, you can use the following steps:
- Declare two file pointers, one for the source file and one for the destination file.
- Open the source file for reading and the destination file for writing.
- Create a buffer to store the data to be copied.
- Read a block of data from the source file into the buffer.
- Write the block of data from the buffer to the destination file.
- Repeat steps 4 and 5 until the end of the source file is reached.
- Close the source file and the destination file.
C code shows how to implement the above steps:
C programming
#include <stdio.h>
void copy_file(const char *source_filename, const char *destination_filename) {
FILE *source_file, *destination_file;
char buffer[1024];
size_t bytes_read, bytes_written;
// Open the source file for reading.
source_file = fopen(source_filename, "r");
if (source_file == NULL) {
printf("Error opening source file: %s\n", source_filename);
return;
}
// Open the destination file for writing.
destination_file = fopen(destination_filename, "w");
if (destination_file == NULL) {
printf("Error opening destination file: %s\n", destination_filename);
fclose(source_file);
return;
}
// Read a block of data from the source file into the buffer.
bytes_read = fread(buffer, 1, sizeof(buffer), source_file);
while (bytes_read > 0) {
// Write the block of data from the buffer to the destination file.
bytes_written = fwrite(buffer, 1, bytes_read, destination_file);
if (bytes_written != bytes_read) {
printf("Error writing to destination file: %s\n", destination_filename);
fclose(source_file);
fclose(destination_file);
return;
}
// Read another block of data from the source file into the buffer.
bytes_read = fread(buffer, 1, sizeof(buffer), source_file);
}
// Close the source file.
fclose(source_file);
// Close the destination file.
fclose(destination_file);
}
int main() {
copy_file("source.txt", "destination.txt");
return 0;
}
To compile and run this code, you can use the following commands:
gcc -o copy copy.c
./copy
This will copy the contents of the file source.txt
to the file destination.txt.
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!