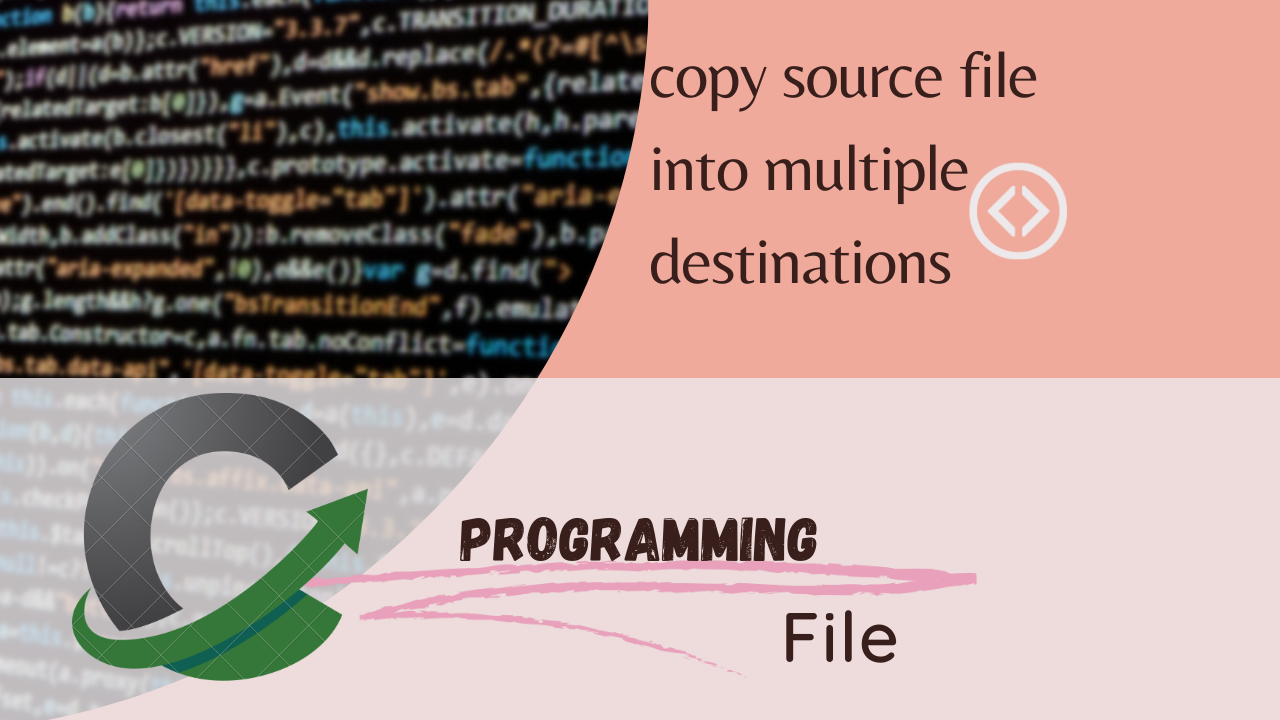
copy source file into multiple destination of string in c
Copy Source File into Multiple Destinations in C - Step-by-Step Guide
"Copy source file into multiple destination of string in C" means writing a C program that can take a source file and an array of destination file names as input, and then copy the contents of the source file into each of the destination files.
In other words, this task involves reading the contents of a source file and writing it to multiple destination files simultaneously. The number of destination files can vary, and the names of the destination files are provided as an array of strings.
By implementing this functionality in C, you can create a flexible and customizable file copying tool that can be used to copy a single file to multiple destinations with different names and formats. This can be useful in scenarios where you must create backups of important files or distribute files to multiple recipients.
Example 01: Write a c program to copy the source file into multiple destinations of the string
#include<stdio.h>
void main(int argc,char **argv)
{
if(argc<3)
{
printf("usage:./my_cp sf d1 d2 d3\n");
return;
}
File *fs,*fd;
char ch;
int i;
fs=fopen(argv[1],"r");
if(fs==0)
{
printf("File not present..\n");
return;
}
for(i=2;i<argc;i++)
{
fd=fopen(argv[i],"w");
while((ch=fgetc(fs))!=-1)
fputc(ch,fd);
rewind(fs);
}
}
Example 02: write a c program to copy the source file into multiple destinations of the string
To copy a source file into multiple destination files in C, you can use the fopen, fread, fwrite, and fclose functions from the standard I/O library.
Here's an example implementation that takes a source file name and an array of destination file names as input and copies the contents of the source file to each of the destination files:
#include <stdio.h>
#include <stdlib.h>
int main(int argc, char *argv[]) {
if (argc < 3) {
printf("Usage: %s [ ...]\n", argv[0]);
return 1;
}
// open the source file for reading in binary mode
FILE *src = fopen(argv[1], "rb");
if (!src) {
printf("Error: could not open source file '%s'\n", argv[1]);
return 1;
}
// open each destination file for writing in binary mode
for (int i = 2; i < argc; i++) {
FILE *dst = fopen(argv[i], "wb");
if (!dst) {
printf("Error: could not open destination file '%s'\n", argv[i]);
fclose(src);
return 1;
}
// read the contents of the source file and write it to the destination file
char buffer[1024];
size_t n;
while ((n = fread(buffer, 1, sizeof(buffer), src))) {
if (fwrite(buffer, 1, n, dst) != n) {
printf("Error: could not write to destination file '%s'\n", argv[i]);
fclose(src);
fclose(dst);
return 1;
}
}
fclose(dst);
}
fclose(src);
printf("Successfully copied '%s' to %d destination files\n", argv[1], argc-2);
return 0;
}
To use this program, simply compile the code into an executable and run it with the source file name and the array of destination file names as arguments:
gcc -o copy copy.c
./copy input.txt output1.txt output2.txt output3.txt
This will copy the contents of input.txt to output1.txt, output2.txt, and output3.txt.