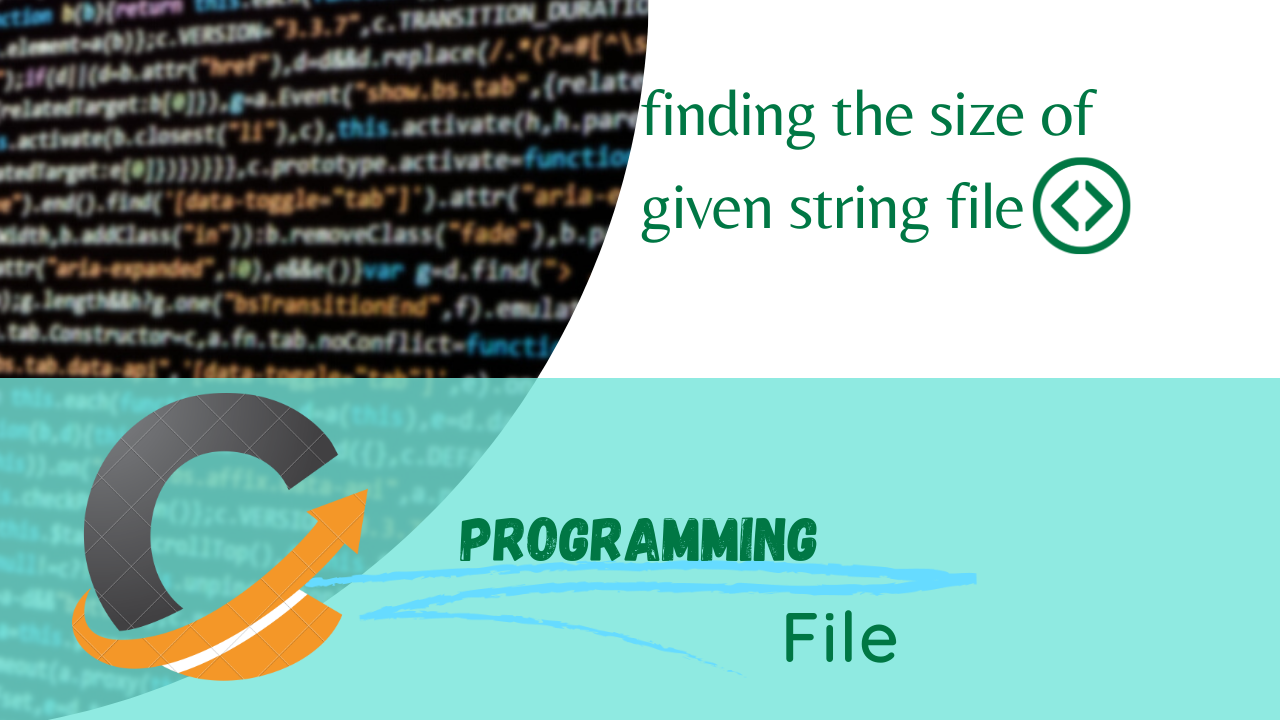
C program for finding the size of given string size using file with electro4u
c program for finding the size of a given string size using file
A C program for finding the size of a given string size using a file is a program that reads a string from a file and calculates its size in bytes. The size of a string in bytes is the number of characters in the string, plus one for the null terminator character.
#include
void main(int argc,char **argv)
{
if(argc!=2)
{
printf("usage:./a.out fname\n");
return;
}
FILE *fp;
fp=fopen(argv[1],"r");
if(fp==0)
{
printf("File not present...\n");
return;
}
int c=0;
char ch;
while((ch=fgetc(fp))!=-1)
c++;
printf("c=%d\n",c);
}
The output of the program will be the number of characters in the file, followed by a newline character.
For example, if the file myfile.txt contains the following text:
This is a test file.
Then the output of the program will be:
19
c program for finding the size of a given string size using file
To find the size of a given string using a file in C, you can use the fseek() and ftell() functions from the standard I/O library. Here's an example program that demonstrates how to use these functions to find the size of a file:
#include
int main() {
char filename[] = "example.txt"; // example file name
FILE *file = fopen(filename, "r"); // open file for reading
if (!file) {
printf("Error: could not open file '%s'\n", filename);
return 1;
}
fseek(file, 0L, SEEK_END); // move file pointer to end of file
long size = ftell(file); // get current position of file pointer (which is the file size)
fseek(file, 0L, SEEK_SET); // move file pointer back to beginning of file
fclose(file); // close the file
printf("The size of file '%s' is %ld bytes\n", filename, size);
return 0;
}
In this program, we define a file name filename and open the file for reading using fopen(). We then use fseek() to move the file pointer to the end of the file and ftell() to get the current position of the file pointer, which is the size of the file. We store the file size in a long variable size and then move the file pointer back to the beginning of the file using fseek().
Finally, we close the file using fclose() and print the size of the file to the console using printf().
When we compile and run this program, it will output something like:
The size of file 'example.txt' is 123 bytes
which indicates the size of the file in bytes.
Top Resources
- Learn-C.org
- C Programming at LearnCpp.com
- GeeksforGeeks - C Programming Language
- C Programming on Tutorialspoint
- Codecademy - Learn C
- CProgramming.com
- C Programming Wikibook
- C Programming - Reddit Community
- C Programming Language - Official Documentation
- GitHub - Awesome C
- HackerRank - C Language
- LeetCode - C