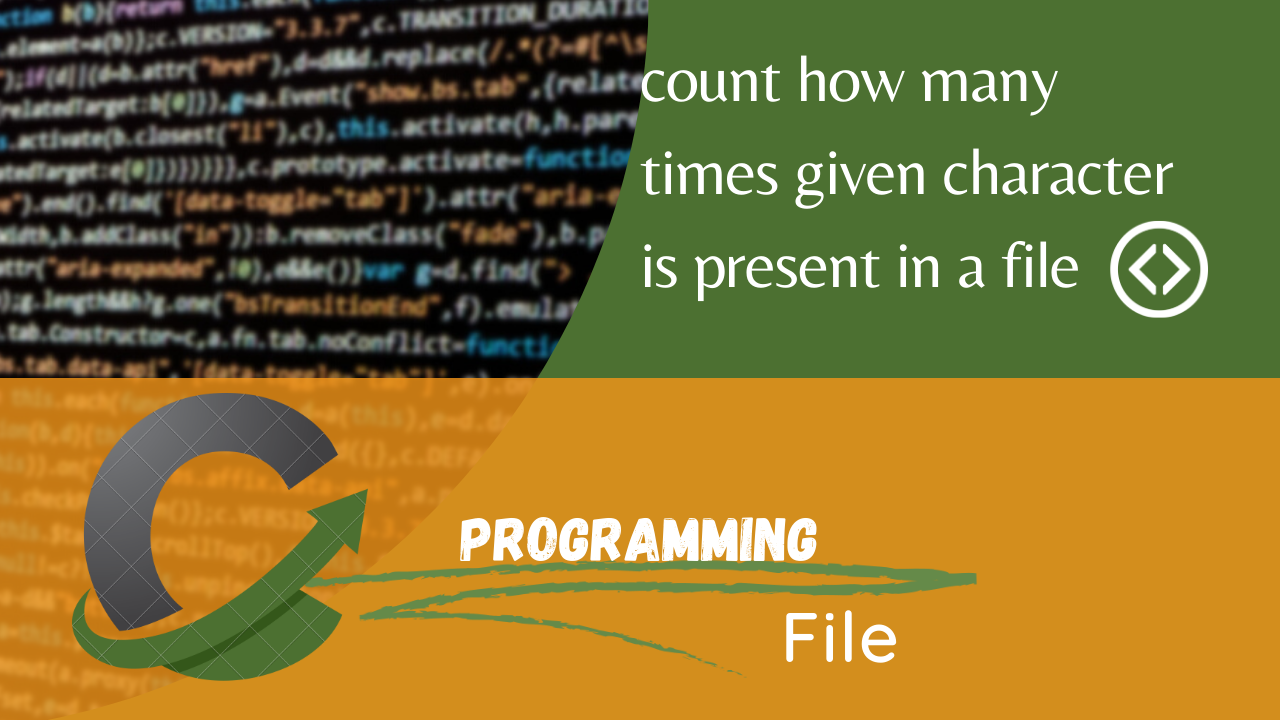
write a c program to count how many times given character is present in a file
Character Frequency Counter in C - File Handling
"Write a C program to count how many times a given character is present in a file" means that you need to create a program in the C programming language that reads a file and counts the number of times a specific character appears in the file. The program should prompt the user to input the name of the file and the character to be counted. Then, the program should open the file, read it character by character, and count how many times the given character appears. Finally, the program should print out the total count of the given characters in the file.
Example: write a c program to count how many times a given character is present in a file
#include<stdio.h>
void main(int argc, char **argv)
{
if(argc!=3)
{
printf("usage:./a.out fname char\n");
return;
}
FILE *fp;
fp=fopen(argv[1],"r");
if(fp==0)
{
printf("file is not present...\n");
return;
}
int c=0;
char ch;
while((ch=fgetc(fp))!=-1)
{
if(argv[2][0]==ch)
c++;
}
printf("c=%d\n",c);
}
The output of the program will be the number of occurrences of the character in the file, followed by a newline character.
For example, if the file myfile.txt contains the following text:
This is a test file.
And the user specifies the character a, then the output of the program will be:
3
Example 02: c program to count how many times a given character is present in a file
C program that counts the number of times a given character is present in a file:
#include<stdio.h>
int main() {
FILE *fp;
char filename[100], ch;
int count = 0;
printf("Enter the filename: ");
scanf("%s", filename);
printf("Enter the character to be counted: ");
scanf(" %c", &ch);
fp = fopen(filename, "r");
if (fp == NULL) {
printf("Could not open file %s", filename);
return 1;
}
while ((ch = fgetc(fp)) != EOF) {
if (ch == getchar()) {
count++;
}
}
printf("The character '%c' occurs %d times in the file.", ch, count);
fclose(fp);
return 0;
}
For example, if the user enters the filename myfile.txt and the character a, then the program will print the following output:
The character 'a' occurs 3 times in the file.
The program first prompts the user to enter the filename and the character to be counted. It then attempts to open the file for reading using the open function. If the file cannot be opened, the program will print an error message and exit with a non-zero status code. The program then reads the file character by character using the fgetc function. If the character matches the given character, the program increments the count. Finally, the program prints the number of times the character occurs in the file and closes the file using the fclose function.
Note that this program assumes that the file is a plain text file. If the file is binary, some characters may not be printable and the output may be unexpected.
Top Resources
- Learn-C.org
- C Programming at LearnCpp.com
- GeeksforGeeks - C Programming Language
- C Programming on Tutorialspoint
- Codecademy - Learn
- CProgramming.com
- C Programming Wikibook
- C Programming - Reddit Community
- C Programming Language - Official Documentation
- GitHub - Awesome C
- HackerRank - C Language
- LeetCode - C