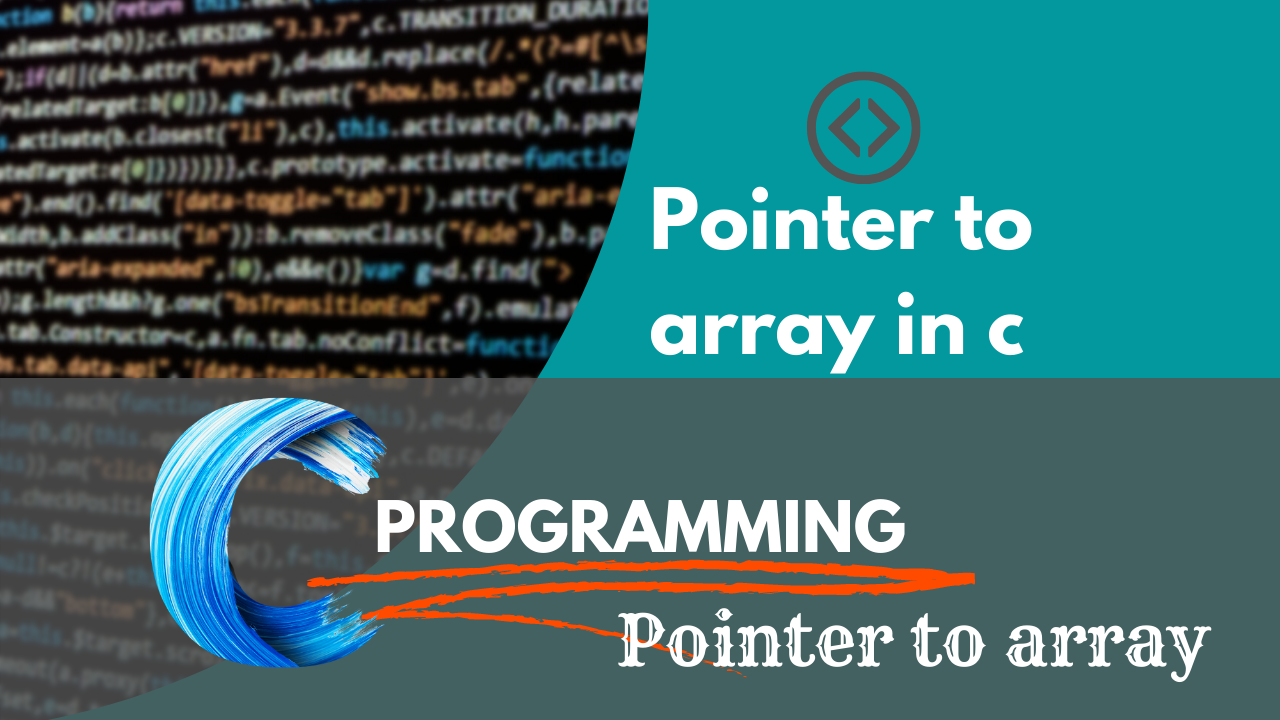
Pointer to array in c
Pointer to array in C
A pointer to an array is a variable that stores the address of the first element of the array. This means that you can use a pointer to access and modify the elements of the array without having to use the array name.
syntax:
For example, to declare a pointer to an array of integers, you would use the following declaration:
int *array_ptr;
Once you have declared a pointer to an array, you can initialize it by assigning it the address of the array. You can do this using the & operator. For example, the following code initializes the pointer array_ptr to point to the array array:
int array[10];
int *array_ptr = &array;
Once a pointer to an array has been initialized, you can use it to access and modify the elements of the array. To do this, you simply dereference the pointer using the * operator. For example, the following code prints the value of the first element of the array array to the console:
printf("%d", *array_ptr);
You can also use a pointer to an array to iterate over the elements of the array. For example, the following code prints the values of all elements of the array array to the console:
for (int i = 0; i < 10; i++) {
printf("%d ", *(array_ptr + i));
}
Advantages of using pointers to arrays
There are several advantages to using pointers to arrays:
- Pointers can be used to access and modify the elements of an array without having to use the array name. This can make your code more concise and readable.
- Pointers can be used to pass arrays to functions as arguments. This can make your code more modular and reusable.
- Pointers can be used to implement dynamic arrays. Dynamic arrays are arrays whose size can be changed at runtime.
Disadvantages of using pointers to arrays
There are also a few disadvantages to using pointers to arrays:
- Pointers can be dangerous if they are not used correctly. For example, if you accidentally dereference a null pointer, your program will crash.
- Pointers can make your code more difficult to understand. This is because you need to keep track of what each pointer is pointing to.
If you are new to pointers, I recommend that you start by learning about the basics of pointers before using pointers to arrays. Once you have a good understanding of pointers, you can start using them to improve your code.
Further Reading:
What is Pointer to an array? Give one example.
Print The array element using Pointer to an array
Implications of Passing an Array and a Pointer to an Array as Function Arguments in C
Write a C program that uses a pointer to an array to find the sum of all elements.
Assingment of pointer to an array in c
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!