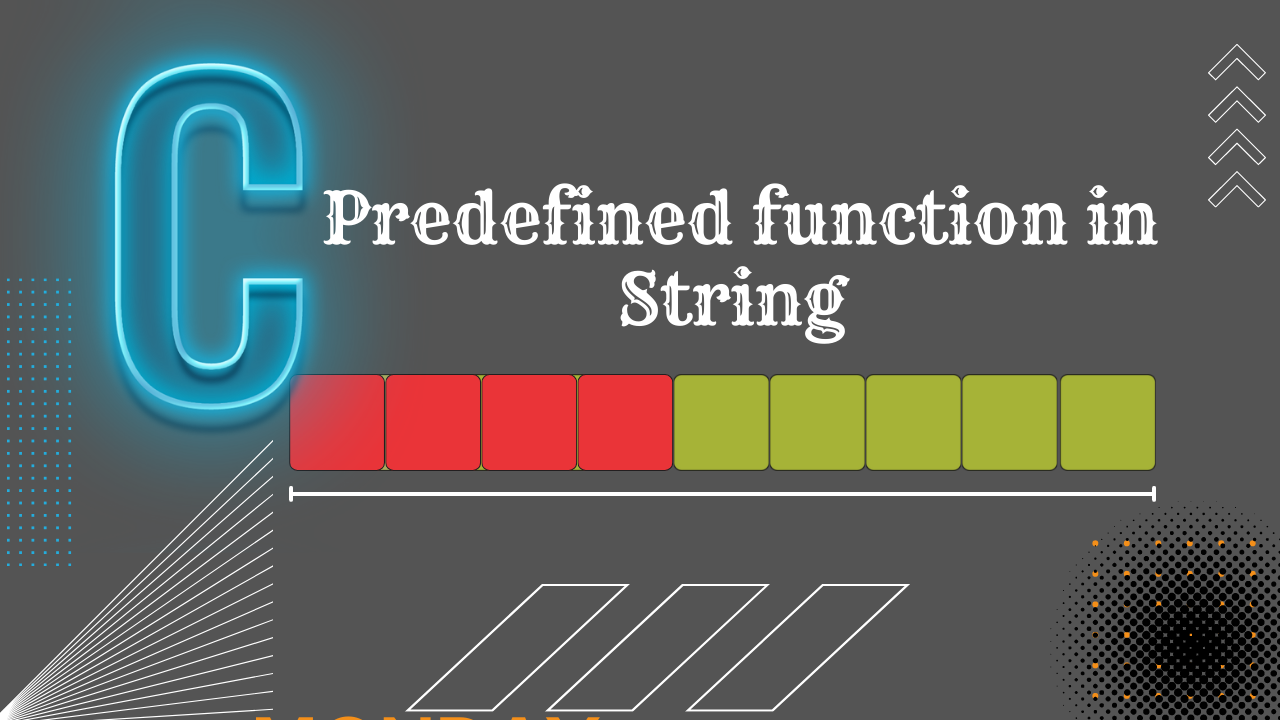
Predefined function in string in c
Predefined functions in string
Introduction
Programming languages provide a number of predefined functions for working with strings. These functions can be used to perform a variety of tasks, such as copying, concatenating, searching, and comparing strings.
In this page, we will discuss some of the most common predefined functions in string.
Strcpy
The strcpy()
function copies one string to another string. The syntax for the strcpy()
function is as follows:
char* strcpy(char* destination, const char* source);
The strcpy()
function takes two arguments:
- destination: The pointer to the destination string.
- source: The pointer to the source string.
The strcpy()
function copies the characters from the source string to the destination string, until it reaches the null terminator character (\0).
Strcat()
The strcat() function concatenates two strings. The syntax for the strcat() function is as follows:
char* strcat(char* destination, const char* source);
The strcat()
function takes two arguments:
- destination: The pointer to the destination string.
- source: The pointer to the source string.
The strcat() function appends the characters from the source string to the end of the destination string.
strlen()
The strlen() function returns the length of a string. The syntax for the strlen() function is as follows:
size_t strlen(const char* string);
The strlen()
function takes one argument:
- string: The pointer to the string.
The strlen()
function returns the number of characters in the string, excluding the null terminator character.
strcmp()
The strcmp()
function compares two strings. The syntax for the strcmp() function is as follows:
int strcmp(const char* string1, const char* string2);
The strcmp()
function takes two arguments:
- string1: The pointer to the first string.
- string2: The pointer to the second string.
The strcmp()
function returns an integer value, which indicates the relationship between the two strings:
- 0: The two strings are equal.
- A positive value: The first string is greater than the second string.
- A negative value: The first string is less than the second string.
Example
The following example shows how to use the strcpy(), strcat(), strlen(), and strcmp() functions:
#include <stdio.h>
#include <string.h>
int main() {
char str1[100], str2[100], str3[200];
printf("Enter the first string: ");
gets(str1);
printf("Enter the second string: ");
gets(str2);
// Copy the first string to the third string
strcpy(str3, str1);
// Concatenate the second string to the third string
strcat(str3, str2);
// Print the length of the third string
printf("The length of the third string is %d.\n", strlen(str3));
// Compare the first and second strings
if (strcmp(str1, str2) == 0) {
printf("The two strings are equal.\n");
} else {
printf("The two strings are not equal.\n");
}
return 0;
}
Output:
Enter the first string: Hello Enter the second string: World The length of the third string is 10. The two strings are not equal.
Conclusion
Predefined functions in string are a powerful tool for working with strings. They can be used to perform a variety of tasks, such as copying, concatenating, searching, and comparing strings.
Further Reading:
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [[email protected]].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them