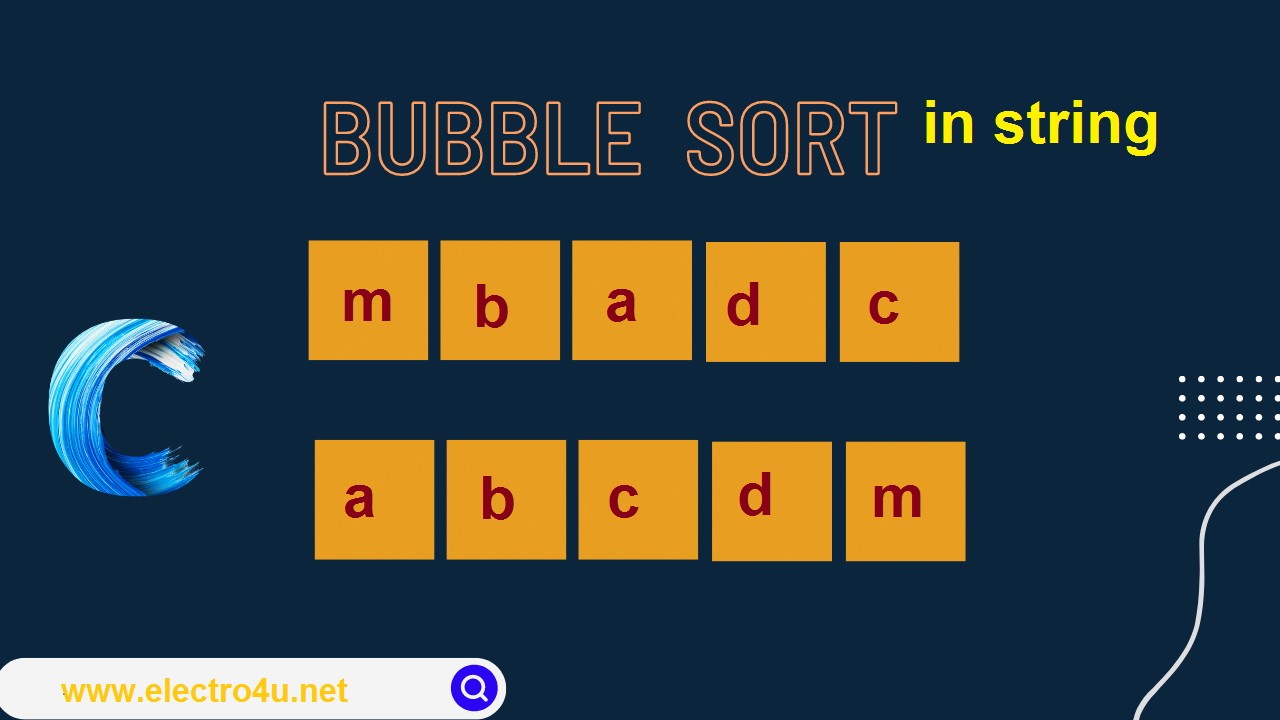
Bubble short in string in c
Bubble Sort for Strings in C Programming
Bubble sort is a simple sorting algorithm that works by repeatedly swapping adjacent elements if they are in the wrong order. It is called bubble sort because smaller elements "bubble"
to the top of the array or string, while larger elements sink to the bottom.
To implement a bubble sort in C for strings, we can use the following algorithm:
- Initialize two pointers,
i
andj
, to the beginning of the array. - Compare the strings at indices
i
andj.
If the string at indexi
is greater than the string at indexj,
swap them. - Increment
j
and repeat steps2
and3
untilj
reaches the end of the array. - Decrement
i
and repeat steps2-4
until i reaches the beginning of the array.
Bubble short in string in c programming
C programming
#include
void main()
{
char s[20],t;
int i,j,l;
printf("Enter the string:\n");
scanf("%s",s);
printf("Before s=%s\n",s);
for(l=0;s[l];l++);
for(i=0;i<l-1;i++)
{
for(j=0;j<l-1-i;j++)
{
if(s[j]>s[j+1])
{
t=s[j];
s[j]=s[j+1];
s[j+1]=t;
}
}
}
printf("After s:%s\n",s);
}
Output:
Enter the string: mbadct
Before s=mbadct
After s:abcdmt
When applied to strings, bubble sort works by comparing pairs of adjacent strings and swapping them if they are in the wrong order. The comparison is usually done using the strcmp
function, which compares two strings lexicographically (i.e., based on their alphabetical order).
C code implements the bubble sort algorithm for strings:
#include <stdio.h>
#include <string.h>
void bubble_sort(char **strings, int n) {
int i, j;
for (i = 0; i < n - 1; i++) {
for (j = 0; j < n - i - 1; j++) {
if (strcmp(strings[j], strings[j + 1]) > 0) {
char *temp = strings[j];
strings[j] = strings[j + 1];
strings[j + 1] = temp;
}
}
}
}
int main() {
char *strings[] = {"Alice", "Bob", "Carol", "Dave", "Eve"};
int n = sizeof(strings) / sizeof(strings[0]);
printf("Unsorted strings:\n");
for (int i = 0; i < n; i++) {
printf("%s\n", strings[i]);
}
bubble_sort(strings, n);
printf("\nSorted strings:\n");
for (int i = 0; i < n; i++) {
printf("%s\n", strings[i]);
}
return 0;
}
Output:
Unsorted strings:
Alice
Bob
Carol
Dave
Eve
Sorted strings:
Alice
Bob
Carol
Dave
Eve
Further Reading:
Bubble shorting in c programming
Bubble short in string in c
Write a c program to short 2D array using bubble short
Selection shorting in c Programming
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!