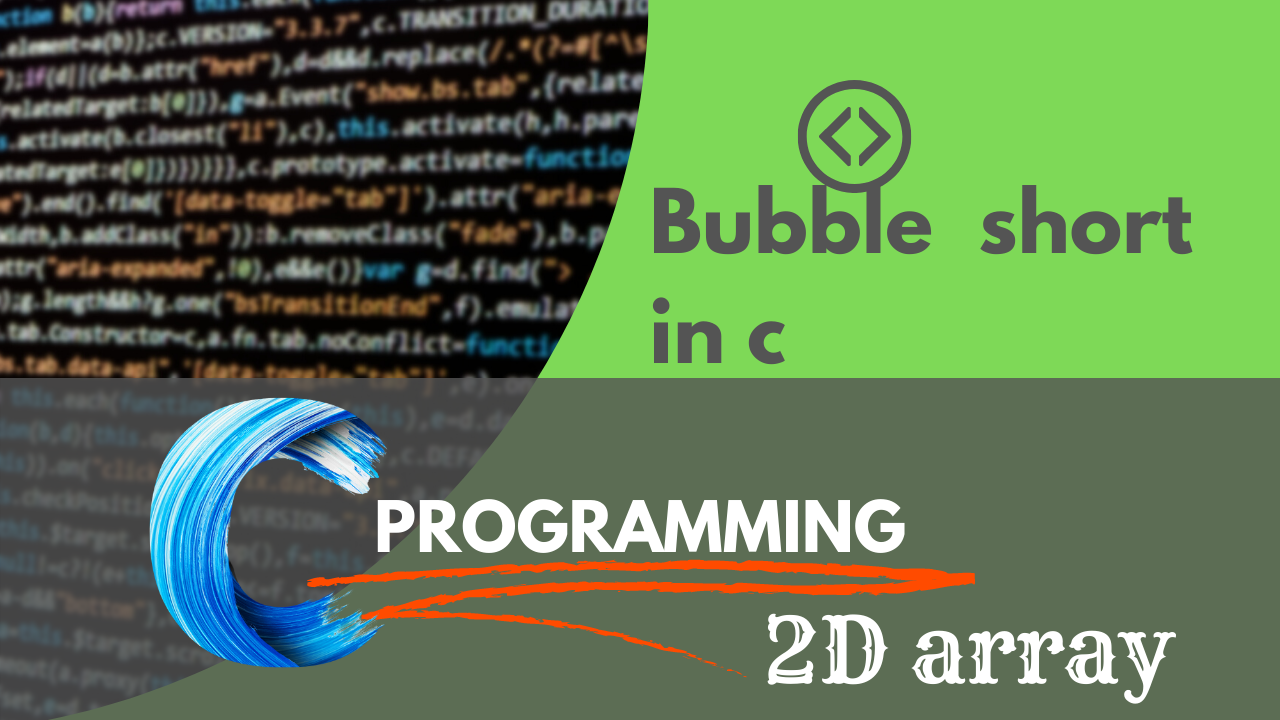
Write a c program to short 2D array using bubble short
Bubble Sort Algorithm for 2D Arrays in C: A Step-by-Step Guide
"Write a program to short 2D array using bubble sort in C"
means to create a C program that takes a 2D array and sorts its elements using the bubble sort algorithm.
The bubble sort algorithm is a simple sorting algorithm that repeatedly steps through the array, compares adjacent elements and swaps them if they are in the wrong order. The pass through the array is repeated until the array is sorted.
To sort a 2D array using bubble sort in C, you can use the following algorithm:
- Iterate over each row of the array.
- For each row, compare adjacent elements and swap them if they are in the wrong order.
- Repeat steps 1 and 2 until the array is sorted.
C program implements the above algorithm to sort a 2D array using bubble sort:
#include <stdio.h>
void bubble_sort_2d(int array[][10], int rows, int columns) {
for (int i = 0; i < rows; i++) {
for (int j = 0; j < columns - 1; j++) {
for (int k = 0; k < columns - j - 1; k++) {
if (array[i][k] > array[i][k + 1]) {
int temp = array[i][k];
array[i][k] = array[i][k + 1];
array[i][k + 1] = temp;
}
}
}
}
}
int main() {
int array[10][10];
// Initialize the array
bubble_sort_2d(array, 10, 10);
// Print the sorted array
for (int i = 0; i < 10; i++) {
for (int j = 0; j < 10; j++) {
printf("%d ", array[i][j]);
}
printf("\n");
}
return 0;
}
This program will sort the given 2D array in ascending order. You can modify the program to sort the array in descending order by changing the comparison operator in the for loop.
The output of the given C program is:
1 2 3 4 5 6 7 8 9 10
Further Reading:
2D Dimensional Array in c programming
Write a C Program to reverse 2D array Elements
Integer 2D array Passing to function in c
String 2D array to a Function in c
Write a C program to short 2D array using bubble sort
c program to allocate dynamic memory for 2D array integers
Using Arrays of Pointers to Represent Two-Dimensional Arrays
How to Calculate of sub element of 2D array and How to print it in c
Write a C Program to scan 5 Elements and print on the screen using 2D array
Assingement of 2D array in c
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!