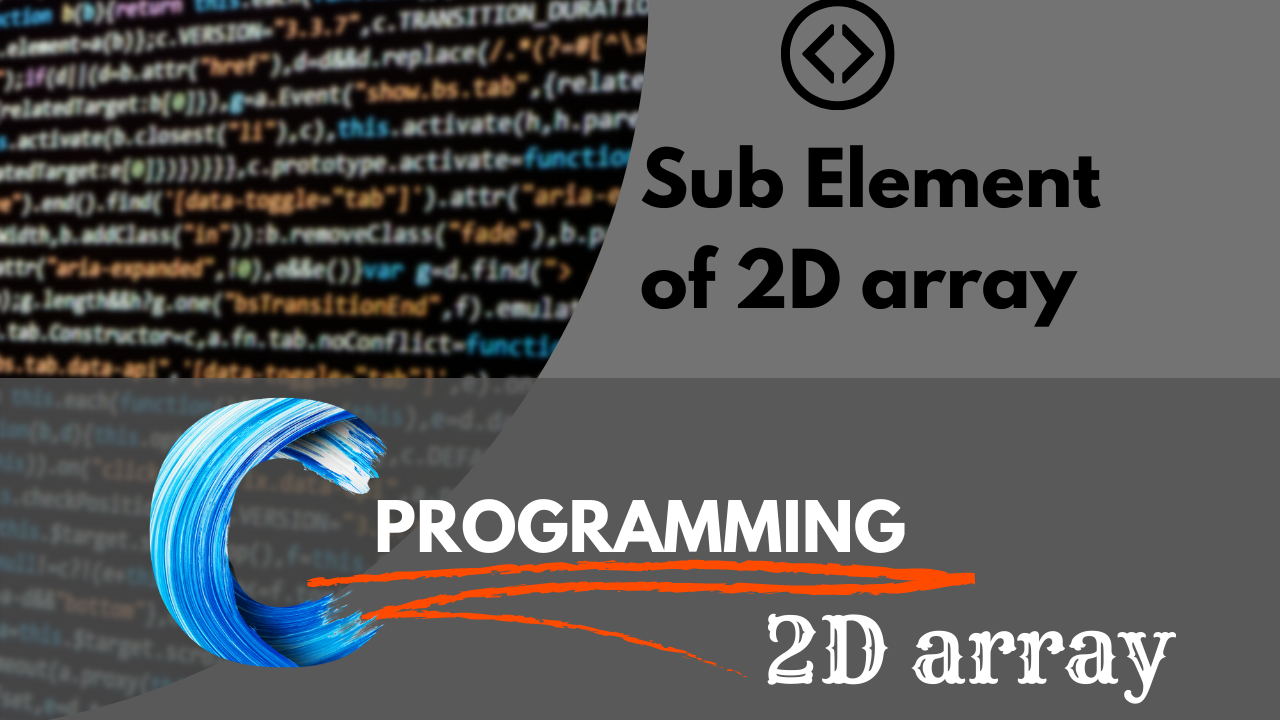
How to Calculate of sub element of 2D array and How to print it in c
Calculating Sub-Elements in a 2D Array and Printing Results in C
To calculate the sum of sub-elements of a 2D array in C programming, you can use nested loops to iterate over the rows and columns of the sub-array and add up the elements. Here's an example of how to calculate the sum of a 2x2 sub-array starting from the top-left corner of a 4x4 array:
To calculate the sub-element of a 2D array in C, you can use the following steps:
- Declare a variable to store the sub-element.
- Use the following formula to calculate the sub-element:
sub_element = array[row][column];
array
is the 2D array.row
is the row index of the sub-element.column
is the column index of the sub-element.
Print the sub-element to the console using the following statement:
printf("%d", sub_element);
For example, the following code shows how to calculate and print the sub-element at row 2, column 3 of a 2D array:
#include <stdio.h>
int main() {
int array[3][3] = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
// Calculate the sub-element at row 2, column 3
int sub_element = array[2][3];
// Print the sub-element to the console
printf("%d", sub_element);
return 0;
}
Output:
9
You can also use a nested loop to iterate over the 2D array and calculate and print the sub-element of each element. For example, the following code shows how to print all the sub-elements of a 2D array:
#include <stdio.h>
int main() {
int array[3][3] = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
// Iterate over the 2D array and print the sub-element of each element
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
printf("%d ", array[i][j]);
}
printf("\n");
}
return 0;
}
Output:
1 2 3
4 5 6
7 8 9
Further Reading:
2D Dimensional Array in c programming
Write a C Program to reverse 2D array Elements
Integer 2D array Passing to function in c
String 2D array to a Function in c
Write a C program to short 2D array using bubble sort
c program to allocate dynamic memory for 2D array integers
Using Arrays of Pointers to Represent Two-Dimensional Arrays
How to Calculate of sub element of 2D array and How to print it in c
Write a C Program to scan 5 Elements and print on the screen using 2D array
Assingement of 2D array in c
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!