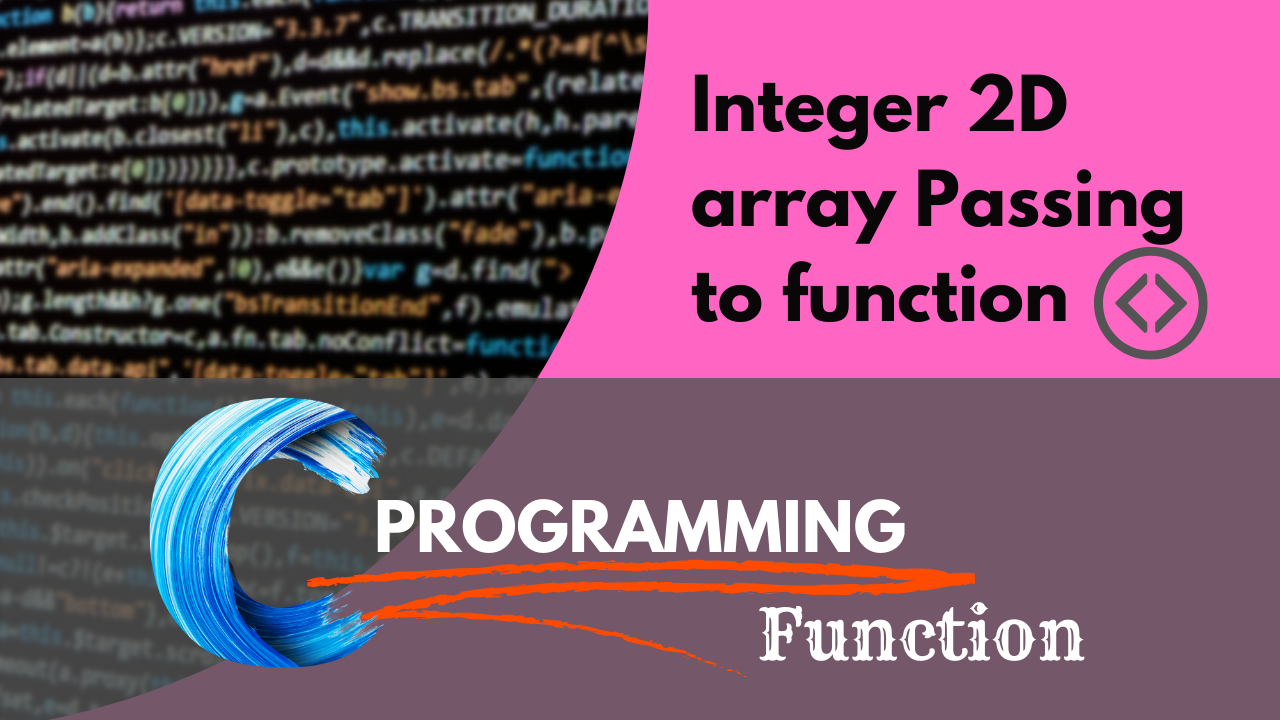
Integer 2D array Passing to function in c
what does mean Integer 2D array Passing to function
Passing an Integer 2D array to a function means that you are sending a 2-dimensional array of integers as an argument to a function in your code. A 2D array is an array of arrays where each element in the array is an array itself.
When passing a 2D array to a function, you are essentially passing a pointer to the first element of the array, which is also an array. The pointer points to the memory address of the first element of the first array.
To pass an integer 2D array to a function in C, you can use one of the following methods:
Pass the entire array as an argument.
This is the simplest method, but it can be inefficient if the array is large. To do this, you declare the function parameter to be a 2D array of the same dimensions as the array you are passing. For example:
void print_array(int array[10][10]) {
for (int i = 0; i < 10; i++) {
for (int j = 0; j < 10; j++) {
printf("%d ", array[i][j]);
}
printf("\n");
}
}
To call the function, you simply pass the 2D array as an argument. For example:
int main() {
int array[10][10];
// Initialize the array
print_array(array);
return 0;
}
Pass an array of pointers to the function.
This method is more efficient than the first method, but it is also more complex. To do this, you declare the function parameter to be an array of pointers to integers. The number of pointers in the array should be equal to the number of rows in the 2D array. For example:
void print_array(int *array[10]) {
for (int i = 0; i < 10; i++) {
for (int j = 0; j < 10; j++) {
printf("%d ", array[i][j]);
}
printf("\n");
}
}
To call the function, you pass an array of pointers to the 2D array as an argument. To do this, you first need to get the address of each row of the 2D array. You can do this using the & operator. For example:
int main() {
int array[10][10];
// Initialize the array
// Get the address of each row of the array
int *array_ptrs[10];
for (int i = 0; i < 10; i++) {
array_ptrs[i] = array[i];
}
print_array(array_ptrs);
return 0;
}
Pass a pointer to the 2D array as an argument.
This method is the most efficient, but it is also the most complex. To do this, you declare the function parameter to be a pointer to a pointer to an integer. For example:
void print_array(int **array) {
for (int i = 0; i < 10; i++) {
for (int j = 0; j < 10; j++) {
printf("%d ", array[i][j]);
}
printf("\n");
}
}
To call the function, you simply pass the address of the 2D array as an argument. For example:
int main() {
int array[10][10];
// Initialize the array
print_array(&array);
return 0;
}
Which method you choose depends on your specific needs. If you need to pass a large array to the function, you should use the second or third method. If you need to pass the array to multiple functions, you should also use the second or third method, as this will make your code more reusable.
How to pass an integer 2D array to a function using the second method:
#include <stdio.h>
void print_array(int *array[10]) {
for (int i = 0; i < 10; i++) {
for (int j = 0; j < 10; j++) {
printf("%d ", array[i][j]);
}
printf("\n");
}
}
int main() {
int array[10][10];
// Initialize the array
// Get the address of each row of the array
int *array_ptrs[10];
for (int i = 0; i < 10; i++) {
array_ptrs[i] = array[i];
}
print_array(array_ptrs);
return 0;
}
To compile and run this program, you can use the following commands:
gcc -o program program.c
./program
This will print the following output to the console:
0 1 2 3 4 5 6 7 8 9
10 11 12 13 14 15 16 17 18 19
...
90 91 92 93 94 95 96 97 98 99
Further Reading:
2D Dimensional Array in c programming
Write a C Program to reverse 2D array Elements
Integer 2D array Passing to function in c
String 2D array to a Function in c
Write a C program to short 2D array using bubble sort
c program to allocate dynamic memory for 2D array integers
Using Arrays of Pointers to Represent Two-Dimensional Arrays
How to Calculate of sub element of 2D array and How to print it in c
Write a C Program to scan 5 Elements and print on the screen using 2D array
Assingement of 2D array in c
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [[email protected]].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them