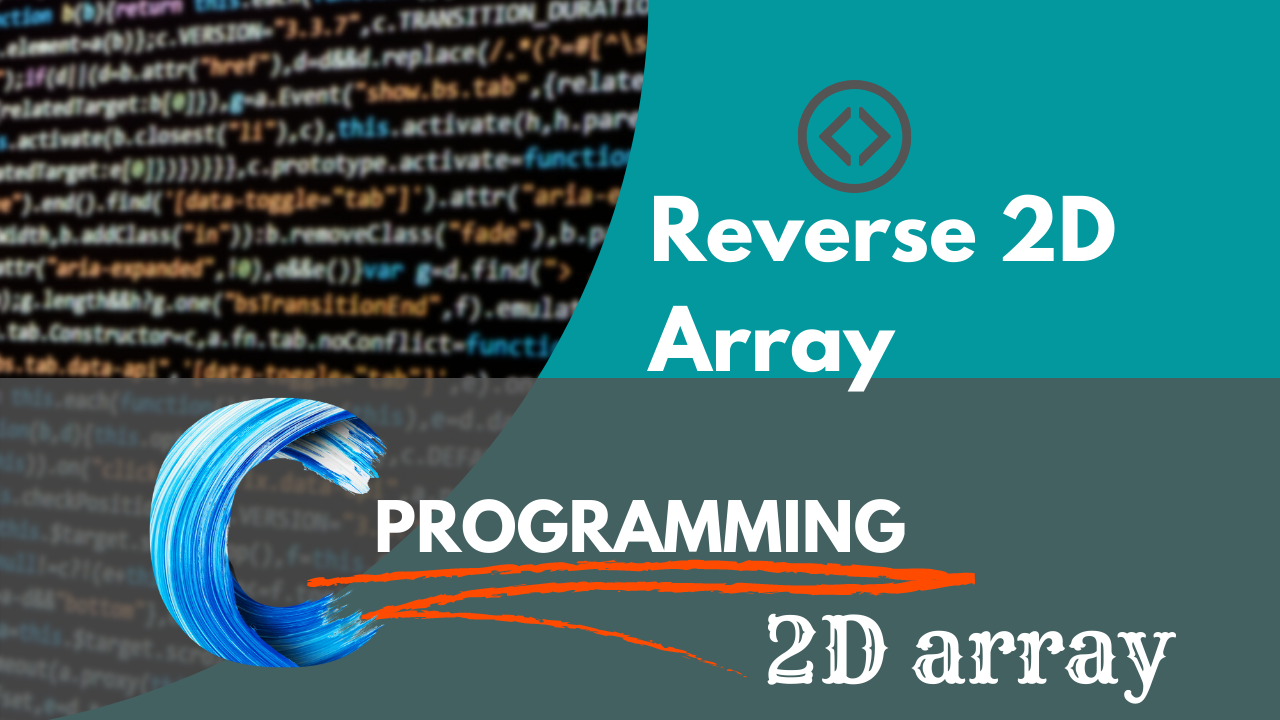
Write a program to reverse 2D array Elements in c
C Program to Reverse Elements in a 2D Array
To write a C program to reverse 2D array elements, we can use the following steps:
- Declare a function to reverse a 2D array. This function should take the 2D array as a parameter and return a pointer to the reversed array.
- In the reverse function, iterate over the rows of the 2D array in reverse order. For each row, swap the elements of the row using two temporary variables.
- Return the pointer to the reversed array.
- In the main function, declare a 2D array and initialize it with some values.
- Call the reverse function to reverse the 2D array.
- Print the contents of the reversed array.
C program to reverse 2D array elements:
#include <stdio.h>
void reverse_array(int array[][10], int rows, int columns) {
for (int i = 0; i < rows / 2; i++) {
for (int j = 0; j < columns; j++) {
int temp = array[i][j];
array[i][j] = array[rows - i - 1][j];
array[rows - i - 1][j] = temp;
}
}
}
void print_array(int array[][10], int rows, int columns) {
for (int i = 0; i < rows; i++) {
for (int j = 0; j < columns; j++) {
printf("%d ", array[i][j]);
}
printf("\n");
}
}
int main() {
int array[10][10];
int rows, columns;
printf("Enter the number of rows: ");
scanf("%d", &rows);
printf("Enter the number of columns: ");
scanf("%d", &columns);
for (int i = 0; i < rows; i++) {
for (int j = 0; j < columns; j++) {
printf("Enter element at row %d, column %d: ", i, j);
scanf("%d", &array[i][j]);
}
}
printf("Original array:\n");
print_array(array, rows, columns);
reverse_array(array, rows, columns);
printf("Reversed array:\n");
print_array(array, rows, columns);
return 0;
}
Example output:
Enter the number of rows: 3
Enter the number of columns: 3
Enter element at row 0, column 0: 1
Enter element at row 0, column 1: 2
Enter element at row 0, column 2: 3
Enter element at row 1, column 0: 4
Enter element at row 1, column 1: 5
Enter element at row 1, column 2: 6
Enter element at row 2, column 0: 7
Enter element at row 2, column 1: 8
Enter element at row 2, column 2: 9
Original array:
1 2 3
4 5 6
7 8 9
Reversed array:
7 8 9
4 5 6
1 2 3
Further Reading:
2D Dimensional Array in c programming
Write a C Program to reverse 2D array Elements
Integer 2D array Passing to function in c
String 2D array to a Function in c
Write a C program to short 2D array using bubble sort
c program to allocate dynamic memory for 2D array integers
Using Arrays of Pointers to Represent Two-Dimensional Arrays
How to Calculate of sub element of 2D array and How to print it in c
Write a C Program to scan 5 Elements and print on the screen using 2D array
Assingement of 2D array in c
Enroll Now:
[ C-Programming From Scratch to Advanced 2023-2024] "Start Supercharging Your Productivity!"
Contact Us:
- For any inquiries, please email us at [info@electro4u.net].
- Follow us on insta [ electro4u_offical_ ] for updates and tips.
Note: If you encounter any issues or specific errors when running this program, please let me know and I'll be happy to help debug them!